Kross
#include <manager.h>
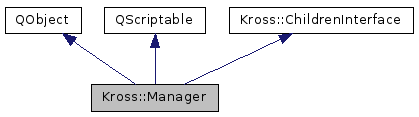
Public Slots | |
QObject * | action (const QString &name) |
void | addQObject (QObject *obj, const QString &name=QString()) |
void | deleteModules () |
bool | executeScriptFile (const QUrl &file=QUrl()) |
bool | hasAction (const QString &name) |
QStringList | interpreters () const |
QObject * | module (const QString &modulename) |
QObject * | qobject (const QString &name) const |
QStringList | qobjectNames () const |
Signals | |
void | finished (Kross::Action *) |
void | started (Kross::Action *) |
Static Public Member Functions | |
static Manager & | self () |
Additional Inherited Members | |
![]() | |
enum | Options { NoOption = 0x00, AutoConnectSignals = 0x01, LastOption = 0x1000000 } |
Detailed Description
The Manager class is a singleton that provides the main entry point to deal with the Kross Scripting Framework.
Use Interpreter to just work with some implementated interpreter like python or ruby. While Action implements a flexible abstract container to deal with single script files.
Constructor & Destructor Documentation
|
explicit |
The constructor.
Use self() to access the Manager singleton instance and don't call this direct.
Definition at line 121 of file manager.cpp.
|
virtual |
Destructor.
Definition at line 231 of file manager.cpp.
Member Function Documentation
ActionCollection * Manager::actionCollection | ( | ) | const |
- Returns
- the root ActionCollection instance. Each collection could have children of other collections and/or Action instances.
Definition at line 285 of file manager.cpp.
Definition at line 380 of file manager.cpp.
|
slot |
External modules are dynamically loadable and are normally deleted when the kross library is unloaded.
Applications may choose to call deleteModules() instead to control deletion of the modules at another time.
Definition at line 364 of file manager.cpp.
Execute a script file.
- Parameters
-
file The script file that should be executed.
Definition at line 370 of file manager.cpp.
|
signal |
This signal is emitted when the execution of a script is finished.
- Returns
- true if there exists a Action QObject instance which is child of this Manager instance and is defined as
name
else false is returned.
Definition at line 290 of file manager.cpp.
bool Manager::hasHandlerAssigned | ( | const QByteArray & | typeName | ) | const |
- Returns
- whether
typeName
has a handler assigned or not.
Definition at line 425 of file manager.cpp.
- Returns
- true if there exists an interpreter with the name
interpretername
else false.
Definition at line 245 of file manager.cpp.
Interpreter * Manager::interpreter | ( | const QString & | interpretername | ) | const |
Return the Interpreter instance defined by the interpretername.
- Parameters
-
interpretername The name of the interpreter. e.g. "python" or "kjs".
- Returns
- The Interpreter instance or NULL if there does not exists an interpreter with such an interpretername.
Definition at line 271 of file manager.cpp.
InterpreterInfo * Manager::interpreterInfo | ( | const QString & | interpretername | ) | const |
- Returns
- the InterpreterInfo* matching to the defined
interpretername
or NULL if there does not exists such a interpreter.
Definition at line 250 of file manager.cpp.
QHash< QString, InterpreterInfo * > Manager::interpreterInfos | ( | ) | const |
- Returns
- a map with InterpreterInfo* instances used to describe interpreters.
Definition at line 240 of file manager.cpp.
Return the name of the Interpreter that feels responsible for the defined file
.
- Parameters
-
file The filename we should try to determinate the interpretername for.
- Returns
- The name of the Interpreter which will be used to execute the file or QString() if we failed to determinate a matching interpreter for the file.
Definition at line 255 of file manager.cpp.
|
slot |
- Returns
- a list of names of all supported scripting interpreters. The list may contain for example "python", "ruby" and "kjs" depending on what interpreter-plugins are installed.
Definition at line 280 of file manager.cpp.
MetaTypeHandler * Manager::metaTypeHandler | ( | const QByteArray & | typeName | ) | const |
- Returns
- the MetaTypeHandler instance for custom types of type
typeName
.
- Since
- 4.2
Definition at line 395 of file manager.cpp.
Load and return an external module.
Modules are dynamic loadable plugins which could be loaded on demand to provide additional functionality.
- Parameters
-
modulename The name of the module we should try to load.
- Returns
- The QObject instance that repesents the module or NULL if loading failed.
Definition at line 307 of file manager.cpp.
Definition at line 385 of file manager.cpp.
|
slot |
Definition at line 390 of file manager.cpp.
void Manager::registerMetaTypeHandler | ( | const QByteArray & | typeName, |
MetaTypeHandler::FunctionPtr * | handler | ||
) |
Register a handler for custom types.
See also the WrapperInterface class.
- Parameters
-
typeName The custom type the handler should handle. handler Function that should be called to handle a custom type.
- Since
- 4.2
Definition at line 400 of file manager.cpp.
void Manager::registerMetaTypeHandler | ( | const QByteArray & | typeName, |
MetaTypeHandler::FunctionPtr2 * | handler | ||
) |
Register a handler for custom types.
See also the WrapperInterface class.
- Parameters
-
typeName The custom type the handler should handle. handler Function that should be called to handle a custom type.
- Since
- 4.2
Definition at line 405 of file manager.cpp.
void Manager::registerMetaTypeHandler | ( | const QByteArray & | typeName, |
MetaTypeHandler * | handler | ||
) |
Register a handler for custom types.
See also the WrapperInterface class.
- Parameters
-
typeName The custom type the handler should handle. handler Function that should be called to handle a custom type.
- Since
- 4.2
Definition at line 410 of file manager.cpp.
|
static |
Return the Manager instance.
Always use this function to access the Manager singleton.
Definition at line 73 of file manager.cpp.
void Manager::setStrictTypesEnabled | ( | bool | enabled | ) |
Enable more strict type handling.
If enabled then scripting-backends don't handle unknown pointer-types where no MetaTypeHandler was registered for. If disabled, such unknown types will be reinterpret_cast to QObject* what allows to also handle unknown QObject's but will also result in a crash if the unknown type isn't a QObject. Per default strict type handling is enabled.
- Since
- 4.2
Definition at line 420 of file manager.cpp.
|
signal |
This signal is emitted when the execution of a script is started.
bool Manager::strictTypesEnabled | ( | ) | const |
Returns true if strict type handling is enabled.
- Since
- 4.2
Definition at line 415 of file manager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.