Plasma
#include <Plasma/Dialog>
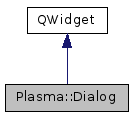
Public Types | |
enum | ResizeCorner { NoCorner = 0, NorthEast = 1, SouthEast = 2, NorthWest = 4, SouthWest = 8, All = NorthEast | SouthEast | NorthWest | SouthWest } |
Public Slots | |
void | syncToGraphicsWidget () |
Signals | |
void | dialogResized () |
void | dialogVisible (bool status) |
Public Member Functions | |
Dialog (QWidget *parent=0, Qt::WindowFlags f=Qt::Window) | |
virtual | ~Dialog () |
void | animatedHide (Plasma::Direction direction) |
void | animatedShow (Plasma::Direction direction) |
Plasma::AspectRatioMode | aspectRatioMode () const |
void | getMinimumResizeLimits (int *left, int *top, int *right, int *bottom) |
QGraphicsWidget * | graphicsWidget () |
bool | isUserResizing () const |
ResizeCorners | resizeCorners () const |
void | setAspectRatioMode (Plasma::AspectRatioMode mode) |
void | setGraphicsWidget (QGraphicsWidget *widget) |
void | setMinimumResizeLimits (int left, int top, int right, int bottom) |
void | setResizeHandleCorners (ResizeCorners corners) |
Protected Member Functions | |
bool | event (QEvent *event) |
bool | eventFilter (QObject *watched, QEvent *event) |
void | focusInEvent (QFocusEvent *event) |
void | hideEvent (QHideEvent *event) |
bool | inControlArea (const QPoint &point) |
void | keyPressEvent (QKeyEvent *event) |
void | mouseMoveEvent (QMouseEvent *event) |
void | mousePressEvent (QMouseEvent *event) |
void | mouseReleaseEvent (QMouseEvent *event) |
void | moveEvent (QMoveEvent *event) |
void | paintEvent (QPaintEvent *e) |
void | resizeEvent (QResizeEvent *e) |
void | showEvent (QShowEvent *event) |
Detailed Description
A dialog that uses the Plasma style.
Dialog provides a dialog-like widget that can be used to display additional information.
Dialog uses the plasma theme, and usually has no window decoration. It's meant as an interim solution to display widgets as extension to plasma applets, for example when you click on an applet like the devicenotifier or the clock, the widget that is then displayed, is a Dialog.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
- Parameters
-
parent the parent widget, for plasmoids, this is usually 0. f the Qt::WindowFlags, default is to not show a windowborder.
Definition at line 381 of file dialog.cpp.
|
virtual |
Definition at line 409 of file dialog.cpp.
Member Function Documentation
void Plasma::Dialog::animatedHide | ( | Plasma::Direction | direction | ) |
Causes an animated hide; requires compositing to work, otherwise the dialog will simply hide.
- Since
- 4.3
Definition at line 796 of file dialog.cpp.
void Plasma::Dialog::animatedShow | ( | Plasma::Direction | direction | ) |
Causes an animated show; requires compositing to work, otherwise the dialog will simply show.
- Since
- 4.3
Definition at line 829 of file dialog.cpp.
Plasma::AspectRatioMode Plasma::Dialog::aspectRatioMode | ( | ) | const |
- Returns
- the preferred aspect ratio mode for placement and resizing
- Since
- 4.4
Definition at line 872 of file dialog.cpp.
|
signal |
Fires when the dialog automatically resizes.
|
signal |
Emit a signal when the dialog become visible/invisible.
|
protected |
Definition at line 551 of file dialog.cpp.
|
protected |
Definition at line 673 of file dialog.cpp.
|
protected |
Definition at line 730 of file dialog.cpp.
void Plasma::Dialog::getMinimumResizeLimits | ( | int * | left, |
int * | top, | ||
int * | right, | ||
int * | bottom | ||
) |
Retrives the minimum resize limits for the dialog.
- Parameters
-
left the screen coordinate that the left may not go beyond; -1 for no limit top the screen coordinate that the top may not go beyond; -1 for no limit right the screen coordinate that the right may not go beyond; -1 for no limit bottom the screen coordinate that the bottom may not go beyond; -1 for no limit
Definition at line 777 of file dialog.cpp.
QGraphicsWidget * Plasma::Dialog::graphicsWidget | ( | ) |
- Returns
- the graphics widget shown in this dialog
Definition at line 668 of file dialog.cpp.
|
protected |
Definition at line 692 of file dialog.cpp.
|
protected |
Convenience method to know whether the point is in a control area (e.g.
resize area) or not.
- Returns
- true if the point is in the control area.
Definition at line 862 of file dialog.cpp.
bool Plasma::Dialog::isUserResizing | ( | ) | const |
- Returns
- true if currently being resized by the user
Definition at line 764 of file dialog.cpp.
|
protected |
Definition at line 544 of file dialog.cpp.
|
protected |
Definition at line 422 of file dialog.cpp.
|
protected |
Definition at line 516 of file dialog.cpp.
|
protected |
Definition at line 533 of file dialog.cpp.
|
protected |
Definition at line 744 of file dialog.cpp.
|
protected |
Reimplemented from QWidget.
Definition at line 415 of file dialog.cpp.
Dialog::ResizeCorners Plasma::Dialog::resizeCorners | ( | ) | const |
Convenience method to get the enabled resize corners.
- Returns
- which resize corners are active.
Definition at line 759 of file dialog.cpp.
|
protected |
Definition at line 556 of file dialog.cpp.
void Plasma::Dialog::setAspectRatioMode | ( | Plasma::AspectRatioMode | mode | ) |
Sets the preferred aspect ratio mode for placement and resizing.
- Since
- 4.4
Definition at line 877 of file dialog.cpp.
void Plasma::Dialog::setGraphicsWidget | ( | QGraphicsWidget * | widget | ) |
Sets a QGraphicsWidget to be shown as the content in this dialog.
The dialog will then set up a QGraphicsView and coordinate geometry with the widget automatically.
- Parameters
-
widget the QGraphicsWidget to display in this dialog
Definition at line 614 of file dialog.cpp.
void Plasma::Dialog::setMinimumResizeLimits | ( | int | left, |
int | top, | ||
int | right, | ||
int | bottom | ||
) |
Sets the minimum values that each of four sides of the rect may expand to or from.
- Parameters
-
left the screen coordinate that the left may not go beyond; -1 for no limit top the screen coordinate that the top may not go beyond; -1 for no limit right the screen coordinate that the right may not go beyond; -1 for no limit bottom the screen coordinate that the bottom may not go beyond; -1 for no limit
Definition at line 769 of file dialog.cpp.
void Plasma::Dialog::setResizeHandleCorners | ( | ResizeCorners | corners | ) |
- Parameters
-
corners the corners the resize handlers should be placed in.
Definition at line 751 of file dialog.cpp.
|
protected |
Definition at line 698 of file dialog.cpp.
|
slot |
Adjusts the dialog to the associated QGraphicsWidget's geometry Should not normally need to be called by users of Dialog as Dialog does it automatically.
Event compression may cause unwanted delays, however, and so this method may be called to immediately cause a synchronization.
- Since
- 4.5
Definition at line 283 of file dialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.