KHTML
#include <khtmlview.h>
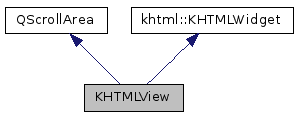
Public Types | |
enum | SmoothScrollingMode { SSMDisabled = 0, SSMWhenEfficient, SSMEnabled } |
Public Slots | |
void | layout () |
virtual void | resizeContents (int w, int h) |
Signals | |
void | cleared () |
void | findAheadActive (bool) |
void | finishedLayout () |
void | hideAccessKeys () |
void | repaintAccessKeys () |
void | zoomView (int) |
Public Member Functions | |
KHTMLView (KHTMLPart *part, QWidget *parent) | |
virtual | ~KHTMLView () |
void | addChild (QWidget *child, int dx, int dy) |
int | contentsHeight () const |
QPoint | contentsToViewport (const QPoint &p) const |
void | contentsToViewport (int x, int y, int &cx, int &cy) const |
int | contentsWidth () const |
int | contentsX () const |
int | contentsY () const |
void | displayAccessKeys () |
int | frameWidth () const |
int | marginHeight () |
int | marginWidth () const |
KHTMLPart * | part () const |
void | print (bool quick=false) |
void | repaintContents (const QRect &r) |
void | repaintContents (int x, int y, int w, int h) |
void | scrollBy (int x, int y) |
void | setContentsPos (int x, int y) |
virtual void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy policy) |
void | setMarginHeight (int y) |
void | setMarginWidth (int x) |
void | setSmoothScrollingMode (SmoothScrollingMode m) |
virtual void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy policy) |
void | setZoomLevel (int percent) |
SmoothScrollingMode | smoothScrollingMode () const |
void | updateContents (const QRect &r) |
void | updateContents (int x, int y, int w, int h) |
QPoint | viewportToContents (const QPoint &p) const |
void | viewportToContents (int x, int y, int &cx, int &cy) const |
int | visibleHeight () const |
int | visibleWidth () const |
int | zoomLevel () const |
![]() | |
KHTMLWidget () | |
~KHTMLWidget () | |
Protected Slots | |
void | slotPaletteChanged () |
Protected Member Functions | |
void | clear () |
virtual void | closeEvent (QCloseEvent *) |
void | doAutoScroll () |
virtual void | dragEnterEvent (QDragEnterEvent *) |
virtual void | dropEvent (QDropEvent *) |
virtual bool | event (QEvent *event) |
virtual bool | eventFilter (QObject *, QEvent *) |
virtual void | focusInEvent (QFocusEvent *) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | hideEvent (QHideEvent *) |
void | keyPressEvent (QKeyEvent *_ke) |
void | keyReleaseEvent (QKeyEvent *_ke) |
virtual void | mouseDoubleClickEvent (QMouseEvent *) |
virtual void | mouseMoveEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
virtual void | paintEvent (QPaintEvent *) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | scrollContentsBy (int dx, int dy) |
void | setSmoothScrollingModeDefault (SmoothScrollingMode m) |
virtual void | showEvent (QShowEvent *) |
void | timerEvent (QTimerEvent *) |
virtual bool | viewportEvent (QEvent *e) |
virtual void | wheelEvent (QWheelEvent *) |
virtual bool | widgetEvent (QEvent *) |
Friends | |
void | khtml::applyRule (DOM::CSSProperty *prop) |
Additional Inherited Members | |
![]() | |
KHTMLWidgetPrivate * | m_kwp |
Detailed Description
Renders and displays HTML in a QScrollArea.
Suitable for use as an application's main view.
Definition at line 92 of file khtmlview.h.
Member Enumeration Documentation
Smooth Scrolling Mode enumeration.
- SSMDisabled smooth scrolling is disabled
- SSMWhenEfficient only use smooth scrolling on pages that do not require a full repaint of the content area when scrolling
- SSMAlways smooth scrolling is performed unconditionally
Enumerator | |
---|---|
SSMDisabled | |
SSMWhenEfficient | |
SSMEnabled |
Definition at line 305 of file khtmlview.h.
Constructor & Destructor Documentation
Constructs a KHTMLView.
Definition at line 551 of file khtmlview.cpp.
|
virtual |
Definition at line 565 of file khtmlview.cpp.
Member Function Documentation
void KHTMLView::addChild | ( | QWidget * | child, |
int | dx, | ||
int | dy | ||
) |
Definition at line 3954 of file khtmlview.cpp.
|
protected |
Definition at line 623 of file khtmlview.cpp.
|
signal |
|
protectedvirtual |
Definition at line 1064 of file khtmlview.cpp.
int KHTMLView::contentsHeight | ( | ) | const |
Returns the contents area's height.
Definition at line 678 of file khtmlview.cpp.
Returns a point translated to viewport coordinates.
- Parameters
-
p the contents area point to translate
Definition at line 749 of file khtmlview.cpp.
void KHTMLView::contentsToViewport | ( | int | x, |
int | y, | ||
int & | cx, | ||
int & | cy | ||
) | const |
Returns a point translated to viewport coordinates.
- Parameters
-
x x coordinate of contents area point to translate y y coordinate of contents area point to translate cx resulting x coordinate cy resulting y coordinate
Definition at line 754 of file khtmlview.cpp.
int KHTMLView::contentsWidth | ( | ) | const |
Returns the contents area's width.
Definition at line 673 of file khtmlview.cpp.
int KHTMLView::contentsX | ( | ) | const |
Returns the x coordinate of the contents area point that is currently located at the top left in the viewport.
Definition at line 692 of file khtmlview.cpp.
int KHTMLView::contentsY | ( | ) | const |
Returns the y coordinate of the contents area point that is currently located at the top left in the viewport.
Definition at line 697 of file khtmlview.cpp.
void KHTMLView::displayAccessKeys | ( | ) |
Display all accesskeys in small tooltips.
Definition at line 2504 of file khtmlview.cpp.
|
protected |
Definition at line 1889 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 3718 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 3724 of file khtmlview.cpp.
Definition at line 483 of file khtmlview.cpp.
Definition at line 2026 of file khtmlview.cpp.
|
signal |
|
signal |
This signal is used for internal layouting.
Don't use it to check if rendering finished. Use KHTMLPart completed() signal instead.
|
protectedvirtual |
Definition at line 3730 of file khtmlview.cpp.
Definition at line 1870 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 3741 of file khtmlview.cpp.
|
inline |
Definition at line 137 of file khtmlview.h.
|
signal |
|
protectedvirtual |
Definition at line 643 of file khtmlview.cpp.
|
protected |
Definition at line 1678 of file khtmlview.cpp.
|
protected |
Definition at line 1828 of file khtmlview.cpp.
|
slot |
ensure the display is up to date
Definition at line 956 of file khtmlview.cpp.
|
inline |
Returns the margin height.
A return value of -1 means the default value will be used.
Definition at line 161 of file khtmlview.h.
|
inline |
Returns the margin width.
A return value of -1 means the default value will be used.
Definition at line 149 of file khtmlview.h.
|
protectedvirtual |
Definition at line 1244 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 1303 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 1115 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 1547 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 871 of file khtmlview.cpp.
|
inline |
Returns a pointer to the KHTMLPart that is rendering the page.
Definition at line 135 of file khtmlview.h.
void KHTMLView::print | ( | bool | quick = false | ) |
Prints the HTML document.
- Parameters
-
quick if true, fully automated printing, without print dialog
Definition at line 3029 of file khtmlview.cpp.
|
signal |
void KHTMLView::repaintContents | ( | const QRect & | r | ) |
Requests an immediate repaint of the content area.
- Parameters
-
r the content area rectangle to repaint
Definition at line 802 of file khtmlview.cpp.
void KHTMLView::repaintContents | ( | int | x, |
int | y, | ||
int | w, | ||
int | h | ||
) |
Definition at line 791 of file khtmlview.cpp.
|
virtualslot |
Resize the contents area.
- Parameters
-
w the new width h the new height
Definition at line 683 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 839 of file khtmlview.cpp.
void KHTMLView::scrollBy | ( | int | x, |
int | y | ||
) |
Scrolls the content area by a given amount.
- Parameters
-
x x offset y y offset
Definition at line 741 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 3754 of file khtmlview.cpp.
void KHTMLView::setContentsPos | ( | int | x, |
int | y | ||
) |
Place the contents area point x/y at the top left of the viewport.
Definition at line 734 of file khtmlview.cpp.
|
virtual |
Sets horizontal scrollbar mode.
WARNING: do not call this method on a base class pointer unless you specifically want QAbstractScrollArea's variant (not recommended). QAbstractScrollArea::setHorizontalScrollBarPolicy is not virtual.
Definition at line 3379 of file khtmlview.cpp.
void KHTMLView::setMarginHeight | ( | int | y | ) |
Definition at line 950 of file khtmlview.cpp.
void KHTMLView::setMarginWidth | ( | int | x | ) |
Sets a margin in x direction.
Definition at line 944 of file khtmlview.cpp.
void KHTMLView::setSmoothScrollingMode | ( | SmoothScrollingMode | m | ) |
Set the smooth scrolling mode.
Smooth scrolling mode is normally controlled by the configuration file's SmoothScrolling key. Using this setter will override the configuration file's settings.
- Since
- 4.1
Definition at line 1087 of file khtmlview.cpp.
|
protected |
Definition at line 1095 of file khtmlview.cpp.
|
virtual |
Sets vertical scrollbar mode.
WARNING: do not call this method on a base class pointer unless you specifically want QAbstractScrollArea's variant (not recommended). QAbstractScrollArea::setVerticalScrollBarPolicy is not virtual.
Definition at line 3369 of file khtmlview.cpp.
void KHTMLView::setZoomLevel | ( | int | percent | ) |
Apply a zoom level to the content area.
- Parameters
-
percent a zoom level expressed as a percentage
Definition at line 1070 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 648 of file khtmlview.cpp.
|
protectedslot |
Definition at line 3217 of file khtmlview.cpp.
KHTMLView::SmoothScrollingMode KHTMLView::smoothScrollingMode | ( | ) | const |
|
protected |
Definition at line 3967 of file khtmlview.cpp.
void KHTMLView::updateContents | ( | const QRect & | r | ) |
Requests an update of the content area.
- Parameters
-
r the content area rectangle to update
Definition at line 786 of file khtmlview.cpp.
void KHTMLView::updateContents | ( | int | x, |
int | y, | ||
int | w, | ||
int | h | ||
) |
Definition at line 775 of file khtmlview.cpp.
Definition at line 1981 of file khtmlview.cpp.
Returns a point translated to contents area coordinates.
- Parameters
-
p the viewport point to translate
Definition at line 762 of file khtmlview.cpp.
void KHTMLView::viewportToContents | ( | int | x, |
int | y, | ||
int & | cx, | ||
int & | cy | ||
) | const |
Returns a point translated to contents area coordinates.
- Parameters
-
x x coordinate of viewport point to translate y y coordinate of viewport point to translate cx resulting x coordinate cy resulting y coordinate
Definition at line 767 of file khtmlview.cpp.
int KHTMLView::visibleHeight | ( | ) | const |
Returns the height of the viewport.
Definition at line 718 of file khtmlview.cpp.
int KHTMLView::visibleWidth | ( | ) | const |
Returns the width of the viewport.
Definition at line 702 of file khtmlview.cpp.
|
protectedvirtual |
Definition at line 3638 of file khtmlview.cpp.
Definition at line 2197 of file khtmlview.cpp.
int KHTMLView::zoomLevel | ( | ) | const |
Retrieve the current zoom level.
Definition at line 1082 of file khtmlview.cpp.
|
signal |
Friends And Related Function Documentation
|
friend |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.