Plasma
#include <scrollwidget.h>
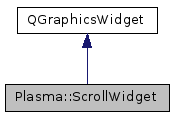
Signals | |
void | scrollStateChanged (QAbstractAnimation::State newState, QAbstractAnimation::State oldState) |
void | viewportGeometryChanged (const QRectF &geomety) |
Public Member Functions | |
ScrollWidget (QGraphicsWidget *parent=0) | |
ScrollWidget (QGraphicsItem *parent) | |
~ScrollWidget () | |
Qt::Alignment | alignment () const |
QSizeF | contentsSize () const |
Q_INVOKABLE void | ensureItemVisible (QGraphicsItem *item) |
Q_INVOKABLE void | ensureRectVisible (const QRectF &rect) |
bool | hasOverShoot () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QWidget * | nativeWidget () const |
bool | overflowBordersVisible () const |
Q_INVOKABLE void | registerAsDragHandle (QGraphicsWidget *item) |
QPointF | scrollPosition () const |
void | setAlignment (Qt::Alignment align) |
void | setHorizontalScrollBarPolicy (const Qt::ScrollBarPolicy policy) |
void | setOverflowBordersVisible (const bool visible) |
void | setOverShoot (bool enable) |
void | setScrollPosition (const QPointF &position) |
void | setSnapSize (const QSizeF &size) |
void | setStyleSheet (const QString &stylesheet) |
void | setVerticalScrollBarPolicy (const Qt::ScrollBarPolicy policy) |
void | setWidget (QGraphicsWidget *widget) |
QSizeF | snapSize () const |
QString | styleSheet () const |
Q_INVOKABLE void | unregisterAsDragHandle (QGraphicsWidget *item) |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QRectF | viewportGeometry () const |
QGraphicsWidget * | widget () const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) |
void | focusInEvent (QFocusEvent *event) |
void | keyPressEvent (QKeyEvent *event) |
void | mouseMoveEvent (QGraphicsSceneMouseEvent *event) |
void | mousePressEvent (QGraphicsSceneMouseEvent *event) |
void | mouseReleaseEvent (QGraphicsSceneMouseEvent *event) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
bool | sceneEventFilter (QGraphicsItem *i, QEvent *e) |
QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint) const |
void | wheelEvent (QGraphicsSceneWheelEvent *event) |
Properties | |
Qt::Alignment | alignment |
QSizeF | contentsSize |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy |
bool | overflowBordersVisible |
bool | overShoot |
QPointF | scrollPosition |
QSizeF | snapSize |
QString | styleSheet |
Qt::ScrollBarPolicy | verticalScrollBarPolicy |
QRectF | viewportGeometry |
QGraphicsWidget | widget |
Detailed Description
A container of widgets that can have scrollbars.
A container of widgets that can have horizontal and vertical scrollbars if the content is bigger than the widget itself
- Since
- 4.3
Definition at line 43 of file scrollwidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a new ScrollWidget.
- Parameters
-
parent the parent of this widget
Definition at line 1117 of file scrollwidget.cpp.
|
explicit |
Definition at line 1110 of file scrollwidget.cpp.
Plasma::ScrollWidget::~ScrollWidget | ( | ) |
Definition at line 1124 of file scrollwidget.cpp.
Member Function Documentation
Qt::Alignment Plasma::ScrollWidget::alignment | ( | ) | const |
- Returns
- currently set alignment for the inner widget
- Since
- 4.5
QSizeF Plasma::ScrollWidget::contentsSize | ( | ) | const |
- Returns
- the size of the internal widget
- Since
- 4.4
void Plasma::ScrollWidget::ensureItemVisible | ( | QGraphicsItem * | item | ) |
Scroll the view until the given item is visible.
- Parameters
-
item item we want visible
- Since
- 4.4
Definition at line 1209 of file scrollwidget.cpp.
void Plasma::ScrollWidget::ensureRectVisible | ( | const QRectF & | rect | ) |
Scroll the view until the given rectangle is visible.
- Parameters
-
rect rect we want visible, in coordinates mapped to the inner widget
- Since
- 4.4
Definition at line 1199 of file scrollwidget.cpp.
|
protected |
Definition at line 1415 of file scrollwidget.cpp.
|
protected |
Definition at line 1324 of file scrollwidget.cpp.
bool Plasma::ScrollWidget::hasOverShoot | ( | ) | const |
Qt::ScrollBarPolicy Plasma::ScrollWidget::horizontalScrollBarPolicy | ( | ) | const |
- Returns
- the horizontal scrollbar policy
|
protected |
Definition at line 1358 of file scrollwidget.cpp.
|
protected |
Definition at line 1363 of file scrollwidget.cpp.
|
protected |
Definition at line 1375 of file scrollwidget.cpp.
|
protected |
Definition at line 1393 of file scrollwidget.cpp.
QWidget * Plasma::ScrollWidget::nativeWidget | ( | ) | const |
- Returns
- the native widget wrapped by this ScrollWidget
Definition at line 1319 of file scrollwidget.cpp.
bool Plasma::ScrollWidget::overflowBordersVisible | ( | ) | const |
- Returns
- true if the widget shows borders when the inner widget is bigger than the viewport
- Since
- 4.6
void Plasma::ScrollWidget::registerAsDragHandle | ( | QGraphicsWidget * | item | ) |
Register an item as a drag handle, it means mouse events will pass trough it and will be possible to drag the view by dragging the item itself.
The item will still receive mouse clicks if the mouse didn't move between press and release.
This function is no more necessary, since it's the authomatic behaviour for all children items, the implementation has now no effect
- Parameters
-
item the drag handle item. widget() must be an ancestor if it in the parent hierarchy. if item doesn't accept mose press events it's not necessary to call this function.
- Since
- 4.4
Definition at line 1237 of file scrollwidget.cpp.
|
protected |
Definition at line 1334 of file scrollwidget.cpp.
|
protected |
Definition at line 1478 of file scrollwidget.cpp.
QPointF Plasma::ScrollWidget::scrollPosition | ( | ) | const |
- Returns
- the position of the internal widget relative to this widget
- Since
- 4.4
|
signal |
The widget started or stopped an animated scroll.
- Since
- 4.4
void Plasma::ScrollWidget::setAlignment | ( | Qt::Alignment | align | ) |
Sets the alignment for the inner widget.
It is only meaningful if the inner widget is smaller than the viewport.
- Since
- 4.5
Definition at line 1576 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setHorizontalScrollBarPolicy | ( | const Qt::ScrollBarPolicy | policy | ) |
Sets the horizontal scrollbar policy.
- Parameters
-
policy desired policy
Definition at line 1162 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setOverflowBordersVisible | ( | const bool | visible | ) |
Sets whether borders should be shown when the inner widget is bigger than the viewport.
- Parameters
-
visible true if the border should be visible when the inner widget overflows
- Since
- 4.6
Definition at line 1189 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setOverShoot | ( | bool | enable | ) |
Tells the scrollwidget whether the widget can scroll a little beyond its boundaries and then automatically snap back or whether the widget scrolling always stops at the edges.
- Since
- 4.5
Definition at line 1591 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setScrollPosition | ( | const QPointF & | position | ) |
Sets the position of the internal widget relative to this widget.
- Since
- 4.4
Definition at line 1274 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setSnapSize | ( | const QSizeF & | size | ) |
Set the nap size of the kinetic scrolling: the scrolling will always stop at multiples of that size.
- Parameters
-
the desired snap size
- Since
- 4.5
Definition at line 1297 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setStyleSheet | ( | const QString & | stylesheet | ) |
Sets the stylesheet used to control the visual display of this ScrollWidget.
- Parameters
-
stylesheet a CSS string
Definition at line 1307 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setVerticalScrollBarPolicy | ( | const Qt::ScrollBarPolicy | policy | ) |
Sets the vertical scrollbar policy.
- Parameters
-
policy desired policy
Definition at line 1174 of file scrollwidget.cpp.
void Plasma::ScrollWidget::setWidget | ( | QGraphicsWidget * | widget | ) |
Sets the widget this ScrollWidget will contain ownership is transferred to this scrollwidget, if an old one was already in, it will be deleted.
If the widget size policy allows for horizontal and/or vertical expansion, it will be resized when possible, otherwise it will be kept to whichever width the widget resizes itself.
- Parameters
-
widget the new main sub widget
Definition at line 1129 of file scrollwidget.cpp.
|
protected |
Definition at line 1457 of file scrollwidget.cpp.
QSizeF Plasma::ScrollWidget::snapSize | ( | ) | const |
- Returns
- the snap size of the kinetic scrolling
- Since
- 4.5
QString Plasma::ScrollWidget::styleSheet | ( | ) | const |
- Returns
- the stylesheet currently used with this widget
void Plasma::ScrollWidget::unregisterAsDragHandle | ( | QGraphicsWidget * | item | ) |
Unregister the given item as drag handle (if it was registered)
This function is no more necessary, since it's the authomatic behaviour for all children items, the implementation has now no effect
- Since
- 4.4
Definition at line 1245 of file scrollwidget.cpp.
Qt::ScrollBarPolicy Plasma::ScrollWidget::verticalScrollBarPolicy | ( | ) | const |
- Returns
- the vertical scrollbar policy
QRectF Plasma::ScrollWidget::viewportGeometry | ( | ) | const |
The geometry of the viewport.
- Since
- 4.4
|
signal |
The viewport geomety changed, for instance due a widget resize.
- Since
- 4.5
|
protected |
Definition at line 1403 of file scrollwidget.cpp.
QGraphicsWidget* Plasma::ScrollWidget::widget | ( | ) | const |
- Returns
- the main widget
Property Documentation
|
readwrite |
Definition at line 55 of file scrollwidget.h.
|
read |
Definition at line 51 of file scrollwidget.h.
|
readwrite |
Definition at line 47 of file scrollwidget.h.
|
readwrite |
Definition at line 49 of file scrollwidget.h.
|
readwrite |
Definition at line 56 of file scrollwidget.h.
|
readwrite |
Definition at line 50 of file scrollwidget.h.
|
readwrite |
Definition at line 53 of file scrollwidget.h.
|
readwrite |
Definition at line 54 of file scrollwidget.h.
|
readwrite |
Definition at line 48 of file scrollwidget.h.
|
read |
Definition at line 52 of file scrollwidget.h.
|
readwrite |
Definition at line 46 of file scrollwidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.