Plasma
#include <Plasma/Widgets/TabBar>
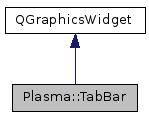
Public Slots | |
void | setCurrentIndex (int index) |
Signals | |
void | currentChanged (int index) |
Public Member Functions | |
TabBar (QGraphicsWidget *parent=0) | |
~TabBar () | |
Q_INVOKABLE int | addTab (const QIcon &icon, const QString &label, QGraphicsLayoutItem *content=0) |
Q_INVOKABLE int | addTab (const QString &label, QGraphicsLayoutItem *content=0) |
int | count () const |
int | currentIndex () const |
QGraphicsWidget * | firstPositionWidget () const |
Q_INVOKABLE int | insertTab (int index, const QIcon &icon, const QString &label, QGraphicsLayoutItem *content=0) |
Q_INVOKABLE int | insertTab (int index, const QString &label, QGraphicsLayoutItem *content=0) |
bool | isTabBarShown () const |
Q_INVOKABLE bool | isTabHighlighted (int index) const |
QGraphicsWidget * | lastPositionWidget () const |
KTabBar * | nativeWidget () const |
Q_INVOKABLE void | removeTab (int index) |
void | setFirstPositionWidget (QGraphicsWidget *widget) |
void | setLastPositionWidget (QGraphicsWidget *widget) |
void | setStyleSheet (const QString &stylesheet) |
void | setTabBarShown (bool show) |
Q_INVOKABLE void | setTabHighlighted (int index, bool highlight) |
Q_INVOKABLE void | setTabIcon (int index, const QIcon &icon) |
Q_INVOKABLE void | setTabText (int index, const QString &label) |
QString | styleSheet () const |
Q_INVOKABLE QGraphicsLayoutItem * | tabAt (int index) |
Q_INVOKABLE QIcon | tabIcon (int index) const |
Q_INVOKABLE QString | tabText (int index) const |
Q_INVOKABLE QGraphicsLayoutItem * | takeTab (int index) |
Protected Member Functions | |
void | changeEvent (QEvent *event) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
void | wheelEvent (QGraphicsSceneWheelEvent *event) |
Properties | |
int | count |
int | currentIndex |
QGraphicsWidget | firstPositionWidget |
QGraphicsWidget | lastPositionWidget |
KTabBar | nativeWidget |
QString | styleSheet |
bool | tabBarShown |
Detailed Description
A tab bar widget, to be used for tabbed interfaces.
Provides a Tab bar for use in a tabbed interface where each page is a QGraphicsLayoutItem. Only one of them is displayed at a given time. It is possible to add and remove tabs or modify their text label or their icon.
Constructor & Destructor Documentation
|
explicit |
Constructs a new TabBar.
- Parameters
-
parent the parent of this widget
Definition at line 221 of file tabbar.cpp.
Plasma::TabBar::~TabBar | ( | ) |
Definition at line 269 of file tabbar.cpp.
Member Function Documentation
int Plasma::TabBar::addTab | ( | const QIcon & | icon, |
const QString & | label, | ||
QGraphicsLayoutItem * | content = 0 |
||
) |
Adds a new tab in the last position.
- Parameters
-
icon the icon for this tab label the text label of the tab content the page content that will be shown by this tab
- Returns
- the index of the inserted tab
Definition at line 322 of file tabbar.cpp.
int Plasma::TabBar::addTab | ( | const QString & | label, |
QGraphicsLayoutItem * | content = 0 |
||
) |
Adds a new tab in the last position This is an overloaded member provided for convenience equivalent to addTab(QIcon(), label, page)
- Parameters
-
label the text label of the tab content the page content that will be shown by this tab
- Returns
- the index of the inserted tab
Definition at line 327 of file tabbar.cpp.
|
protected |
Definition at line 614 of file tabbar.cpp.
int Plasma::TabBar::count | ( | ) | const |
- Returns
- the number of tabs in this tabbar
|
signal |
Emitted when the active tab changes.
- Parameters
-
index the newly activated tab
int Plasma::TabBar::currentIndex | ( | ) | const |
- Returns
- the index of the tab currently active
QGraphicsWidget* Plasma::TabBar::firstPositionWidget | ( | ) | const |
- Returns
- the widget in the first position
- Since
- 4.6
int Plasma::TabBar::insertTab | ( | int | index, |
const QIcon & | icon, | ||
const QString & | label, | ||
QGraphicsLayoutItem * | content = 0 |
||
) |
Adds a new tab in the desired position.
- Parameters
-
index the position where to insert the new tab, if index <=0 will be the first position, if index >= count() will be the last icon the icon for this tab label the text label of the tab content the page content that will be shown by this tab
- Returns
- the index of the inserted tab
Definition at line 275 of file tabbar.cpp.
int Plasma::TabBar::insertTab | ( | int | index, |
const QString & | label, | ||
QGraphicsLayoutItem * | content = 0 |
||
) |
Adds a new tab in the desired position This is an overloaded member provided for convenience equivalent to insertTab(index, QIcon(), label);.
- Parameters
-
index the position where to insert the new tab, if index <=0 will be the first position, if index >= count() will be the last label the text label of the tab content the page content that will be shown by this tab
- Returns
- the index of the inserted tab
Definition at line 317 of file tabbar.cpp.
bool Plasma::TabBar::isTabBarShown | ( | ) | const |
bool Plasma::TabBar::isTabHighlighted | ( | int | index | ) | const |
QGraphicsWidget* Plasma::TabBar::lastPositionWidget | ( | ) | const |
- Returns
- the widget in the last position
- Since
- 4.6
KTabBar* Plasma::TabBar::nativeWidget | ( | ) | const |
- Returns
- the native widget wrapped by this TabBar
void Plasma::TabBar::removeTab | ( | int | index | ) |
Removes a tab, contents are deleted.
- Parameters
-
index the index of the tab to remove
Definition at line 434 of file tabbar.cpp.
|
protected |
Definition at line 337 of file tabbar.cpp.
|
slot |
Activate a given tab.
- Parameters
-
index the index of the tab to activate
Definition at line 350 of file tabbar.cpp.
void Plasma::TabBar::setFirstPositionWidget | ( | QGraphicsWidget * | widget | ) |
Set a widget to be displayed on one side of the TabBar, depending on the LayoutDirection and the Shape.
- Parameters
-
widget the widget to be displayed. Passing 0 will show nothing. Any previous widget will be deleted.
- Since
- 4.6
Definition at line 620 of file tabbar.cpp.
void Plasma::TabBar::setLastPositionWidget | ( | QGraphicsWidget * | widget | ) |
Set a widget to be displayed on one side of the TabBar, depending on the LayoutDirection and the Shape.
- Parameters
-
widget the widget to be displayed. Passing 0 will show nothing. Any previous widget will be deleted.
- Since
- 4.6
Definition at line 650 of file tabbar.cpp.
void Plasma::TabBar::setStyleSheet | ( | const QString & | stylesheet | ) |
Sets the stylesheet used to control the visual display of this TabBar.
- Parameters
-
stylesheet a CSS string
Definition at line 583 of file tabbar.cpp.
void Plasma::TabBar::setTabBarShown | ( | bool | show | ) |
shows or hides the tabbar, used if you just want to display the pages, when the tabbar doesn't have content pages at all this function has no effect
- Parameters
-
show true if we want to show the tabbar
- Since
- 4.3
Definition at line 559 of file tabbar.cpp.
void Plasma::TabBar::setTabHighlighted | ( | int | index, |
bool | highlight | ||
) |
Highlight the specified tab.
- Parameters
-
index of the tab to highlight highlight true if it should be highlighted, wrong if not
- Since
- 4.7
Definition at line 593 of file tabbar.cpp.
void Plasma::TabBar::setTabIcon | ( | int | index, |
const QIcon & | icon | ||
) |
Sets an icon for a given tab.
- Parameters
-
index the index of the tab to modify icon the new icon for the given tab
Definition at line 549 of file tabbar.cpp.
void Plasma::TabBar::setTabText | ( | int | index, |
const QString & | label | ||
) |
Sets the text label of the given tab.
- Parameters
-
index the index of the tab to modify label the new text label of the given tab
Definition at line 535 of file tabbar.cpp.
QString Plasma::TabBar::styleSheet | ( | ) | const |
- Returns
- the stylesheet currently used with this widget
QGraphicsLayoutItem * Plasma::TabBar::tabAt | ( | int | index | ) |
Returns the contents of a page.
- Parameters
-
index the index of the tab to retrieve
- Since
- 4.4
Definition at line 516 of file tabbar.cpp.
QIcon Plasma::TabBar::tabIcon | ( | int | index | ) | const |
- Returns
- the current icon for a given tab
- Parameters
-
index the index of the tab we want to know its icon
Definition at line 554 of file tabbar.cpp.
QString Plasma::TabBar::tabText | ( | int | index | ) | const |
- Returns
- the text label of the given tab
- Parameters
-
index the index of the tab we want to know its label
Definition at line 544 of file tabbar.cpp.
QGraphicsLayoutItem * Plasma::TabBar::takeTab | ( | int | index | ) |
Removes a tab, the page is reparented to 0 and is returned.
- Parameters
-
index the index of the tab to remove
- Since
- 4.4
Definition at line 467 of file tabbar.cpp.
|
protected |
Definition at line 608 of file tabbar.cpp.
Property Documentation
|
readwrite |
|
readwrite |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.