KNewStuff
#include <coreengine.h>
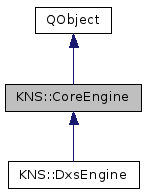
Public Types | |
enum | AutomationPolicy { AutomationOn, AutomationOff } |
enum | CachePolicy { CacheNever, CacheReplaceable, CacheResident, CacheOnly } |
Signals | |
void | signalDownloadDialogDone (KNS::Entry::List) |
void | signalEntriesFailed () |
void | signalEntriesFeedFinished (const KNS::Feed *feed) |
void | signalEntriesFinished () |
void | signalEntryChanged (KNS::Entry *entry) |
void | signalEntryFailed () |
void | signalEntryLoaded (KNS::Entry *entry, const KNS::Feed *feed, const KNS::Provider *provider) |
void | signalEntryRemoved (KNS::Entry *entry, const KNS::Feed *feed) |
void | signalEntryUploaded () |
void | signalInstallationFailed () |
void | signalInstallationFinished () |
void | signalPayloadFailed (KNS::Entry *entry) |
void | signalPayloadLoaded (KUrl payload) |
void | signalPreviewFailed () |
void | signalPreviewLoaded (KUrl preview) |
void | signalProgress (const QString &message, int percentage) |
void | signalProviderChanged (KNS::Provider *provider) |
void | signalProviderLoaded (KNS::Provider *provider) |
void | signalProvidersFailed () |
void | signalProvidersFinished () |
Public Member Functions | |
CoreEngine (QObject *parent) | |
~CoreEngine () | |
QString | componentName () const |
void | downloadPayload (Entry *entry) |
void | downloadPreview (Entry *entry) |
bool | init (const QString &configfile) |
bool | install (const QString &payloadfile) |
void | loadEntries (Provider *provider) |
void | setAutomationPolicy (AutomationPolicy policy) |
void | setCachePolicy (CachePolicy policy) |
void | start () |
bool | uninstall (KNS::Entry *entry) |
bool | uploadEntry (Provider *provider, Entry *entry) |
Protected Member Functions | |
void | mergeEntries (Entry::List entries, Feed *feed, const Provider *provider) |
Detailed Description
KNewStuff core engine.
A core engine keeps track of data which is available locally and remote and offers high-level synchronization calls as well as upload and download primitives using an underlying GHNS protocol.
Definition at line 47 of file coreengine.h.
Member Enumeration Documentation
Engine automation can be activated to let the engine take care by itself of all the method calls needed in a workflow.
For example, the download workflow will require entries to be loaded after the providers, and preview images for all entries afterwards.
Calling the methods for those load operations is necessary when automation is off, but it is redundant (and in fact considered an error) when automation is switched on.
The default automation policy is AutomationOff.
Enumerator | |
---|---|
AutomationOn |
Turn on automation, and take care of method calls. Turn off automation, and let the application call the methods. (default) |
AutomationOff |
Definition at line 98 of file coreengine.h.
Policy on how to cache the data received from the network.
While CacheNever completely switches off all caching, the other two settings CacheReplaceable and CacheResident will optimize the network traffic needed for all workflows. CacheOnly will never download from the network at all and can be used to inspect the local cache.
Provider files, feeds, entries and preview images are subject to this policy.
The default cache policy is CacheNever.
Definition at line 75 of file coreengine.h.
Constructor & Destructor Documentation
CoreEngine::CoreEngine | ( | QObject * | parent | ) |
Constructor.
Definition at line 57 of file coreengine.cpp.
CoreEngine::~CoreEngine | ( | ) |
Destructor.
Frees up all the memory again which might be taken by cached entries and providers.
Definition at line 63 of file coreengine.cpp.
Member Function Documentation
QString CoreEngine::componentName | ( | ) | const |
- Returns
- the component name the engine is using, or an empty string if not initialized yet
Definition at line 186 of file coreengine.cpp.
void CoreEngine::downloadPayload | ( | Entry * | entry | ) |
Downloads a payload file.
The payload file matching most closely the current user language preferences will be downloaded. The file will not be installed set, for this install must be called.
- Parameters
-
entry Entry to download payload file for
Definition at line 300 of file coreengine.cpp.
void CoreEngine::downloadPreview | ( | Entry * | entry | ) |
Downloads a preview file.
The preview file matching most closely the current user language preferences will be downloaded.
This method should not be called if automation is activated.
- Parameters
-
entry Entry to download preview image for
Definition at line 269 of file coreengine.cpp.
Initializes the engine.
This step is application-specific and relies on an external configuration file, which determines all the details about the initialization.
- Parameters
-
configfile KNewStuff2 configuration file (*.knsrc)
- Returns
- true if any valid configuration was found, false otherwise
Definition at line 68 of file coreengine.cpp.
Installs an entry's payload file.
This includes verification, if necessary, as well as decompression and other steps according to the application's *.knsrc file. Note that this method is asynchronous and thus the return value will only report the successful start of the installation.
- Parameters
-
payloadfile Path to file to install
- Returns
- Whether or not installation was started successfully
- Note
- FIXME: use Entry as parameter
Definition at line 1333 of file coreengine.cpp.
void CoreEngine::loadEntries | ( | Provider * | provider | ) |
Loads all entries of all the feeds from a provider.
This means that meta information about those entries is retrieved from the cache and/or from the network, depending on the cache policy.
This method should not be called if automation is activated.
- Parameters
-
provider Provider from where to load the entries
- See also
- signalEntryLoaded
- signalEntryChanged
- signalEntriesFailed
- signalEntriesFinished
- signalEntriesFeedFinished
Definition at line 231 of file coreengine.cpp.
|
protected |
Definition at line 1036 of file coreengine.cpp.
void CoreEngine::setAutomationPolicy | ( | AutomationPolicy | policy | ) |
Definition at line 1640 of file coreengine.cpp.
void CoreEngine::setCachePolicy | ( | CachePolicy | policy | ) |
Definition at line 1645 of file coreengine.cpp.
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
Indicates that the list of providers has been successfully loaded.
This signal might occur twice, for the local cache and for updated provider information from the ProvidersUrl.
|
signal |
|
signal |
void CoreEngine::start | ( | ) |
Starts the engine.
This method reports all cached and registered providers to the application. Depending on the cache policy, the engine will then try to synchronize the cache by updating all information about the providers.
If engine automation is activated, this method will proceed to synchronize all feeds, entries and preview images. For each provider, all feeds are considered. The synchronization is complete if signalEntriesFinished is emitted, but applications should continue watching signalEntryChanged.
- See also
- signalProviderLoaded
- signalProviderChanged
- signalProvidersFailed
- signalProvidersFinished
- signalEntryLoaded
- signalEntryChanged
- signalEntriesFailed
- signalEntriesFinished
- signalEntriesFeedFinished
- signalPreviewLoaded
- signalPreviewFailed
Definition at line 195 of file coreengine.cpp.
bool CoreEngine::uninstall | ( | KNS::Entry * | entry | ) |
Uninstalls an entry.
It reverses the steps which were performed during the installation.
- Parameters
-
entry The entry to deinstall
- Returns
- Whether or not deinstallation was successful
- Note
- FIXME: I don't believe this works yet :)
Definition at line 1576 of file coreengine.cpp.
Uploads a complete entry, including its payload and preview files (if present) and all associated meta information.
Note that this method is asynchronous and thus the return value will not report the final success of all upload steps. It will merely check that the provider supports upload and so forth.
- Returns
- Whether or not upload was started successfully
- See also
- signalEntryUploaded
- signalEntryFailed
Definition at line 340 of file coreengine.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:49 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.