KParts
#include <scriptableextension.h>
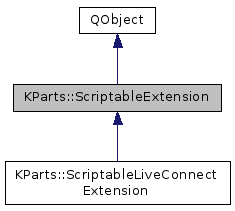
Classes | |
struct | Exception |
struct | FunctionRef |
struct | Null |
struct | Object |
struct | Undefined |
lifetime | |
ScriptableExtension (QObject *parent) | |
virtual | ~ScriptableExtension () |
static ScriptableExtension * | childObject (QObject *obj) |
static ScriptableExtension * | adapterFromLiveConnect (QObject *parentObj, LiveConnectExtension *oldApi) |
Object Hierarchy | |
void | setHost (ScriptableExtension *host) |
ScriptableExtension * | host () const |
virtual QVariant | rootObject () |
QVariant | enclosingObject () |
Object Operations | |
All these methods share the following conventions:
| |
enum | ScriptLanguage { ECMAScript, EnumLimit = 0xFFFF } |
typedef QList< QVariant > | ArgList |
virtual QVariant | callAsFunction (ScriptableExtension *callerPrincipal, quint64 objId, const ArgList &args) |
virtual QVariant | callFunctionReference (ScriptableExtension *callerPrincipal, quint64 objId, const QString &f, const ArgList &args) |
virtual QVariant | callAsConstructor (ScriptableExtension *callerPrincipal, quint64 objId, const ArgList &args) |
virtual bool | hasProperty (ScriptableExtension *callerPrincipal, quint64 objId, const QString &propName) |
virtual QVariant | get (ScriptableExtension *callerPrincipal, quint64 objId, const QString &propName) |
virtual bool | put (ScriptableExtension *callerPrincipal, quint64 objId, const QString &propName, const QVariant &value) |
virtual bool | removeProperty (ScriptableExtension *callerPrincipal, quint64 objId, const QString &propName) |
virtual bool | enumerateProperties (ScriptableExtension *callerPrincipal, quint64 objId, QStringList *result) |
virtual bool | setException (ScriptableExtension *callerPrincipal, const QString &message) |
virtual QVariant | evaluateScript (ScriptableExtension *callerPrincipal, quint64 contextObjectId, const QString &code, ScriptLanguage language=ECMAScript) |
virtual bool | isScriptLanguageSupported (ScriptLanguage lang) const |
virtual void | acquire (quint64 objid) |
virtual void | release (quint64 objid) |
static QVariant | acquireValue (const QVariant &v) |
static QVariant | releaseValue (const QVariant &v) |
Detailed Description
An extension class that permits KParts to be scripted (such as when embedded inside a KHTMLPart) and to access the host's scriptable objects as well.
See Script Value Types for how values are passed to/from various methods here.
- Since
- 4.5
Definition at line 44 of file scriptableextension.h.
Member Typedef Documentation
Definition at line 192 of file scriptableextension.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ECMAScript | |
EnumLimit |
< also known as JavaScript |
Definition at line 247 of file scriptableextension.h.
Constructor & Destructor Documentation
|
protected |
Definition at line 34 of file scriptableextension.cpp.
|
virtual |
Definition at line 38 of file scriptableextension.cpp.
Member Function Documentation
|
virtual |
increases reference count of object objId
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 190 of file scriptableextension.cpp.
Helper that calls acquire on any object or function reference base stored in v
.
- Returns
- a copy of the passed in value
Definition at line 195 of file scriptableextension.cpp.
|
static |
This returns a bridge object that permits KParts implementing the older LiveConnectExtension to be used via the ScriptableExtension API.
The bridge's parent will be the parentObj
.
Definition at line 48 of file scriptableextension.cpp.
|
virtual |
Try to use the object objId
associated with 'this' as a constructor (corresponding to ECMAScript's new foo(bar, baz, glarch) expression).
Definition at line 109 of file scriptableextension.cpp.
|
virtual |
Try to use the object objId
associated with 'this' as a function.
Definition at line 89 of file scriptableextension.cpp.
|
virtual |
Try to use a function reference to field f
of object as a function.
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 98 of file scriptableextension.cpp.
|
static |
Queries obj
for a child object which inherits from this ScriptableExtension class.
Convenience method.
Definition at line 43 of file scriptableextension.cpp.
QVariant KParts::ScriptableExtension::enclosingObject | ( | ) |
Returns an object that represents the host()'s view of us.
For example, if the host is an HTML part, it would return a DOM node of an <object> handled by this part. May be undefined or null
Implemented in terms of objectForKid
Definition at line 69 of file scriptableextension.cpp.
|
virtual |
Tries to enumerate all fields of object objId
associated with this to result
.
Returns true on success
Definition at line 155 of file scriptableextension.cpp.
|
virtual |
Tries to evaluate a script code
with the given object as its context.
The parameter language
specifies the language to execute it as. Use isScriptLanguageSupported to check for support.
Definition at line 172 of file scriptableextension.cpp.
|
virtual |
Tries to get field propName
from object objId
associated with 'this'.
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 127 of file scriptableextension.cpp.
|
virtual |
Returns true if the object objId
associated with 'this' has the property propName
.
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 118 of file scriptableextension.cpp.
ScriptableExtension * KParts::ScriptableExtension::host | ( | ) | const |
Returns any registered parent scripting context.
May be 0 if setHost was not called (or not call yet).
Definition at line 59 of file scriptableextension.cpp.
|
virtual |
returns true if this extension can execute scripts in the given language
Definition at line 184 of file scriptableextension.cpp.
|
virtual |
Tries to set the field propName
from object objId
associated with 'this' to value
.
Returns true on success
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 136 of file scriptableextension.cpp.
|
virtual |
decreases reference count of object objId
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 207 of file scriptableextension.cpp.
Helper that calls release on any object or function reference base stored in v
.
- Returns
- a copy of the passed in value
Definition at line 212 of file scriptableextension.cpp.
|
virtual |
Tries to remove the field d propName
from object objId
associated with 'this'.
Returns true on success
Definition at line 146 of file scriptableextension.cpp.
|
virtual |
Return the root scriptable object of this KPart.
For example for an HTML part, it would represent a Window object. May be undefined or null
Reimplemented in KParts::ScriptableLiveConnectExtension.
Definition at line 64 of file scriptableextension.cpp.
|
virtual |
Tries to raise an exception with given message in this extension's scripting context.
Returns true on success
Definition at line 164 of file scriptableextension.cpp.
void KParts::ScriptableExtension::setHost | ( | ScriptableExtension * | host | ) |
Reports the hosting ScriptableExtension to a child.
It's the responsibility of a parent part to call this method on all of its kids' ScriptableExtensions as soon as possible.
Definition at line 54 of file scriptableextension.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.