Kross
Kross::FormAssistant Class Reference
#include <form.h>
Inheritance diagram for Kross::FormAssistant:
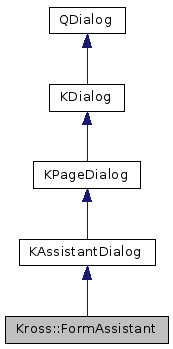
Public Types | |
enum | AssistantButtonCode { None = 0x00000000, Help = 0x00000001, Default = 0x00000002, Cancel = 0x00000020, Finish = 0x00001000, Next = 0x00002000, Back = 0x00004000, NoDefault = 0x00008000 } |
![]() | |
enum | FaceType |
Public Slots | |
QWidget * | addPage (const QString &name, const QString &header=QString(), const QString &iconname=QString()) |
void | back () |
QString | currentPage () const |
int | exec () |
int | exec_loop () |
bool | isAppropriate (const QString &name) const |
bool | isValid (const QString &name) const |
void | next () |
QWidget * | page (const QString &name) const |
QString | result () |
void | setAppropriate (const QString &name, bool appropriate) |
bool | setCurrentPage (const QString &name) |
void | setValid (const QString &name, bool enable) |
void | showHelpButton (bool) |
![]() | |
virtual void | back () |
virtual void | next () |
Signals | |
void | backClicked () |
void | nextClicked () |
![]() | |
void | currentPageChanged (KPageWidgetItem *current, KPageWidgetItem *before) |
void | pageRemoved (KPageWidgetItem *page) |
Additional Inherited Members | |
![]() | |
KAssistantDialog (KPageWidget *widget, QWidget *parent=0, Qt::WindowFlags flags=0) | |
KPageDialog (KPageDialogPrivate &dd, KPageWidget *widget, QWidget *parent, Qt::WindowFlags flags=0) | |
KPageDialog (KPageWidget *widget, QWidget *parent, Qt::WindowFlags flags=0) | |
KPageWidget * | pageWidget () |
const KPageWidget * | pageWidget () const |
void | setPageWidget (KPageWidget *widget) |
virtual void | showEvent (QShowEvent *event) |
Detailed Description
The FormAssistant class provides access to KAssistantDialog objects as top-level containers.
Example (in Python) :
import Kross
import sys,os
ourPath=(filter(lambda p: os.path.exists(p+'/mywidget.ui'),sys.path)+[''])[0]
forms = Kross.module("forms")
myassistant = forms.createAssistant("MyAssistant")
myassistant.showHelpButton(0)
mypage = myassistant.addPage("name","header")
mywidget = forms.createWidgetFromUIFile(mypage, ourPath+'/mywidget.ui')
mypage2 = myassistant.addPage("name2","header2")
mywidget2 = forms.createWidgetFromUIFile(mypage2, ourPath+'/mywidget.ui')
mypage3 = myassistant.addPage("name3","header3")
mywidget3 = forms.createWidgetFromUIFile(mypage3, ourPath+'/mywidget.ui')
mywidget["lineEdit"].setText("some string")
def nextClicked():
myassistant.setAppropriate("name2",0)
def finished():
...
myassistant.deleteLater() #remember to cleanup
myassistant.connect("nextClicked()",nextClicked)
myassistant.connect("finished()",finished)
myassistant.show()
Member Enumeration Documentation
Constructor & Destructor Documentation
Member Function Documentation
|
slot |
Add and return a new page.
- Parameters
-
name The name the page has. This name is for example returned at the currentPage() method and should be unique. The name is also used to display a short title for the page. header The longer header title text used for display purposes. iconname The name of the icon which the page have. This could be for example "about_kde", "document-open", "configure" or any other iconname known by KDE.
- Returns
- the new QWidget page instance.
|
slot |
|
signal |
|
slot |
|
inlineslot |
Shows the dialog as a modal dialog, blocking until the user closes it and returns the execution result.
- Returns
- >=1 if the dialog was accepted (e.g. "Finished" pressed) else the user rejected the dialog (e.g. by pressing "Cancel" or just closing the dialog by pressing the escape-key).
|
inlineslot |
- See also
- KAssistantDialog::isValid()
|
slot |
|
signal |
use it to setAppropriate()
|
slot |
- See also
- KAssistantDialog::setValid()
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.