Plasma
#include <Plasma/RunnerContext>
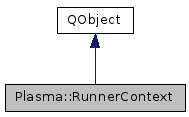
Public Types | |
enum | Type { None = 0, UnknownType = 1, Directory = 2, File = 4, NetworkLocation = 8, Executable = 16, ShellCommand = 32, Help = 64, FileSystem = Directory | File | Executable | ShellCommand } |
Signals | |
void | matchesChanged () |
Public Member Functions | |
RunnerContext (QObject *parent=0) | |
RunnerContext (RunnerContext &other, QObject *parent=0) | |
~RunnerContext () | |
bool | addMatch (const QString &term, const QueryMatch &match) |
bool | addMatches (const QString &term, const QList< QueryMatch > &matches) |
bool | isValid () const |
QueryMatch | match (const QString &id) const |
QList< QueryMatch > | matches () const |
QString | mimeType () const |
RunnerContext & | operator= (const RunnerContext &other) |
QString | query () const |
bool | removeMatch (const QString matchId) |
bool | removeMatches (const QStringList matchIdList) |
bool | removeMatches (AbstractRunner *runner) |
void | reset () |
void | restore (const KConfigGroup &config) |
void | run (const QueryMatch &match) |
void | save (KConfigGroup &config) |
void | setQuery (const QString &term) |
void | setSingleRunnerQueryMode (bool enabled) |
bool | singleRunnerQueryMode () const |
Type | type () const |
Detailed Description
The RunnerContext class provides information related to a search, including the search term, metadata on the search term and collected matches.
Definition at line 46 of file runnercontext.h.
Member Enumeration Documentation
Enumerator | |
---|---|
None | |
UnknownType | |
Directory | |
File | |
NetworkLocation | |
Executable | |
ShellCommand | |
Help | |
FileSystem |
Definition at line 51 of file runnercontext.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 266 of file runnercontext.cpp.
Plasma::RunnerContext::RunnerContext | ( | RunnerContext & | other, |
QObject * | parent = 0 |
||
) |
Copy constructor.
Definition at line 273 of file runnercontext.cpp.
Plasma::RunnerContext::~RunnerContext | ( | ) |
Definition at line 281 of file runnercontext.cpp.
Member Function Documentation
bool Plasma::RunnerContext::addMatch | ( | const QString & | term, |
const QueryMatch & | match | ||
) |
Appends a match to the existing list of matches.
If you are going to be adding multiple matches, use addMatches instead.
- Parameters
-
term the search term that this match was generated for. match the match to add
- Returns
- true if the match was added, false otherwise.
Definition at line 403 of file runnercontext.cpp.
bool Plasma::RunnerContext::addMatches | ( | const QString & | term, |
const QList< QueryMatch > & | matches | ||
) |
Appends lists of matches to the list of matches.
This method is thread safe and causes the matchesChanged() signal to be emitted.
- Returns
- true if matches were added, false if matches were e.g. outdated
Definition at line 368 of file runnercontext.cpp.
bool Plasma::RunnerContext::isValid | ( | ) | const |
- Returns
- true if this context is no longer valid and therefore matching using it should abort. Most useful as an optimization technique inside of AbstractRunner subclasses in the match method, e.g.:
while (.. a possibly large iteration) { if (!context.isValid()) { return; }
... some processing ... }
While not required to be used within runners, it provies a nice way to avoid unnecessary processing in runners that may run for an extended period (as measured in 10s of ms) and therefore improve the user experience.
- Since
- 4.2.3
Definition at line 362 of file runnercontext.cpp.
QueryMatch Plasma::RunnerContext::match | ( | const QString & | id | ) | const |
Retrieves a match by id.
- Parameters
-
id the id of the match to return
- Returns
- the match associated with this id, or an invalid QueryMatch object if the id does not eixst
Definition at line 525 of file runnercontext.cpp.
QList< QueryMatch > Plasma::RunnerContext::matches | ( | ) | const |
Retrieves all available matches for the current search term.
- Returns
- a list of matches
Definition at line 517 of file runnercontext.cpp.
|
signal |
QString Plasma::RunnerContext::mimeType | ( | ) | const |
The mimetype that the search term refers to, if discoverable.
- Returns
- QString() if the mimetype can not be determined, otherwise the mimetype of the object being referred to by the search string.
Definition at line 357 of file runnercontext.cpp.
RunnerContext & Plasma::RunnerContext::operator= | ( | const RunnerContext & | other | ) |
QString Plasma::RunnerContext::query | ( | ) | const |
- Returns
- the current search query term.
Definition at line 344 of file runnercontext.cpp.
bool Plasma::RunnerContext::removeMatch | ( | const QString | matchId | ) |
Removes a match from the existing list of matches.
If you are going to be removing multiple matches, use removeMatches instead.
- Parameters
-
matchId the id of match to remove
- Returns
- true if the match was removed, false otherwise.
- Since
- 4.4
Definition at line 466 of file runnercontext.cpp.
bool Plasma::RunnerContext::removeMatches | ( | const QStringList | matchIdList | ) |
Removes lists of matches from the existing list of matches.
This method is thread safe and causes the matchesChanged() signal to be emitted.
- Parameters
-
matchIdList the list of matches id to remove
- Returns
- true if at least one match was removed, false otherwise.
- Since
- 4.4
Definition at line 429 of file runnercontext.cpp.
bool Plasma::RunnerContext::removeMatches | ( | Plasma::AbstractRunner * | runner | ) |
Removes lists of matches from a given AbstractRunner.
This method is thread safe and causes the matchesChanged() signal to be emitted.
- Parameters
-
runner the AbstractRunner from which to remove matches
- Returns
- true if at least one match was removed, false otherwise.
- Since
- 4.10
Definition at line 486 of file runnercontext.cpp.
void Plasma::RunnerContext::reset | ( | ) |
Resets the search term for this object.
This removes all current matches in the process and turns off single runner query mode.
Definition at line 300 of file runnercontext.cpp.
void Plasma::RunnerContext::restore | ( | const KConfigGroup & | config | ) |
Sets the launch counts for the associated match ids.
If a runner adds a match to this context, the context will check if the match id has been launched before and increase the matches relevance correspondingly. In this manner, any front end can implement adaptive search by sorting items according to relevance.
- Parameters
-
config the config group where launch data was stored
Definition at line 548 of file runnercontext.cpp.
void Plasma::RunnerContext::run | ( | const QueryMatch & | match | ) |
Run a match using the information from this context.
The context will also keep track of the number of times the match was launched to sort future matches according to user habits
- Parameters
-
match the match to run
Definition at line 575 of file runnercontext.cpp.
void Plasma::RunnerContext::save | ( | KConfigGroup & | config | ) |
- Parameters
-
config the config group where launch data should be stored
Definition at line 561 of file runnercontext.cpp.
void Plasma::RunnerContext::setQuery | ( | const QString & | term | ) |
Sets the query term for this object and attempts to determine the type of the search.
Definition at line 332 of file runnercontext.cpp.
void Plasma::RunnerContext::setSingleRunnerQueryMode | ( | bool | enabled | ) |
Sets single runner query mode.
Note that a call to reset() will turn off single runner query mode.
- See also
- reset()
- Since
- 4.4
Definition at line 538 of file runnercontext.cpp.
bool Plasma::RunnerContext::singleRunnerQueryMode | ( | ) | const |
- Returns
- true if the current query is a single runner query
- Since
- 4.4
Definition at line 543 of file runnercontext.cpp.
RunnerContext::Type Plasma::RunnerContext::type | ( | ) | const |
The type of item the search term might refer to.
- See also
- Type
Definition at line 352 of file runnercontext.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.