KUtils
#include <kcmoduleproxy.h>
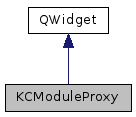
Public Slots | |
void | defaults () |
void | deleteClient () |
Signals | |
void | changed (bool state) |
void | changed (KCModuleProxy *mod) |
void | childClosed () |
void | quickHelpChanged () |
Public Member Functions | |
KCModuleProxy (const KCModuleInfo &info, QWidget *parent=0, const QStringList &args=QStringList()) | |
KCModuleProxy (const QString &serviceName, QWidget *parent=0, const QStringList &args=QStringList()) | |
KCModuleProxy (const KService::Ptr &service, QWidget *parent=0, const QStringList &args=QStringList()) | |
~KCModuleProxy () | |
const KAboutData * | aboutData () const |
KCModule::Buttons | buttons () const |
bool | changed () const |
KComponentData | componentData () const |
QString | dbusPath () const |
QString | dbusService () const |
void | load () |
QSize | minimumSizeHint () const |
KCModuleInfo | moduleInfo () const |
QString | quickHelp () const |
KCModule * | realModule () const |
QString | rootOnlyMessage () const |
void | save () |
bool | useRootOnlyMessage () const |
Protected Member Functions | |
void | showEvent (QShowEvent *) |
Protected Attributes | |
KCModuleProxyPrivate *const | d_ptr |
Detailed Description
Encapsulates a KCModule for embedding.
KCModuleProxy is a wrapper for KCModule intended for cases where modules are to be displayed. It ensures layout is consistent and in general takes care of the details needed for making a module available in an interface. A KCModuleProxy can be treated as a QWidget, without worrying about the details specific for modules such as library loading. KCModuleProxy is not a sub class of KCModule but its API closely resembles KCModule's.
Usually, an instance is created by passing one of the constructors a KService::Ptr, KCModuleInfo or simply the name of the module and then added to the layout as any other widget.
When the user has changed the module, changed(bool) as well as changed(KCModuleProxy *) is emitted. KCModuleProxy does not take care of prompting for saving - if the object is deleted while changes is not saved the changes will be lost. changed() returns true if changes are unsaved.
KCModuleProxy does not take care of authorization of KCModules.
KCModuleProxy implements lazy loading, meaning the library will not be loaded or any other initialization done before its show() function is called. This means modules will only be loaded when they are actually needed as well as it is possible to load many KCModuleProxy without any speed penalty.
KCModuleProxy should be used in all cases where modules are embedded in order to promote code efficiency and usability consistency.
Definition at line 67 of file kcmoduleproxy.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KCModuleProxy from a KCModuleInfo class.
- Parameters
-
info The KCModuleInfo to construct the module from. parent the parent QWidget. args This is used in the implementation and is internal. Use the default.
Definition at line 250 of file kcmoduleproxy.cpp.
|
explicit |
Constructs a KCModuleProxy from a module's service name, which is equivalent to the desktop file for the kcm without the ".desktop" part.
Otherwise equal to the one above.
- Parameters
-
serviceName The module's service name to construct from. parent the parent QWidget. args This is used in the implementation and is internal. Use the default.
Definition at line 257 of file kcmoduleproxy.cpp.
|
explicit |
Constructs a KCModuleProxy from KService.
Otherwise equal to the one above.
- Parameters
-
service The KService to construct from. parent the parent QWidget. args This is used in the implementation and is internal. Use the default.
Definition at line 243 of file kcmoduleproxy.cpp.
KCModuleProxy::~KCModuleProxy | ( | ) |
Default destructor.
Definition at line 209 of file kcmoduleproxy.cpp.
Member Function Documentation
const KAboutData * KCModuleProxy::aboutData | ( | ) | const |
- Returns
- the module's aboutData()
Definition at line 297 of file kcmoduleproxy.cpp.
KCModule::Buttons KCModuleProxy::buttons | ( | ) | const |
- Returns
- what buttons the module needs
Definition at line 302 of file kcmoduleproxy.cpp.
bool KCModuleProxy::changed | ( | ) | const |
- Returns
- true if the module is modified and needs to be saved.
Definition at line 324 of file kcmoduleproxy.cpp.
|
signal |
|
signal |
This is emitted in the same situations as in the one above.
Practical when several KCModuleProxys are loaded.
|
signal |
When a module running with root privileges and exits, returns to normal mode, the childClosed() signal is emitted.
KComponentData KCModuleProxy::componentData | ( | ) | const |
Returns the embedded KCModule's KComponentData.
- Returns
- The module's KComponentData.
Definition at line 319 of file kcmoduleproxy.cpp.
QString KCModuleProxy::dbusPath | ( | ) | const |
Returns the DBUS Path.
Definition at line 342 of file kcmoduleproxy.cpp.
QString KCModuleProxy::dbusService | ( | ) | const |
Returns the DBUS Service name.
Definition at line 336 of file kcmoduleproxy.cpp.
|
slot |
Calling it will cause the contained module to load its default values.
Definition at line 285 of file kcmoduleproxy.cpp.
|
slot |
Calling this, results in deleting the contained module, and unregistering from DCOP.
A similar result is achieved by deleting the KCModuleProxy itself.
Definition at line 217 of file kcmoduleproxy.cpp.
void KCModuleProxy::load | ( | ) |
Calling it will cause the contained module to run its load() routine.
Definition at line 265 of file kcmoduleproxy.cpp.
QSize KCModuleProxy::minimumSizeHint | ( | ) | const |
Returns the recommended minimum size for the widget.
Definition at line 348 of file kcmoduleproxy.cpp.
KCModuleInfo KCModuleProxy::moduleInfo | ( | ) | const |
- Returns
- a KCModuleInfo for the encapsulated module
Definition at line 330 of file kcmoduleproxy.cpp.
QString KCModuleProxy::quickHelp | ( | ) | const |
- Returns
- the module's quickHelp();
Definition at line 292 of file kcmoduleproxy.cpp.
|
signal |
KCModule * KCModuleProxy::realModule | ( | ) | const |
Access to the actual module.
However, if the module is running in root mode, see rootMode(), this function returns a NULL pointer, since the module is in another process. It may also return NULL if anything goes wrong.
- Returns
- the encapsulated module.
Definition at line 71 of file kcmoduleproxy.cpp.
QString KCModuleProxy::rootOnlyMessage | ( | ) | const |
- Returns
- The module's custom root message, if it has one
Definition at line 309 of file kcmoduleproxy.cpp.
void KCModuleProxy::save | ( | ) |
Calling it will cause the contained module to run its save() routine.
If the module was not modified, it will not be asked to save.
Definition at line 275 of file kcmoduleproxy.cpp.
|
protected |
Reimplemented for internal purposes.
Makes sure the encapsulated module is loaded before the show event is taken care of.
Definition at line 194 of file kcmoduleproxy.cpp.
bool KCModuleProxy::useRootOnlyMessage | ( | ) | const |
- Returns
- If the module is a root module.
Definition at line 314 of file kcmoduleproxy.cpp.
Member Data Documentation
|
protected |
Definition at line 253 of file kcmoduleproxy.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.