Nepomuk
#include <Nepomuk/Query/ComparisonTerm>
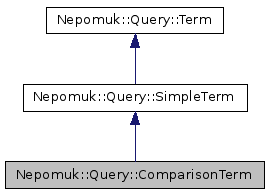
Public Types | |
enum | AggregateFunction { NoAggregateFunction = 0, Count, DistinctCount, Max, Min, Sum, DistinctSum, Average, DistinctAverage } |
enum | Comparator { Contains, Regexp, Equal, Greater, Smaller, GreaterOrEqual, SmallerOrEqual } |
![]() | |
enum | Type { Invalid, Literal, Resource, And, Or, Comparison, ResourceType, Negation, Optional } |
Related Functions | |
(Note that these are not member functions.) | |
Term | operator!= (const Types::Property &property, const Term &term) |
ComparisonTerm | operator< (const Types::Property &property, const Term &term) |
ComparisonTerm | operator<= (const Types::Property &property, const Term &term) |
ComparisonTerm | operator== (const Types::Property &property, const Term &term) |
ComparisonTerm | operator> (const Types::Property &property, const Term &term) |
ComparisonTerm | operator>= (const Types::Property &property, const Term &term) |
![]() | |
static Term | fromProperty (const Types::Property &property, const Variant &variant) |
Additional Inherited Members | |
![]() | |
static Term | fromString (const QString &s) |
static Term | fromVariant (const Variant &variant) |
Detailed Description
A term matching the value of a property.
The ComparisonTerm is the most important term in the query API. It can be used to match the values of properties. As such its core components are a property(), a comparator() (see Comparator for details) and a subTerm(). The latter can be any other term including AndTerm, OrTerm, or even an invalid term. The matching is done as follows:
- A LiteralTerm as subTerm() is directly matched to the value of a literal property (see also Nepomuk::Types::Property::literalRangeType()) or to the labels of related resources in case of a property that has a resource range.
- An invalid sub term simply matches any resource or value, effectively acting as a wildcard.
- Any other term type will be used as a sort of subquery to match the related resources. This means that the property() needs to have a resource range.
In addition to these basic features ComparisonTerm has a few tricks up its sleeve:
- By forcing the variable name via setVariableName() it is possible to include a value matched by the term in the resulting SPARQL query (Query::toSparqlQuery()) or accessing it via Result::additionalBinding().
- It is possible to set an aggregate function via setAggregateFunction() to count or sum up the results instead of returning the actual values.
- Using setSortWeight() the sorting of the results in the final query can be influenced in a powerful way - especially when combined with setAggregateFunction().
- Since
- 4.4
Definition at line 70 of file comparisonterm.h.
Member Enumeration Documentation
Aggregate functions which can be applied to a comparison term to influence the value they return.
- See also
- setAggregateFunction()
- Since
- 4.5
Enumerator | |
---|---|
NoAggregateFunction |
Do not use any aggregate function. |
Count |
Count the number of matching results instead of returning the results themselves. For counting the results of a complete query use Query::CreateCountQuery. |
DistinctCount |
The same as Count except that no two similar results are counted twice. |
Max |
Return the maximum value of all results instead of the results themselves. Does only make sense for numerical values. |
Min |
Return the minimum value of all results instead of the results themselves. Does only make sense for numerical values. |
Sum |
Return the sum of all result values instead of the results themselves. Does only make sense for numerical values. |
DistinctSum |
The same as Sum except that no two similar results are added twice. |
Average |
Return the average value of all results instead of the results themselves. Does only make sense for numerical values. |
DistinctAverage |
The same as Average except that no two similar results are counted twice. |
Definition at line 125 of file comparisonterm.h.
ComparisonTerm supports different ways to compare values.
Enumerator | |
---|---|
Contains |
A LiteralTerm sub-term is matched against string literal values. Case is ignored. The literal value can contain wildcards like *. |
Regexp |
A LiteralTerm sub-term is matched against a string literal value using the literal term's value as a regular expression. It is highly recommended to prefer Contains over Regexp as the latter will result in much slower SPARQL REGEX filters. |
Equal |
A sub-term is matched one-to-one. Sub-terms can be any other term type. |
Greater |
A LiteralTerm sub-term is matched to greater literal values. |
Smaller |
A LiteralTerm sub-term is matched to smaller literal values. |
GreaterOrEqual |
A LiteralTerm sub-term is matched to greater or equal literal values. |
SmallerOrEqual |
A LiteralTerm sub-term is matched to smaller or equal literal values. |
Definition at line 76 of file comparisonterm.h.
Constructor & Destructor Documentation
Nepomuk::Query::ComparisonTerm::ComparisonTerm | ( | ) |
Default constructor: creates a comparison term that matches all properties.
Nepomuk::Query::ComparisonTerm::ComparisonTerm | ( | const ComparisonTerm & | term | ) |
Copy constructor.
Nepomuk::Query::ComparisonTerm::ComparisonTerm | ( | const Types::Property & | property, |
const Term & | term, | ||
Comparator | comparator = Contains |
||
) |
Convinience constructor which covers most simple use cases.
- Parameters
-
property The property that should be matched. An invalid property will act as a wildcard. term The sub term to match to. comparator The Comparator to use for comparison. Not all Comparators make sense with all sub term types.
Nepomuk::Query::ComparisonTerm::~ComparisonTerm | ( | ) |
Destructor.
Member Function Documentation
AggregateFunction Nepomuk::Query::ComparisonTerm::aggregateFunction | ( | ) | const |
The aggregate function to be used with the additional binding set in setVariableName().
- See also
- setAggregateFunction()
- Since
- 4.5
Comparator Nepomuk::Query::ComparisonTerm::comparator | ( | ) | const |
The Comparator used by ComparisonTerm Terms.
- See also
- setComparator
ComparisonTerm Nepomuk::Query::ComparisonTerm::inverted | ( | ) | const |
Create an inverted copy of this ComparisonTerm.
This is a convenience method to allow inline creation of inverted comparison terms when creating queries in a single line of code.
Be aware that calling this method twice wil result in a non-inverted comparison term:
- See also
- setInverted()
- Since
- 4.5
bool Nepomuk::Query::ComparisonTerm::isInverted | ( | ) | const |
ComparisonTerm& Nepomuk::Query::ComparisonTerm::operator= | ( | const ComparisonTerm & | term | ) |
Assignment operator.
Types::Property Nepomuk::Query::ComparisonTerm::property | ( | ) | const |
A property used for ComparisonTerm Terms.
An invalid property will act as a wildcard.
- See also
- setProperty
void Nepomuk::Query::ComparisonTerm::setAggregateFunction | ( | AggregateFunction | function | ) |
Set an aggregate function which changes the result.
The typical usage is to count the results instead of actually returning them. For counting the results of a complete query use Query::CreateCountQuery.
- See also
- aggregateFunction()
- Since
- 4.5
void Nepomuk::Query::ComparisonTerm::setComparator | ( | Comparator | ) |
Set the comparator.
void Nepomuk::Query::ComparisonTerm::setInverted | ( | bool | invert | ) |
Invert the comparison, i.e.
make the subterm the subject of the term and match to objects of the term.
A typical example would be:
which would yield a query like the following:
to get all tags attached to a file.
Be aware that this does only make sense with sub terms that match to resources. When using LiteralTerm as a sub term invert
is ignored.
- See also
- inverted()
- Since
- 4.5
void Nepomuk::Query::ComparisonTerm::setProperty | ( | const Types::Property & | ) |
Set the property for ComparisonTerm Terms.
An invalid property will act as a wildcard.
- See also
- property
void Nepomuk::Query::ComparisonTerm::setSortWeight | ( | int | weight, |
Qt::SortOrder | sortOrder = Qt::AscendingOrder |
||
) |
Set the sort weight of this property.
By default all ComparisonTerms have a weight of 0 which means that they are ignored for sorting. By setting weight
to a value different from 0 (typically higher than 0) the comparison subterm will be used for sorting.
Be aware that as with the variableName() sorting does not apply to sub terms of types ResourceTerm or LiteralTerm. In those cases the value will be ignored. The only exception are LiteralTerm sub terms that are compared other than with equals.
- Parameters
-
weight The new sort weight. If different from 0 this term will be used for sorting in the Query. sortOrder The sort order to use for this term.
- See also
- sortWeight()
- Since
- 4.5
void Nepomuk::Query::ComparisonTerm::setVariableName | ( | const QString & | name | ) |
Set the variable name that is to be used for the variable to match to.
The variable will then be added to the set of variables returned in the results and can be read via Result::additionalBinding(). Setting the variable name can be seen as a less restricted variant of Query::addRequestProperty().
When manually setting the variable name on more than one ComparisonTerm there is no guarantee for the uniqueness of variable names anymore which can result in unwanted query results. However, this can also be used deliberately in case one needs to compare the same variable twice:
The above example would result in a SPARQL query pattern along the lines of
Be aware that the variable name does not apply to sub terms of types ResourceTerm or LiteralTerm. In those cases the value will be ignored. The only exception are LiteralTerm sub terms that are compared other than with equals.
- Parameters
-
name The name of the variable to be used when requesting the binding via Result::additionalBinding()
- Since
- 4.5
Qt::SortOrder Nepomuk::Query::ComparisonTerm::sortOrder | ( | ) | const |
- Returns
- The sort order as set in setSortWeight().
- Since
- 4.5
int Nepomuk::Query::ComparisonTerm::sortWeight | ( | ) | const |
- Returns
- The sort weight as set in setSortWeight() or 0 if sorting is disabled for this term.
- Since
- 4.5
QString Nepomuk::Query::ComparisonTerm::variableName | ( | ) | const |
The variable name set in setVariableName() or an empty string if none has been set.
- See also
- setVariableName()
- Since
- 4.5
Friends And Related Function Documentation
|
related |
Comparision operator for simple creation of negated ComparisionTerm objects using the ComparisonTerm::Equal comparator.
- Since
- 4.6
- Returns
- A Term equvalent to:
- See also
- NegationTerm
|
related |
Comparision operator for simple creation of ComparisonTerm objects using the ComparisonTerm::Smaller comparator.
- Returns
- A ComparisonTerm equvalent to:
- Since
- 4.6
|
related |
Comparision operator for simple creation of ComparisonTerm objects using the ComparisonTerm::SmallerOrEqual comparator.
- Returns
- A ComparisonTerm equvalent to:
- Since
- 4.6
|
related |
Comparision operator for simple creation of ComparisonTerm objects using the ComparisonTerm::Equal comparator.
- Returns
- A ComparisonTerm equvalent to:
- Since
- 4.6
|
related |
Comparision operator for simple creation of ComparisonTerm objects using the ComparisonTerm::Greater comparator.
- Returns
- A ComparisonTerm equvalent to:
- Since
- 4.6
|
related |
Comparision operator for simple creation of ComparisonTerm objects using the ComparisonTerm::GreaterOrEqual comparator.
- Returns
- A ComparisonTerm equvalent to:
- Since
- 4.6
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.