Plasma
#include <Plasma/PackageStructure>
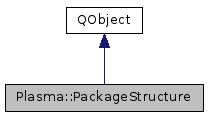
Public Types | |
typedef KSharedPtr < PackageStructure > | Ptr |
Signals | |
void | newWidgetBrowserFinished () |
Public Member Functions | |
PackageStructure (QObject *parent=0, const QString &type=i18nc("A non-functional package","Invalid")) | |
virtual | ~PackageStructure () |
void | addDirectoryDefinition (const char *key, const QString &path, const QString &name) |
void | addFileDefinition (const char *key, const QString &path, const QString &name) |
bool | allowExternalPaths () const |
QString | contentsPrefix () const |
QStringList | contentsPrefixPaths () const |
virtual void | createNewWidgetBrowser (QWidget *parent=0) |
QString | defaultPackageRoot () const |
QList< const char * > | directories () const |
QStringList | entryList (const char *key) |
QList< const char * > | files () const |
virtual bool | installPackage (const QString &archivePath, const QString &packageRoot) |
bool | isRequired (const char *key) const |
virtual PackageMetadata | metadata () |
QStringList | mimetypes (const char *key) const |
QString | name (const char *key) const |
PackageStructure & | operator= (const PackageStructure &rhs) |
QString | path (const char *key) const |
QString | path () const |
void | read (const KConfigBase *config) |
void | removeDefinition (const char *key) |
QList< const char * > | requiredDirectories () const |
QList< const char * > | requiredFiles () const |
QStringList | searchPath (const char *key) const |
QString | servicePrefix () const |
void | setDefaultMimetypes (QStringList mimetypes) |
void | setMimetypes (const char *key, QStringList mimetypes) |
void | setPath (const QString &path) |
void | setRequired (const char *key, bool required) |
void | setServicePrefix (const QString &servicePrefix) |
QString | type () const |
virtual bool | uninstallPackage (const QString &packageName, const QString &packageRoot) |
void | write (KConfigBase *config) const |
Static Public Member Functions | |
static PackageStructure::Ptr | load (const QString &packageFormat) |
Protected Member Functions | |
virtual void | pathChanged () |
void | setAllowExternalPaths (bool allow) |
void | setContentsPrefix (const QString &prefix) |
void | setContentsPrefixPaths (const QStringList &prefixPaths) |
void | setDefaultPackageRoot (const QString &packageRoot) |
Detailed Description
A description of the expected file structure of a given package type.
PackageStructure defines what is in a package. This information is used to create packages and provides a way to programatically refer to contents.
An example usage of this class might be:
One may also choose to create a subclass of PackageStructure and include the setup in the constructor.
Either way, PackageStructure creates a sort of "contract" between the packager and the application which is also self-documenting.
Definition at line 72 of file packagestructure.h.
Member Typedef Documentation
typedef KSharedPtr<PackageStructure> Plasma::PackageStructure::Ptr |
Definition at line 77 of file packagestructure.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor for a package structure definition.
- Parameters
-
type the type of package. This is often application specific.
Definition at line 107 of file packagestructure.cpp.
|
virtual |
Destructor.
Definition at line 113 of file packagestructure.cpp.
Member Function Documentation
void Plasma::PackageStructure::addDirectoryDefinition | ( | const char * | key, |
const QString & | path, | ||
const QString & | name | ||
) |
Adds a directory to the structure of the package.
It is added as a not-required element with no associated mimetypes.
Starting in 4.6, if an entry with the given key already exists, the path is added to it as a search alternative.
- Parameters
-
key used as an internal label for this directory path the path within the package for this directory name the user visible (translated) name for the directory
Definition at line 311 of file packagestructure.cpp.
void Plasma::PackageStructure::addFileDefinition | ( | const char * | key, |
const QString & | path, | ||
const QString & | name | ||
) |
Adds a file to the structure of the package.
It is added as a not-required element with no associated mimetypes.
Starting in 4.6, if an entry with the given key already exists, the path is added to it as a search alternative.
- Parameters
-
key used as an internal label for this file path the path within the package for this file name the user visible (translated) name for the file
Definition at line 330 of file packagestructure.cpp.
bool Plasma::PackageStructure::allowExternalPaths | ( | ) | const |
- Returns
- true if paths/symlinks outside the package itself should be followed. By default this is set to false for security reasons.
Definition at line 657 of file packagestructure.cpp.
QString Plasma::PackageStructure::contentsPrefix | ( | ) | const |
- Returns
- the prefix inserted between the base path and content entries
- Deprecated:
- use contentsPrefixPaths() instead.
Definition at line 533 of file packagestructure.cpp.
QStringList Plasma::PackageStructure::contentsPrefixPaths | ( | ) | const |
- Returns
- the prefix paths inserted between the base path and content entries, in order of priority. When searching for a file, all paths will be tried in order.
- Since
- 4.6
Definition at line 544 of file packagestructure.cpp.
|
virtual |
When called, the package plugin should display a window to the user that they can use to browser, select and then install widgets supported by this package plugin with.
The user interface may be an in-process dialog or an out-of-process application.
When the process is complete, the newWidgetBrowserFinished() signal must be emitted.
- Parameters
-
parent the parent widget to use for the widget
Definition at line 575 of file packagestructure.cpp.
QString Plasma::PackageStructure::defaultPackageRoot | ( | ) | const |
- Returns
- preferred package root. This defaults to plasma/plasmoids/
Definition at line 581 of file packagestructure.cpp.
QList< const char * > Plasma::PackageStructure::directories | ( | ) | const |
The directories defined for this package.
Definition at line 219 of file packagestructure.cpp.
QStringList Plasma::PackageStructure::entryList | ( | const char * | key | ) |
Get the list of files of a given type.
- Parameters
-
key the type of file to look for
- Returns
- list of files by name
- Since
- 4.3
Definition at line 272 of file packagestructure.cpp.
QList< const char * > Plasma::PackageStructure::files | ( | ) | const |
The individual files, by key, that are defined for this package.
Definition at line 246 of file packagestructure.cpp.
|
virtual |
Installs a package matching this package structure.
By default installs a native Plasma::Package.
- Parameters
-
archivePath path to the package archive file packageRoot path to the directory where the package should be installed to
- Returns
- true on successful installation, false otherwise
Definition at line 565 of file packagestructure.cpp.
bool Plasma::PackageStructure::isRequired | ( | const char * | key | ) | const |
- Returns
- true if the item at path exists and is required
Definition at line 397 of file packagestructure.cpp.
|
static |
Loads a package format by name.
- Parameters
-
format If not empty, attempts to locate the given format, either from built-ins or via plugins.
- Returns
- a package that matches the format, if available. The caller is responsible for deleting the object.
Definition at line 118 of file packagestructure.cpp.
|
virtual |
- Returns
- the package metadata object.
Definition at line 616 of file packagestructure.cpp.
QStringList Plasma::PackageStructure::mimetypes | ( | const char * | key | ) | const |
- Returns
- the mimetypes associated with the path, if any
Definition at line 422 of file packagestructure.cpp.
QString Plasma::PackageStructure::name | ( | const char * | key | ) | const |
- Returns
- user visible name for the given entry
Definition at line 377 of file packagestructure.cpp.
|
signal |
Emitted when the new widget browser process completes.
PackageStructure & Plasma::PackageStructure::operator= | ( | const PackageStructure & | rhs | ) |
Assignment operator.
Definition at line 204 of file packagestructure.cpp.
QString Plasma::PackageStructure::path | ( | const char * | key | ) | const |
- Returns
- path relative to the package root for the given entry use searchPaths instead
Definition at line 353 of file packagestructure.cpp.
QString Plasma::PackageStructure::path | ( | ) | const |
- Returns
- the path to the package, or QString() if none
Definition at line 464 of file packagestructure.cpp.
|
protectedvirtual |
Called whenever the path changes so that subclasses may take package specific actions.
Definition at line 469 of file packagestructure.cpp.
void Plasma::PackageStructure::read | ( | const KConfigBase * | config | ) |
Read a package structure from a config file.
Definition at line 474 of file packagestructure.cpp.
void Plasma::PackageStructure::removeDefinition | ( | const char * | key | ) |
Removes a definition from the structure of the package.
- Since
- 4.6
- Parameters
-
key the internal label of the file or directory to remove
Definition at line 348 of file packagestructure.cpp.
QList< const char * > Plasma::PackageStructure::requiredDirectories | ( | ) | const |
The required directories defined for this package.
Definition at line 232 of file packagestructure.cpp.
QList< const char * > Plasma::PackageStructure::requiredFiles | ( | ) | const |
The individual required files, by key, that are defined for this package.
Definition at line 259 of file packagestructure.cpp.
QStringList Plasma::PackageStructure::searchPath | ( | const char * | key | ) | const |
- Returns
- a list of paths relative to the package root for the given entry. They are orted by importance: when searching for a file the paths will be searched in order
- Since
- 4.6
Definition at line 365 of file packagestructure.cpp.
QString Plasma::PackageStructure::servicePrefix | ( | ) | const |
- Returns
- service prefix used in desktop files. This defaults to plasma-applet-
Definition at line 586 of file packagestructure.cpp.
|
protected |
Sets whether or not external paths/symlinks can be followed by a package.
- Parameters
-
allow true if paths/symlinks outside of the package should be followed, false if they should be rejected.
Definition at line 662 of file packagestructure.cpp.
|
protected |
Sets the prefix that all the contents in this package should appear under.
This defaults to "contents/" and is added automatically between the base path and the entries as defined by the package structure
- Parameters
-
prefix the directory prefix to use
- Deprecated:
- use setContentsPrefixPaths() instead.
Definition at line 538 of file packagestructure.cpp.
|
protected |
Sets the prefixes that all the contents in this package should appear under.
This defaults to "contents/" and is added automatically between the base path and the entries as defined by the package structure. Multiple entries can be added. In this case each file request will be searched in all prefixes in order, and the first found will be returned.
- Parameters
-
prefix paths the directory prefix to use
- Since
- 4.6
Definition at line 549 of file packagestructure.cpp.
void Plasma::PackageStructure::setDefaultMimetypes | ( | QStringList | mimetypes | ) |
Defines the default mimetypes for any definitions that do not have associated mimetypes.
Handy for packages with only one or predominantly one file type.
- Parameters
-
mimetypes a list of mimetypes
Definition at line 407 of file packagestructure.cpp.
|
protected |
Sets preferred package root.
Definition at line 591 of file packagestructure.cpp.
void Plasma::PackageStructure::setMimetypes | ( | const char * | key, |
QStringList | mimetypes | ||
) |
Define mimetypes for a given part of the structure The path must already have been added using addDirectoryDefinition or addFileDefinition.
- Parameters
-
key the entry within the package mimetypes a list of mimetypes
Definition at line 412 of file packagestructure.cpp.
void Plasma::PackageStructure::setPath | ( | const QString & | path | ) |
Sets the path to the package.
Useful for package formats which do not have well defined contents prior to installation.
Definition at line 436 of file packagestructure.cpp.
void Plasma::PackageStructure::setRequired | ( | const char * | key, |
bool | required | ||
) |
Sets whether or not a given part of the structure is required or not.
The path must already have been added using addDirectoryDefinition or addFileDefinition.
- Parameters
-
key the entry within the package required true if this entry is required, false if not
Definition at line 387 of file packagestructure.cpp.
void Plasma::PackageStructure::setServicePrefix | ( | const QString & | servicePrefix | ) |
Sets service prefix.
Definition at line 596 of file packagestructure.cpp.
QString Plasma::PackageStructure::type | ( | ) | const |
Type of package this structure describes.
Definition at line 214 of file packagestructure.cpp.
|
virtual |
Uninstalls a package matching this package structure.
- Parameters
-
packageName the name of the package to remove packageRoot path to the directory where the package should be installed to
- Returns
- true on successful removal of the package, false otherwise
Definition at line 570 of file packagestructure.cpp.
void Plasma::PackageStructure::write | ( | KConfigBase * | config | ) | const |
Write this package structure to a config file.
Definition at line 506 of file packagestructure.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.