KHTML
#include <kjavaapplet.h>
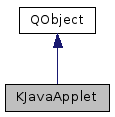
Public Types | |
enum | AppletState { UNKNOWN = 0, CLASS_LOADED = 1, INSTANCIATED = 2, INITIALIZED = 3, STARTED = 4, STOPPED = 5, DESTROYED = 6 } |
Signals | |
void | jsEvent (const QStringList &args) |
Detailed Description
Definition at line 50 of file kjavaapplet.h.
Member Enumeration Documentation
Enumerator | |
---|---|
UNKNOWN | |
CLASS_LOADED | |
INSTANCIATED | |
INITIALIZED | |
STARTED | |
STOPPED | |
DESTROYED |
Definition at line 57 of file kjavaapplet.h.
Constructor & Destructor Documentation
KJavaApplet::KJavaApplet | ( | KJavaAppletWidget * | _parent, |
KJavaAppletContext * | _context = 0 |
||
) |
Definition at line 49 of file kjavaapplet.cpp.
KJavaApplet::~KJavaApplet | ( | ) |
Definition at line 64 of file kjavaapplet.cpp.
Member Function Documentation
QString & KJavaApplet::appletClass | ( | ) |
Get the name of the Class file the applet should run.
Definition at line 88 of file kjavaapplet.cpp.
int KJavaApplet::appletId | ( | ) |
Returns the unique ID this applet is given.
Definition at line 202 of file kjavaapplet.cpp.
QString & KJavaApplet::appletName | ( | ) |
Get the name the applet should be called in its context.
Definition at line 175 of file kjavaapplet.cpp.
QString & KJavaApplet::archives | ( | ) |
Get the list of Archives that should be searched for class files and other resources.
Definition at line 143 of file kjavaapplet.cpp.
|
inline |
Definition at line 222 of file kjavaapplet.h.
QString & KJavaApplet::baseURL | ( | ) |
get the Base URL of the document embedding the applet
Definition at line 113 of file kjavaapplet.cpp.
QString & KJavaApplet::codeBase | ( | ) |
Get the codebase of the applet classes.
Definition at line 123 of file kjavaapplet.cpp.
void KJavaApplet::create | ( | ) |
Send message to AppletServer to create this applet's frame to be swallowed and download the applet classes.
Definition at line 180 of file kjavaapplet.cpp.
bool KJavaApplet::failed | ( | ) | const |
Definition at line 283 of file kjavaapplet.cpp.
|
inline |
Definition at line 204 of file kjavaapplet.h.
Get a reference to the Parameters and their values.
Definition at line 103 of file kjavaapplet.cpp.
QString & KJavaApplet::getWindowName | ( | ) |
Get the window title this applet should use.
Definition at line 170 of file kjavaapplet.cpp.
void KJavaApplet::init | ( | ) |
Send message to AppletServer to Initialize and show this applet.
Definition at line 187 of file kjavaapplet.cpp.
bool KJavaApplet::isAlive | ( | ) | const |
Definition at line 271 of file kjavaapplet.cpp.
bool KJavaApplet::isCreated | ( | ) |
Returns status of applet- whether it's been created or not.
Definition at line 72 of file kjavaapplet.cpp.
|
inline |
JavaScript coming from Java.
Definition at line 237 of file kjavaapplet.h.
|
signal |
Look up the parameter value for the given Parameter.
Returns QString() if the name has not been set.
Definition at line 93 of file kjavaapplet.cpp.
|
inline |
Definition at line 216 of file kjavaapplet.h.
void KJavaApplet::resizeAppletWidget | ( | int | width, |
int | height | ||
) |
Interface for applets to resize themselves.
Definition at line 148 of file kjavaapplet.cpp.
void KJavaApplet::setAppletClass | ( | const QString & | clazzName | ) |
Specify the name of the class file to run.
For example 'Lake.class'.
Definition at line 83 of file kjavaapplet.cpp.
void KJavaApplet::setAppletContext | ( | KJavaAppletContext * | _context | ) |
Set the applet context'.
Definition at line 77 of file kjavaapplet.cpp.
void KJavaApplet::setAppletId | ( | int | id | ) |
Set the applet ID.
Definition at line 207 of file kjavaapplet.cpp.
void KJavaApplet::setAppletName | ( | const QString & | name | ) |
Set the name the applet should be called in its context.
Definition at line 160 of file kjavaapplet.cpp.
void KJavaApplet::setArchives | ( | const QString & | _archives | ) |
Set the list of archives at the Applet's codebase to search in for class files and other resources.
Definition at line 138 of file kjavaapplet.cpp.
|
inline |
Get/Set the auth name.
Definition at line 221 of file kjavaapplet.h.
void KJavaApplet::setBaseURL | ( | const QString & | base | ) |
Set the URL of the document embedding the applet.
Definition at line 108 of file kjavaapplet.cpp.
void KJavaApplet::setCodeBase | ( | const QString & | codeBase | ) |
Set the codebase of the applet classes.
Definition at line 118 of file kjavaapplet.cpp.
void KJavaApplet::setFailed | ( | ) |
Definition at line 267 of file kjavaapplet.cpp.
Specify a parameter to be passed to the applet.
Definition at line 98 of file kjavaapplet.cpp.
|
inline |
Get/Set the user password.
Definition at line 215 of file kjavaapplet.h.
void KJavaApplet::setSize | ( | QSize | size | ) |
Set the size of the applet.
Definition at line 128 of file kjavaapplet.cpp.
|
inline |
Get/Set the user name.
Definition at line 209 of file kjavaapplet.h.
void KJavaApplet::setWindowName | ( | const QString & | title | ) |
Set the window title for swallowing.
Definition at line 165 of file kjavaapplet.cpp.
QSize KJavaApplet::size | ( | ) |
Get the size of the applet.
Definition at line 133 of file kjavaapplet.cpp.
void KJavaApplet::start | ( | ) |
Run the applet.
Definition at line 192 of file kjavaapplet.cpp.
KJavaApplet::AppletState KJavaApplet::state | ( | ) | const |
Definition at line 279 of file kjavaapplet.cpp.
void KJavaApplet::stateChange | ( | const int | newState | ) |
called from the protocol engine changes the status according to the one on the java side.
Do not call this yourself!
Definition at line 212 of file kjavaapplet.cpp.
void KJavaApplet::stop | ( | ) |
Pause the applet.
Definition at line 197 of file kjavaapplet.cpp.
|
inline |
Definition at line 210 of file kjavaapplet.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:51:23 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.