Nepomuk-Core
#include <fileindexerconfig.h>
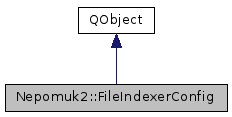
Public Slots | |
bool | forceConfigUpdate () |
void | setInitialRun (bool isInitialRun) |
Signals | |
void | configChanged () |
void | excludeFolderListChanged (const QStringList &added, const QStringList &removed) |
void | fileExcludeFiltersChanged () |
void | includeFolderListChanged (const QStringList &added, const QStringList &removed) |
void | mimeTypeFiltersChanged () |
Public Member Functions | |
FileIndexerConfig (QObject *parent=0) | |
~FileIndexerConfig () | |
QStringList | excludeFilters () const |
QStringList | excludeFolders () const |
bool | folderInFolderList (const QString &path) |
QList< QPair< QString, bool > > | folders () const |
QStringList | includeFolders () const |
bool | indexHiddenFilesAndFolders () const |
bool | initialUpdateDisabled () const |
bool | isDebugModeEnabled () const |
bool | isInitialRun () const |
KIO::filesize_t | minDiskSpace () const |
bool | shouldBeIndexed (const QString &path) const |
bool | shouldFileBeIndexed (const QString &fileName) const |
bool | shouldFolderBeIndexed (const QString &path) const |
bool | shouldMimeTypeBeIndexed (const QString &mimeType) const |
bool | suspendOnPowerSaveDisabled () const |
Static Public Member Functions | |
static FileIndexerConfig * | self () |
Detailed Description
Active config class which emits signals if the config was changed, for example if the KCM saved the config file.
Definition at line 38 of file fileindexerconfig.h.
Constructor & Destructor Documentation
Nepomuk2::FileIndexerConfig::FileIndexerConfig | ( | QObject * | parent = 0 | ) |
Create a new file indexr config.
The first instance to be created will be accessible via self().
Definition at line 57 of file fileindexerconfig.cpp.
Nepomuk2::FileIndexerConfig::~FileIndexerConfig | ( | ) |
Definition at line 77 of file fileindexerconfig.cpp.
Member Function Documentation
|
signal |
QStringList Nepomuk2::FileIndexerConfig::excludeFilters | ( | ) | const |
Definition at line 116 of file fileindexerconfig.cpp.
|
signal |
QStringList Nepomuk2::FileIndexerConfig::excludeFolders | ( | ) | const |
The folders that should be excluded.
Cached and cleaned up. It is perfectly possible to include subfolders again.
Definition at line 105 of file fileindexerconfig.cpp.
|
signal |
bool Nepomuk2::FileIndexerConfig::folderInFolderList | ( | const QString & | path | ) |
Returns true if the folder is in the list indexed directories and not in the list of exclude directories.
Definition at line 226 of file fileindexerconfig.cpp.
QList< QPair< QString, bool > > Nepomuk2::FileIndexerConfig::folders | ( | ) | const |
A cleaned up list of all include and exclude folders with their respective include/exclude flag sorted by path.
None of the paths have trailing slashes.
Definition at line 88 of file fileindexerconfig.cpp.
|
slot |
Reread the config from disk and update the configuration cache.
This is only required for testing as normally the config updates itself whenever the config file on disk changes.
- Returns
true
if the config has actually changed
Definition at line 448 of file fileindexerconfig.cpp.
|
signal |
QStringList Nepomuk2::FileIndexerConfig::includeFolders | ( | ) | const |
The folders to search for files to analyze.
Cached and cleaned up.
Definition at line 94 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::indexHiddenFilesAndFolders | ( | ) | const |
Definition at line 139 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::initialUpdateDisabled | ( | ) | const |
A "hidden" config option which allows to disable the initial update of all indexed folders.
This should be used in combination with isInitialRun() to make sure all folders are at least indexed once.
Definition at line 471 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::isDebugModeEnabled | ( | ) | const |
Check if the debug mode is enabled.
The debug mode is a hidden configuration option (without any GUI) that will make the indexer log errors in a dedicated file.
Definition at line 481 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::isInitialRun | ( | ) | const |
true the first time the service is run (or after manually tampering with the config.
Definition at line 159 of file fileindexerconfig.cpp.
|
signal |
KIO::filesize_t Nepomuk2::FileIndexerConfig::minDiskSpace | ( | ) | const |
The minimal available disk space.
If it drops below indexing will be suspended.
Definition at line 145 of file fileindexerconfig.cpp.
|
static |
Get the first created instance of FileIndexerConfig.
Definition at line 82 of file fileindexerconfig.cpp.
|
slot |
Should be called once the initial indexing is done, ie.
all folders have been indexed.
Definition at line 466 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::shouldBeIndexed | ( | const QString & | path | ) | const |
Check if path
should be indexed taking into account the includeFolders(), the excludeFolders(), and the excludeFilters().
Be aware that this method does not check if parent dirs match any of the exclude filters. Only the name of the dir itself it checked.
- Returns
true
if the file or folder atpath
should be indexed according to the configuration.
Definition at line 165 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::shouldFileBeIndexed | ( | const QString & | fileName | ) | const |
Check fileName
for all exclude filters.
This does not take file paths into account.
- Returns
true
if a file with namefilename
should be indexed according to the configuration.
Definition at line 212 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::shouldFolderBeIndexed | ( | const QString & | path | ) | const |
Check if the folder at path
should be indexed.
Be aware that this method does not check if parent dirs match any of the exclude filters. Only the name of the dir itself it checked.
- Returns
true
if the folder atpath
should be indexed according to the configuration.
Definition at line 179 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::shouldMimeTypeBeIndexed | ( | const QString & | mimeType | ) | const |
Checks if mimeType
should be indexed.
- Returns
true
if the mimetype should be indexed according to the configuration
Definition at line 219 of file fileindexerconfig.cpp.
bool Nepomuk2::FileIndexerConfig::suspendOnPowerSaveDisabled | ( | ) | const |
A "hidden" config option which allows to disable the feature where the file indexing is suspended when in powersave mode.
This is especially useful for mobile devices which always run on battery.
At some point this should be a finer grained configuration.
Definition at line 476 of file fileindexerconfig.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:09 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.