Nepomuk
#include <Nepomuk/Query/QueryServiceClient>
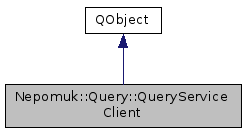
Public Slots | |
bool | blockingDesktopQuery (const QString &query) |
bool | blockingQuery (const Query &query) |
bool | blockingSparqlQuery (const QString &query, const Nepomuk::Query::RequestPropertyMap &requestPropertyMap=Nepomuk::Query::RequestPropertyMap()) |
void | close () |
bool | desktopQuery (const QString &query) |
QString | errorMessage () const |
bool | isListingFinished () const |
bool | query (const Query &query) |
bool | sparqlQuery (const QString &query, const Nepomuk::Query::RequestPropertyMap &requestPropertyMap=Nepomuk::Query::RequestPropertyMap()) |
Signals | |
void | entriesRemoved (const QList< QUrl > &entries) |
void | error (const QString &errorMessage) |
void | finishedListing () |
void | newEntries (const QList< Nepomuk::Query::Result > &entries) |
void | resultCount (int count) |
void | serviceAvailabilityChanged (bool running) |
Public Member Functions | |
QueryServiceClient (QObject *parent=0) | |
~QueryServiceClient () | |
Static Public Member Functions | |
static bool | serviceAvailable () |
static QList < Nepomuk::Query::Result > | syncDesktopQuery (const QString &query, bool *ok=0) |
static QList < Nepomuk::Query::Result > | syncQuery (const Query &query, bool *ok=0) |
static QList < Nepomuk::Query::Result > | syncSparqlQuery (const QString &query, const Nepomuk::Query::RequestPropertyMap &requestPropertyMap=Nepomuk::Query::RequestPropertyMap(), bool *ok=0) |
Detailed Description
Convenience frontend to the Nepomuk Query DBus Service.
The QueryServiceClient provides an easy way to access the Nepomuk Query Service without having to deal with any communication details. By default it monitors queries for changes.
Usage is simple: Create an instance of the client for each search you want to track. One instance may also be reused for subsequent queries if further updates of the persistent query are not necessary.
For quick queries which do not require any updates one of the static query methods can be used: syncQuery(), syncSparqlQuery(), or syncDesktopQuery().
- Since
- 4.4
Definition at line 58 of file queryserviceclient.h.
Constructor & Destructor Documentation
Nepomuk::Query::QueryServiceClient::QueryServiceClient | ( | QObject * | parent = 0 | ) |
Create a new QueryServiceClient instance.
Nepomuk::Query::QueryServiceClient::~QueryServiceClient | ( | ) |
Desctructor.
Closes the query.
Member Function Documentation
|
slot |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a desktop query string which can be parsed by QueryParser.
- See also
- desktopQuery(const QString&), close()
|
slot |
Start a query using the Nepomuk query service.
Results will be reported as with query(const QString&) but a local event loop will be started to block the method call until all results have been listed.
The client will be closed after the initial listing. Thus, changes to results will not be reported as it is the case with the non-blocking methods.
- Parameters
-
query the query to perform.
- Returns
true
if the query service was found and the query was started.false
otherwise.
- See also
- query(const Query&), close()
|
slot |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a SPARQL query which binds results to variable 'r'
.requestPropertyMap An optional mapping of variable binding names in query
to their corresponding properties. For details see sparqlQuery.
- See also
- sparqlQuery(const Query&)
|
slot |
Close the client, thus stop to monitor the query for changes.
Without closing the client it will continue signalling changes to the results.
This will also make any blockingQuery return immediately.
|
slot |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a desktop query string which can be parsed by QueryParser.
|
signal |
Emitted if the search results changed when monitoring a query.
- Parameters
-
entries A list of resource URIs identifying the resources that dropped out of the query results.
|
signal |
Emitted when an error occurs.
This typically happens in case the query service is not running or does not respond. No further signals will be emitted after this one.
- Since
- 4.6
|
slot |
The last error message which has been emitted via error() or an empty string if there was no error.
- Since
- 4.6
|
signal |
Emitted when the initial listing has been finished, ie.
if all results have been reported via newEntries. If no further updates are necessary the client should be closed now.
In case of an error this signal is not emitted.
- See also
- error()
|
slot |
- Returns
true
if all results have been listed (ie. finishedListing() has been emitted), close() has been called, or no query was started.
- Since
- 4.6
|
signal |
Emitted for new search results.
This signal is emitted both for the initial listing and for changes to the search.
|
slot |
Start a query using the Nepomuk query service.
Results will be reported via newEntries. All results have been reported once finishedListing has been emitted.
- Parameters
-
query the query to perform.
- Returns
true
if the query service was found and the query was started.false
otherwise.
|
signal |
The number of results that are reported via newEntries() before the finishedListing() signal.
Emitted once the count of the results is available. This might happen before the first result is emitted, in between the results, or in rare cases it could even happen after all results have been reported.
Also be aware that no count will be provided when using sparqlQuery()
- Since
- 4.6
|
signal |
Emitted when the availability of the query service changes.
- Since
- 4.8
|
static |
|
slot |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a SPARQL query which binds results to variable 'r'
.requestPropertyMap An optional mapping of variable binding names in query
to their corresponding properties. These bindings will then be reported via Result::requestProperties(). This map will be constructed automatically when using query(const Query&).
Examples:
Select a simple request property as can also be done via Query::Query:
This will report the resources themselves and their modification time in the result's request properties:
While using Query::Query restricts to request properties to diret properties of the results using a custom SPARQL query allows to use any binding as request property. The used property URI in the mapping does not even need to match anything in the query:
- See also
- Query::requestPropertyMap()
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a desktop query string which can be parsed by QueryParser. ok a valid boolean pointer, which will be set to true
if the query service was found and the query was started,false
otherwise. If you don't want to track errors, you can pass a null pointer instead.
- See also
- desktopQuery(const QString&)
- Since
- 4.5
|
static |
Start a query using the Nepomuk query service.
A local event loop will be started to block the method call until all results have been listed, and results will be returned. You can check if the query was successful through the ok
pointer.
If updates to the query results are required an instance of QueryServiceClient should be created and one of the non-static query methods be used.
- Parameters
-
query the query to perform. ok a valid boolean pointer, which will be set to true
if the query service was found and the query was started,false
otherwise. If you don't want to track errors, you can pass a null pointer instead.
- Returns
- a list of
Result
for the given query.
- See also
- query(const Query&)
- Since
- 4.5
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
query a SPARQL query which binds results to variable 'r'
.ok a valid boolean pointer, which will be set to true
if the query service was found and the query was started,false
otherwise. If you don't want to track errors, you can pass a null pointer instead.requestPropertyMap An optional mapping of variable binding names in query
to their corresponding properties. For details see sparqlQuery.
- See also
- sparqlQuery(const Query&)
- Since
- 4.5
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.