Plasma
#include <Plasma/Widgets/SignalPlotter>
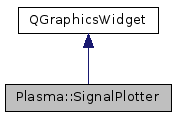
Public Member Functions | |
SignalPlotter (QGraphicsItem *parent=0) | |
~SignalPlotter () | |
Q_INVOKABLE void | addPlot (const QColor &color) |
Q_INVOKABLE void | addSample (const QList< double > &samples) |
QColor | backgroundColor () const |
QFont | font () const |
QColor | fontColor () const |
QPixmap | getSnapshotImage (uint width, uint height) |
QColor | horizontalLinesColor () const |
uint | horizontalLinesCount () const |
uint | horizontalScale () const |
double | lastValue (uint i) const |
QString | lastValueAsString (uint i) const |
QList< PlotColor > & | plotColors () |
Q_INVOKABLE void | removePlot (uint pos) |
Q_INVOKABLE void | reorderPlots (const QList< uint > &newOrder) |
void | scale (qreal delta) |
qreal | scaledBy () const |
void | setBackgroundColor (const QColor &color) |
void | setFont (const QFont &font) |
void | setFontColor (const QColor &color) |
virtual void | setGeometry (const QRectF &geometry) |
void | setHorizontalLinesColor (const QColor &color) |
void | setHorizontalLinesCount (uint count) |
void | setHorizontalScale (uint scale) |
void | setShowHorizontalLines (bool value) |
void | setShowLabels (bool value) |
void | setShowTopBar (bool value) |
void | setShowVerticalLines (bool value) |
void | setStackPlots (bool stack) |
void | setSvgBackground (const QString &filename) |
void | setThinFrame (bool set) |
void | setTitle (const QString &title) |
void | setUnit (const QString &unit) |
void | setUseAutoRange (bool value) |
void | setVerticalLinesColor (const QColor &color) |
void | setVerticalLinesDistance (uint distance) |
void | setVerticalLinesScroll (bool value) |
void | setVerticalRange (double min, double max) |
bool | showHorizontalLines () const |
bool | showLabels () const |
bool | showTopBar () const |
bool | showVerticalLines () const |
bool | stackPlots () const |
QString | svgBackground () |
bool | thinFrame () const |
QString | title () const |
QString | unit () const |
bool | useAutoRange () const |
QColor | verticalLinesColor () const |
uint | verticalLinesDistance () const |
bool | verticalLinesScroll () const |
double | verticalMaxValue () const |
double | verticalMinValue () const |
Protected Member Functions | |
void | calculateNiceRange () |
void | drawAxisText (QPainter *p, int top, int h) |
void | drawBackground (QPainter *p, int w, int h) |
void | drawHorizontalLines (QPainter *p, int top, int w, int h) |
void | drawPlots (QPainter *p, int top, int w, int h, int horizontalScale) |
void | drawThinFrame (QPainter *p, int w, int h) |
void | drawTopBarContents (QPainter *p, int x, int width, int height) |
void | drawTopBarFrame (QPainter *p, int separatorX, int height) |
void | drawVerticalLines (QPainter *p, int top, int w, int h) |
void | drawWidget (QPainter *p, uint w, uint height, int horizontalScale) |
void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
void | updateDataBuffers () |
Properties | |
QColor | backgroundColor |
QFont | font |
QColor | fontColor |
QColor | horizontalLinesColor |
uint | horizontalLinesCount |
uint | horizontalScale |
qreal | scale |
bool | showHorizontalLines |
bool | showLabels |
bool | showTopBar |
bool | showVerticalLines |
bool | stackPlots |
QString | svgBackground |
bool | thinFrame |
QString | title |
QString | unit |
bool | useAutoRange |
QColor | verticalLinesColor |
uint | verticalLinesDistance |
bool | verticalLinesScroll |
Detailed Description
Provides a signal plotter for plasma.
Definition at line 45 of file signalplotter.h.
Constructor & Destructor Documentation
Plasma::SignalPlotter::SignalPlotter | ( | QGraphicsItem * | parent = 0 | ) |
Definition at line 116 of file signalplotter.cpp.
Plasma::SignalPlotter::~SignalPlotter | ( | ) |
Definition at line 155 of file signalplotter.cpp.
Member Function Documentation
void Plasma::SignalPlotter::addPlot | ( | const QColor & | color | ) |
Add a new line to the graph plotter, with the specified color.
Note that the order you add the plots must be the same order that the same data is given in (unless you reorder the plots).
- Parameters
-
color the color to use for this plot
Definition at line 169 of file signalplotter.cpp.
void Plasma::SignalPlotter::addSample | ( | const QList< double > & | samples | ) |
Add data to the graph, and advance the graph by one time period.
The data must be given as a list in the same order that the plots were added (or consequently reordered).
- Parameters
-
samples a list with the new value for each plot
Definition at line 182 of file signalplotter.cpp.
QColor Plasma::SignalPlotter::backgroundColor | ( | ) | const |
The color to set the background.
This might not be seen if an svg is also set.
- Returns
- the color of the plotter background
|
protected |
Definition at line 718 of file signalplotter.cpp.
|
protected |
Definition at line 1038 of file signalplotter.cpp.
|
protected |
Definition at line 699 of file signalplotter.cpp.
|
protected |
Definition at line 1077 of file signalplotter.cpp.
|
protected |
Definition at line 807 of file signalplotter.cpp.
|
protected |
Definition at line 709 of file signalplotter.cpp.
|
protected |
Definition at line 768 of file signalplotter.cpp.
|
protected |
Definition at line 756 of file signalplotter.cpp.
|
protected |
Definition at line 799 of file signalplotter.cpp.
|
protected |
Definition at line 606 of file signalplotter.cpp.
QFont Plasma::SignalPlotter::font | ( | ) | const |
The font used for the axis.
- Returns
- the font used to draw the text of the vertical values
QColor Plasma::SignalPlotter::fontColor | ( | ) | const |
The color of the font used for the axis.
- Returns
- the color used to draw the text of the vertical values
QPixmap Plasma::SignalPlotter::getSnapshotImage | ( | uint | width, |
uint | height | ||
) |
Render the graph to the specified width and height, and return it as an image.
This is useful, for example, if you draw a small version of the graph, but then want to show a large version in a tooltip etc
- Parameters
-
width the width of the snapshot height the height of the snapshot
- Returns
- a snapshot of the plotter as an image
Definition at line 571 of file signalplotter.cpp.
QColor Plasma::SignalPlotter::horizontalLinesColor | ( | ) | const |
The color of the horizontal grid lines.
- Returns
- the color used to draw the horizontal grid lines
uint Plasma::SignalPlotter::horizontalLinesCount | ( | ) | const |
The number of horizontal lines to draw.
Doesn't include the top most and bottom most lines.
- Returns
- the number of horizontal lines that will be drawn
uint Plasma::SignalPlotter::horizontalScale | ( | ) | const |
The number of pixels horizontally between data points.
- Returns
- the number of pixel drawn between two data points
double Plasma::SignalPlotter::lastValue | ( | uint | i | ) | const |
Return the last value that we have for plot i.
Returns 0 if not known.
- Parameters
-
i the plot we like to have the last value from
- Returns
- the last value of this plot or 0 if not found
Definition at line 1087 of file signalplotter.cpp.
QString Plasma::SignalPlotter::lastValueAsString | ( | uint | i | ) | const |
Return a translated string like: "34 %" or "100 KB" for plot i.
- Parameters
-
i the plot we like to have the value as string from
- Returns
- the last value of this plot as a string
Definition at line 1095 of file signalplotter.cpp.
|
protected |
Definition at line 589 of file signalplotter.cpp.
QList< PlotColor > & Plasma::SignalPlotter::plotColors | ( | ) |
Return the list of plot colors, in the order that the plots were added (or later reordered).
- Returns
- a list containing the color of every plot
Definition at line 258 of file signalplotter.cpp.
void Plasma::SignalPlotter::removePlot | ( | uint | pos | ) |
Removes the plot at the specified index.
- Parameters
-
pos the index of the plot to be removed
Definition at line 263 of file signalplotter.cpp.
void Plasma::SignalPlotter::reorderPlots | ( | const QList< uint > & | newOrder | ) |
Reorder the plots into the order given.
For example:
- Parameters
-
newOrder a list with the new position of each plot
Definition at line 222 of file signalplotter.cpp.
void Plasma::SignalPlotter::scale | ( | qreal | delta | ) |
Scale all the values down by the given amount.
This is useful when the data is given in, say, kilobytes, but you set the units as megabytes. Thus you would have to call this with value
set to 1024. This affects all the data already entered.
- Parameters
-
delta the factor used to scale down the values
Definition at line 277 of file signalplotter.cpp.
qreal Plasma::SignalPlotter::scaledBy | ( | ) | const |
Amount scaled down by.
- See also
- scale
- Returns
- the factor used to scale down the values
Definition at line 287 of file signalplotter.cpp.
void Plasma::SignalPlotter::setBackgroundColor | ( | const QColor & | color | ) |
The color to set the background.
This might not be seen if an svg is also set.
- Parameters
-
color the color to use for the plotter background
Definition at line 519 of file signalplotter.cpp.
void Plasma::SignalPlotter::setFont | ( | const QFont & | font | ) |
The font used for the axis.
- Parameters
-
font the font used to draw the text of the vertical values
Definition at line 481 of file signalplotter.cpp.
void Plasma::SignalPlotter::setFontColor | ( | const QColor & | color | ) |
The color of the font used for the axis.
- Parameters
-
color the color used to draw the text of the vertical values
Definition at line 414 of file signalplotter.cpp.
|
virtual |
Overwritten to be notified of size changes.
Needed to update the data buffers that are used to store the samples.
Definition at line 582 of file signalplotter.cpp.
void Plasma::SignalPlotter::setHorizontalLinesColor | ( | const QColor & | color | ) |
The color of the horizontal grid lines.
- Parameters
-
color the color used to draw the horizontal grid lines
Definition at line 424 of file signalplotter.cpp.
void Plasma::SignalPlotter::setHorizontalLinesCount | ( | uint | count | ) |
The number of horizontal lines to draw.
Doesn't include the top most and bottom most lines.
- Parameters
-
count the number of horizontal lines to draw
Definition at line 438 of file signalplotter.cpp.
void Plasma::SignalPlotter::setHorizontalScale | ( | uint | scale | ) |
Set the number of pixels horizontally between data points.
- Parameters
-
scale the number of pixel to draw between two data points
Definition at line 328 of file signalplotter.cpp.
void Plasma::SignalPlotter::setShowHorizontalLines | ( | bool | value | ) |
Whether to draw the horizontal grid lines.
- Parameters
-
value true if the lines should be drawn, otherwise false
Definition at line 400 of file signalplotter.cpp.
void Plasma::SignalPlotter::setShowLabels | ( | bool | value | ) |
Whether to show the vertical axis labels.
- Parameters
-
value true if the values for the vertical axis should get drawn
Definition at line 453 of file signalplotter.cpp.
void Plasma::SignalPlotter::setShowTopBar | ( | bool | value | ) |
Whether to show the title etc at the top.
Even if set, it won't be shown if there isn't room
- Parameters
-
value true if the topbar should be shown
Definition at line 467 of file signalplotter.cpp.
void Plasma::SignalPlotter::setShowVerticalLines | ( | bool | value | ) |
Whether to draw the vertical grid lines.
- Parameters
-
value true if the lines should be drawn, otherwise false
Definition at line 344 of file signalplotter.cpp.
void Plasma::SignalPlotter::setStackPlots | ( | bool | stack | ) |
Whether to stack the plots on top of each other.
The first plot added will be at the bottom. The next plot will be drawn on top, and so on.
- Parameters
-
stack true if the plots should be stacked
Definition at line 547 of file signalplotter.cpp.
void Plasma::SignalPlotter::setSvgBackground | ( | const QString & | filename | ) |
The filename of the svg background.
Set to empty to disable again.
- Parameters
-
filename the SVG file to use as a background image
Definition at line 497 of file signalplotter.cpp.
void Plasma::SignalPlotter::setThinFrame | ( | bool | set | ) |
Whether to show a white line on the left and bottom of the widget, for a 3D effect.
- Parameters
-
set true if the frame should get drawn
Definition at line 533 of file signalplotter.cpp.
void Plasma::SignalPlotter::setTitle | ( | const QString & | title | ) |
Set the title of the graph.
Drawn in the top left.
- Parameters
-
title the title to use in the plotter
Definition at line 292 of file signalplotter.cpp.
void Plasma::SignalPlotter::setUnit | ( | const QString & | unit | ) |
Set the units.
Drawn on the vertical axis of the graph. Must be already translated into the local language.
- Parameters
-
unit the unit string to use
Definition at line 164 of file signalplotter.cpp.
void Plasma::SignalPlotter::setUseAutoRange | ( | bool | value | ) |
Set the minimum and maximum values on the vertical axis automatically from the data available.
- Parameters
-
value true if the plotter should calculate its own min and max values, otherwise false
Definition at line 306 of file signalplotter.cpp.
void Plasma::SignalPlotter::setVerticalLinesColor | ( | const QColor & | color | ) |
The color of the vertical grid lines.
- Parameters
-
color the color used to draw the vertical grid lines
Definition at line 358 of file signalplotter.cpp.
void Plasma::SignalPlotter::setVerticalLinesDistance | ( | uint | distance | ) |
The horizontal distance between the vertical grid lines.
- Parameters
-
distance the distance between two vertical grid lines
Definition at line 372 of file signalplotter.cpp.
void Plasma::SignalPlotter::setVerticalLinesScroll | ( | bool | value | ) |
Whether the vertical lines move with the data.
- Parameters
-
value true if the vertical lines should move with the data
Definition at line 386 of file signalplotter.cpp.
void Plasma::SignalPlotter::setVerticalRange | ( | double | min, |
double | max | ||
) |
Change the minimum and maximum values drawn on the graph.
Note that these values are sanitised. For example, if you set the minimum as 3, and the maximum as 97, then the graph would be drawn between 0 and 100. The algorithm to determine this "nice range" attempts to minimize the number of non-zero digits.
Use setAutoRange instead to determine the range automatically from the data.
- Parameters
-
min the minimum value to use for the vertical axis max the maximum value to use for the vertical axis
Definition at line 251 of file signalplotter.cpp.
bool Plasma::SignalPlotter::showHorizontalLines | ( | ) | const |
Whether to draw the horizontal grid lines.
- Returns
- true if the lines will be drawn, otherwise false
bool Plasma::SignalPlotter::showLabels | ( | ) | const |
Whether to show the vertical axis labels.
- Returns
- true if the values for the vertical axis will get drawn
bool Plasma::SignalPlotter::showTopBar | ( | ) | const |
Whether to show the title etc at the top.
Even if set, it won't be shown if there isn't room
- Returns
- true if the topbar will be shown
bool Plasma::SignalPlotter::showVerticalLines | ( | ) | const |
Whether the vertical grid lines will be drawn.
- Returns
- true if the lines will be drawn, otherwise false
bool Plasma::SignalPlotter::stackPlots | ( | ) | const |
QString Plasma::SignalPlotter::svgBackground | ( | ) |
The filename of the svg background.
Set to empty to disable again.
- Returns
- the file used as a background image
bool Plasma::SignalPlotter::thinFrame | ( | ) | const |
show a white line on the left and bottom of the widget for a 3D effect
- Returns
- true if frame show be draw
- Since
- 4.3
QString Plasma::SignalPlotter::title | ( | ) | const |
Get the title of the graph.
Drawn in the top left.
- Returns
- the title to use in the plotter
QString Plasma::SignalPlotter::unit | ( | ) | const |
Return the units used on the vertical axis of the graph.
- Returns
- the unit string used
|
protected |
Definition at line 558 of file signalplotter.cpp.
bool Plasma::SignalPlotter::useAutoRange | ( | ) | const |
Whether the vertical axis range is set automatically.
- Returns
- true if the plotter calculates its own min and max values, otherwise false
QColor Plasma::SignalPlotter::verticalLinesColor | ( | ) | const |
The color of the vertical grid lines.
- Returns
- the color used to draw the vertical grid lines
uint Plasma::SignalPlotter::verticalLinesDistance | ( | ) | const |
The horizontal distance between the vertical grid lines.
- Returns
- the distance between two vertical grid lines
bool Plasma::SignalPlotter::verticalLinesScroll | ( | ) | const |
Whether the vertical lines move with the data.
- Returns
- true if the vertical lines will move with the data
double Plasma::SignalPlotter::verticalMaxValue | ( | ) | const |
Get the max value of the vertical axis.
- See also
- changeRange
- Returns
- the maximum value to use for the vertical axis
Definition at line 323 of file signalplotter.cpp.
double Plasma::SignalPlotter::verticalMinValue | ( | ) | const |
Get the min value of the vertical axis.
- See also
- changeRange
- Returns
- the minimum value to use for the vertical axis
Definition at line 318 of file signalplotter.cpp.
Property Documentation
|
readwrite |
Definition at line 64 of file signalplotter.h.
|
readwrite |
Definition at line 60 of file signalplotter.h.
|
readwrite |
Definition at line 59 of file signalplotter.h.
|
readwrite |
Definition at line 58 of file signalplotter.h.
|
readwrite |
Definition at line 61 of file signalplotter.h.
|
readwrite |
Definition at line 52 of file signalplotter.h.
|
readwrite |
Definition at line 50 of file signalplotter.h.
|
readwrite |
Definition at line 57 of file signalplotter.h.
|
readwrite |
Definition at line 62 of file signalplotter.h.
|
readwrite |
Definition at line 63 of file signalplotter.h.
|
readwrite |
Definition at line 53 of file signalplotter.h.
|
readwrite |
Definition at line 67 of file signalplotter.h.
|
readwrite |
Definition at line 65 of file signalplotter.h.
|
readwrite |
Definition at line 66 of file signalplotter.h.
|
readwrite |
Definition at line 48 of file signalplotter.h.
|
readwrite |
Definition at line 49 of file signalplotter.h.
|
readwrite |
Definition at line 51 of file signalplotter.h.
|
readwrite |
Definition at line 54 of file signalplotter.h.
|
readwrite |
Definition at line 55 of file signalplotter.h.
|
readwrite |
Definition at line 56 of file signalplotter.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.