KHTML
#include <editor.h>
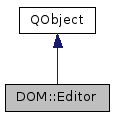
Public Types | |
enum | TriState { FalseTriState, TrueTriState, MixedTriState } |
Detailed Description
This class resembles the editing API when the associated khtml document is editable (in design mode), or contains editable elements.
FIXME: document this thoroughly
API to Wysiwyg Markup-Editor.
Member Enumeration Documentation
Member Function Documentation
void Editor::appliedEditing | ( | khtml::EditCommandImpl * | cmd | ) |
Called when editing has been applied.
Definition at line 404 of file editor.cpp.
void Editor::applyStyle | ( | DOM::CSSStyleDeclarationImpl * | ) |
applies the given style to the current selection
Definition at line 230 of file editor.cpp.
bool Editor::canPaste | ( | ) | const |
returns whether clipboard contains data to be pasted
Definition at line 198 of file editor.cpp.
bool Editor::canRedo | ( | ) | const |
returns whether any actions can be redone
Definition at line 220 of file editor.cpp.
bool Editor::canUndo | ( | ) | const |
returns whether any actions can be undone
Definition at line 225 of file editor.cpp.
void Editor::clearTypingStyle | ( | ) |
Clears the typing style for the document.
Definition at line 479 of file editor.cpp.
void Editor::closeTyping | ( | ) |
Definition at line 484 of file editor.cpp.
void Editor::copy | ( | ) |
copy selection to clipboard
Definition at line 175 of file editor.cpp.
void Editor::cut | ( | ) |
cut selection and insert into clipboard
Definition at line 180 of file editor.cpp.
Executes the given editor command.
- Parameters
-
command name of command userInterface whether a user interface should be used to input data. This is command dependent. value value for command. Its semantic depends on the command.
Definition at line 103 of file editor.cpp.
bool Editor::execCommand | ( | EditorCommand | command, |
bool | userInterface, | ||
const DOMString & | value | ||
) |
Executes the given built-in editor command.
- Parameters
-
EditorCommand index of command userInterface whether a user interface should be used to input data. This is command dependent. value value for command. Its semantic depends on the command.
Definition at line 139 of file editor.cpp.
void Editor::indent | ( | ) |
indent/outdent current selection
Definition at line 491 of file editor.cpp.
PassRefPtr< EditCommandImpl > Editor::lastEditCommand | ( | ) | const |
Returns the most recent edit command applied.
Definition at line 399 of file editor.cpp.
void Editor::outdent | ( | ) |
Definition at line 498 of file editor.cpp.
void Editor::paste | ( | ) |
paste into current selection from clipboard
Definition at line 186 of file editor.cpp.
void Editor::print | ( | ) |
prints the current document
Definition at line 193 of file editor.cpp.
Checks whether the given command is enabled.
Definition at line 109 of file editor.cpp.
bool Editor::queryCommandEnabled | ( | EditorCommand | command | ) |
Checks whether the given command is enabled.
Definition at line 145 of file editor.cpp.
Checks whether the given command's style is indeterminate.
Definition at line 115 of file editor.cpp.
bool Editor::queryCommandIndeterm | ( | EditorCommand | command | ) |
Checks whether the given command's style is indeterminate.
Definition at line 151 of file editor.cpp.
Checks whether the given command's style is state.
Definition at line 121 of file editor.cpp.
bool Editor::queryCommandState | ( | EditorCommand | command | ) |
Checks whether the given command's style is state.
Definition at line 157 of file editor.cpp.
Checks whether the given command is supported in the current context.
Definition at line 127 of file editor.cpp.
bool Editor::queryCommandSupported | ( | EditorCommand | command | ) |
Checks whether the given command is supported in the current context.
Definition at line 163 of file editor.cpp.
Returns the given command's value.
Definition at line 133 of file editor.cpp.
DOMString Editor::queryCommandValue | ( | EditorCommand | command | ) |
Returns the given command's value.
Definition at line 169 of file editor.cpp.
void Editor::reappliedEditing | ( | khtml::EditCommandImpl * | cmd | ) |
Called when editing has been reapplied.
Definition at line 447 of file editor.cpp.
void Editor::redo | ( | ) |
redo last undone action
Definition at line 204 of file editor.cpp.
CSSStyleDeclarationImpl * Editor::selectionComputedStyle | ( | DOM::NodeImpl *& | nodeToRemove | ) | const |
computed style of current selection
Definition at line 361 of file editor.cpp.
Editor::TriState Editor::selectionHasStyle | ( | DOM::CSSStyleDeclarationImpl * | ) | const |
returns whether the selection has got applied the given style
Definition at line 271 of file editor.cpp.
bool Editor::selectionStartHasStyle | ( | DOM::CSSStyleDeclarationImpl * | ) | const |
returns whether the selection has got applied the given style
Definition at line 308 of file editor.cpp.
DOMString Editor::selectionStartStylePropertyValue | ( | int | stylePropertyID | ) | const |
?
Definition at line 341 of file editor.cpp.
void Editor::setTypingStyle | ( | DOM::CSSStyleDeclarationImpl * | ) |
Sets the typing style for the document.
Definition at line 469 of file editor.cpp.
CSSStyleDeclarationImpl * Editor::typingStyle | ( | ) | const |
Returns the typing style for the document.
Definition at line 464 of file editor.cpp.
void Editor::unappliedEditing | ( | khtml::EditCommandImpl * | cmd | ) |
Called when editing has been unapplied.
Definition at line 429 of file editor.cpp.
void Editor::undo | ( | ) |
undo last action
Definition at line 212 of file editor.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.