Plasma
#include <Plasma/Service>
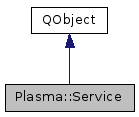
Signals | |
void | finished (Plasma::ServiceJob *job) |
void | operationsChanged () |
void | serviceReady (Plasma::Service *service) |
Public Member Functions | |
~Service () | |
Q_INVOKABLE void | associateItem (QGraphicsObject *item, const QString &operation) |
Q_INVOKABLE void | associateWidget (QWidget *widget, const QString &operation) |
Q_INVOKABLE void | associateWidget (QGraphicsWidget *widget, const QString &operation) |
Q_INVOKABLE QString | destination () const |
Q_INVOKABLE void | disassociateItem (QGraphicsObject *widget) |
Q_INVOKABLE void | disassociateWidget (QWidget *widget) |
Q_INVOKABLE void | disassociateWidget (QGraphicsWidget *widget) |
Q_INVOKABLE bool | isOperationEnabled (const QString &operation) const |
Q_INVOKABLE QString | name () const |
Q_INVOKABLE KConfigGroup | operationDescription (const QString &operationName) |
Q_INVOKABLE QStringList | operationNames () const |
Q_INVOKABLE QMap< QString, QVariant > | parametersFromDescription (const KConfigGroup &description) |
Q_INVOKABLE void | setDestination (const QString &destination) |
Q_INVOKABLE ServiceJob * | startOperationCall (const KConfigGroup &description, QObject *parent=0) |
Static Public Member Functions | |
static Service * | access (const KUrl &url, QObject *parent=0) |
static Service * | load (const QString &name, const QVariantList &args, QObject *parent=0) |
static Service * | load (const QString &name, QObject *parent=0) |
Protected Member Functions | |
Service (QObject *parent=0) | |
Service (QObject *parent, const QVariantList &args) | |
virtual ServiceJob * | createJob (const QString &operation, QMap< QString, QVariant > ¶meters)=0 |
virtual void | registerOperationsScheme () |
void | setName (const QString &name) |
void | setOperationEnabled (const QString &operation, bool enable) |
void | setOperationsScheme (QIODevice *xml) |
Properties | |
QString | destination |
QString | name |
QStringList | operationNames |
Detailed Description
This class provides a generic API for write access to settings or services.
Plasma::Service allows interaction with a "destination", the definition of which depends on the Service itself. For a network settings Service this might be a profile name ("Home", "Office", "Road Warrior") while a web based Service this might be a username ("aseigo", "stranger65").
A Service provides one or more operations, each of which provides some sort of interaction with the destination. Operations are described using config XML which is used to create a KConfig object with one group per operation. The group names are used as the operation names, and the defined items in the group are the parameters available to be set when using that operation.
A service is started with a KConfigGroup (representing a ready to be serviced operation) and automatically deletes itself after completion and signaling success or failure. See KJob for more information on this part of the process.
Services may either be loaded "stand alone" from plugins, or from a DataEngine by passing in a source name to be used as the destination.
Sample use might look like:
Please remember, the service needs to be deleted when it will no longer be used. This can be done manually or by these (perhaps easier) alternatives:
If it is needed throughout the lifetime of the object:
If the service will not be used after just one operation call, use:
Constructor & Destructor Documentation
Plasma::Service::~Service | ( | ) |
Destructor.
Definition at line 63 of file service.cpp.
|
explicitprotected |
Default constructor.
- Parameters
-
parent the parent object for this service
Definition at line 50 of file service.cpp.
|
protected |
Constructor for plugin loading.
Definition at line 56 of file service.cpp.
Member Function Documentation
Used to access a service from an url.
Always check for the signal serviceReady() that fires when this service is actually ready for use.
Definition at line 80 of file service.cpp.
void Plasma::Service::associateItem | ( | QGraphicsObject * | item, |
const QString & | operation | ||
) |
Associates a graphics item with an operation, which allows the service to automatically manage, for example, the enabled state of the item.
This will remove any previous associations the item had with operations on this engine.
- Parameters
-
item the QGraphicsObject to associate with the service operation the operation to associate the item with
Definition at line 288 of file service.cpp.
void Plasma::Service::associateWidget | ( | QWidget * | widget, |
const QString & | operation | ||
) |
Assoicates a widget with an operation, which allows the service to automatically manage, for example, the enabled state of a widget.
This will remove any previous associations the widget had with operations on this engine.
- Parameters
-
widget the QWidget to associate with the service operation the operation to associate the widget with
Definition at line 254 of file service.cpp.
void Plasma::Service::associateWidget | ( | QGraphicsWidget * | widget, |
const QString & | operation | ||
) |
This method only exists to maintain binary compatibility.
- See also
- associateItem
Definition at line 278 of file service.cpp.
|
protectedpure virtual |
Called when a job should be created by the Service.
- Parameters
-
operation which operation to work on parameters the parameters set by the user for the operation
- Returns
- a ServiceJob that can be started and monitored by the consumer
Q_INVOKABLE QString Plasma::Service::destination | ( | ) | const |
- Returns
- the target destination, if any, that this service is associated with
void Plasma::Service::disassociateItem | ( | QGraphicsObject * | widget | ) |
Disassociates a graphics item if it has been associated with an operation on this service.
This will not change the enabled state of the item.
- Parameters
-
widget the QGraphicsItem to disassociate.
Definition at line 302 of file service.cpp.
void Plasma::Service::disassociateWidget | ( | QWidget * | widget | ) |
Disassociates a widget if it has been associated with an operation on this service.
This will not change the enabled state of the widget.
- Parameters
-
widget the QWidget to disassociate.
Definition at line 267 of file service.cpp.
void Plasma::Service::disassociateWidget | ( | QGraphicsWidget * | widget | ) |
This method only exists to maintain binary compatibility.
- See also
- disassociateItem
Definition at line 283 of file service.cpp.
|
signal |
Emitted when a job associated with this Service completes its task.
bool Plasma::Service::isOperationEnabled | ( | const QString & | operation | ) | const |
Query to find if an operation is enabled or not.
- Parameters
-
operation the name of the operation to check
- Returns
- true if the operation is enabled, false otherwise
Definition at line 367 of file service.cpp.
|
static |
Used to load a given service from a plugin.
- Parameters
-
name the plugin name of the service to load args a list of arguments to supply to the service plugin when loading it parent the parent object, if any, for the service
- Returns
- a Service object, guaranteed to be not null.
- Since
- 4.5
Definition at line 75 of file service.cpp.
Used to load a given service from a plugin.
- Parameters
-
name the plugin name of the service to load parent the parent object, if any, for the service
- Returns
- a Service object, guaranteed to be not null.
Definition at line 69 of file service.cpp.
Q_INVOKABLE QString Plasma::Service::name | ( | ) | const |
The name of this service.
KConfigGroup Plasma::Service::operationDescription | ( | const QString & | operationName | ) |
Retrieves the parameters for a given operation.
- Parameters
-
operationName the operation to retrieve parameters for
- Returns
- KConfigGroup containing the parameters
Definition at line 181 of file service.cpp.
Q_INVOKABLE QStringList Plasma::Service::operationNames | ( | ) | const |
- Returns
- the possible operations for this profile
|
signal |
Emitted when the Service's operations change.
For example, a media player service may change what operations are available in response to the state of the player.
QMap< QString, QVariant > Plasma::Service::parametersFromDescription | ( | const KConfigGroup & | description | ) |
- Returns
- a parameter map for the given description
- Parameters
-
description the configuration values to turn into the parameter map
- Since
- 4.4
Definition at line 196 of file service.cpp.
|
protectedvirtual |
By default this is based on the file in plasma/services/name.operations, but can be reimplented to use a different mechanism.
It should result in a call to setOperationsScheme(QIODevice *);
Definition at line 402 of file service.cpp.
|
signal |
Emitted when this service is ready for use.
void Plasma::Service::setDestination | ( | const QString & | destination | ) |
Sets the destination for this Service to operate on.
- Parameters
-
destination specific to each Service, this sets which target or address for ServiceJobs to operate on
Definition at line 161 of file service.cpp.
|
protected |
Sets the name of the Service; useful for Services not loaded from plugins, which use the plugin name for this.
- Parameters
-
name the name to use for this service
Definition at line 318 of file service.cpp.
|
protected |
Enables a given service by name.
- Parameters
-
operation the name of the operation to enable or disable enable true if the operation should be enabld, false if disabled
Definition at line 334 of file service.cpp.
|
protected |
Sets the XML used to define the operation schema for this Service.
Definition at line 372 of file service.cpp.
ServiceJob * Plasma::Service::startOperationCall | ( | const KConfigGroup & | description, |
QObject * | parent = 0 |
||
) |
Called to create a ServiceJob which is associated with a given operation and parameter set.
- Returns
- a started ServiceJob; the consumer may connect to relevant signals before returning to the event loop
Definition at line 215 of file service.cpp.
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.