Plasma
#include <Plasma/Widgets/VideoWidget>
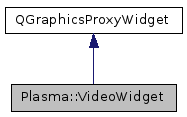
Public Types | |
enum | Control { NoControls = 0, Play = 1, Pause = 2, Stop = 4, PlayPause = 8, Previous = 16, Next = 32, Progress = 64, Volume = 128, OpenFile = 128, DefaultControls = PlayPause|Progress|Volume|OpenFile } |
Public Slots | |
void | pause () |
void | play () |
void | seek (qint64 time) |
void | stop () |
Signals | |
void | aboutToFinish () |
void | nextRequested () |
void | previousRequested () |
void | tick (qint64 time) |
Public Member Functions | |
VideoWidget (QGraphicsWidget *parent=0) | |
~VideoWidget () | |
Q_INVOKABLE Phonon::AudioOutput * | audioOutput () const |
bool | controlsVisible () const |
qint64 | currentTime () const |
Q_INVOKABLE Phonon::MediaObject * | mediaObject () const |
Phonon::VideoWidget * | nativeWidget () const |
qint64 | remainingTime () const |
void | setControlsVisible (bool visible) |
void | setStyleSheet (const QString &stylesheet) |
void | setTickInterval (qint64 interval) |
void | setUrl (const QString &url) |
void | setUsedControls (const Controls controls) |
QString | styleSheet () |
qint64 | tickInterval () const |
qint64 | totalTime () const |
QString | url () const |
Controls | usedControls () const |
Protected Member Functions | |
void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
void | hoverMoveEvent (QGraphicsSceneHoverEvent *event) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
Properties | |
bool | controlsVisible |
qint64 | currentTime |
qint64 | remainingTime |
QString | styleSheet |
qint32 | tickInterval |
qint64 | totalTime |
QString | url |
Controls | usedControls |
Detailed Description
a Video playing widget via Phonon, it encloses the Phonon::MediaObject and Phonon::AudioOutput too
Provides a video player widget
- Since
- KDE4.3
Definition at line 48 of file videowidget.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoControls | |
Play | |
Pause | |
Stop | |
PlayPause | |
Previous | |
Next | |
Progress | |
Volume | |
OpenFile | |
DefaultControls |
Definition at line 63 of file videowidget.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 248 of file videowidget.cpp.
Plasma::VideoWidget::~VideoWidget | ( | ) |
Definition at line 268 of file videowidget.cpp.
Member Function Documentation
|
signal |
Emitted an instant before the playback is finished.
Phonon::AudioOutput * Plasma::VideoWidget::audioOutput | ( | ) | const |
- Returns
- the Phonon::AudioOutput being used
- See also
- Phonon::AudioOutput
Definition at line 278 of file videowidget.cpp.
bool Plasma::VideoWidget::controlsVisible | ( | ) | const |
- Returns
- true if the controls widget is being shown right now
qint64 Plasma::VideoWidget::currentTime | ( | ) | const |
- Returns
- the current time of the current media file
|
protected |
Definition at line 600 of file videowidget.cpp.
|
protected |
Definition at line 611 of file videowidget.cpp.
|
protected |
Definition at line 620 of file videowidget.cpp.
Phonon::MediaObject * Plasma::VideoWidget::mediaObject | ( | ) | const |
- Returns
- the Phonon::MediaObject being used
- See also
- Phonon::MediaObject
Definition at line 273 of file videowidget.cpp.
Phonon::VideoWidget * Plasma::VideoWidget::nativeWidget | ( | ) | const |
- Returns
- the native widget wrapped by this VideoWidget
Definition at line 565 of file videowidget.cpp.
|
signal |
The user pressed the "next" button.
- Since
- 4.3
|
slot |
Pause the current file.
Definition at line 490 of file videowidget.cpp.
|
slot |
Play the current file.
Definition at line 481 of file videowidget.cpp.
|
signal |
The user pressed the "previous" button.
- Since
- 4.3
qint64 Plasma::VideoWidget::remainingTime | ( | ) | const |
- Returns
- the time remaining to the current media file
|
protected |
Definition at line 571 of file videowidget.cpp.
|
slot |
Jump at a given millisecond in the current file.
- Parameters
-
time where we want to jump
Definition at line 508 of file videowidget.cpp.
void Plasma::VideoWidget::setControlsVisible | ( | bool | visible | ) |
Show/hide the main controls widget, if any of them is used.
- Parameters
-
visible if we want to show or hide the main controls
- See also
- setUsedControls()
Definition at line 532 of file videowidget.cpp.
void Plasma::VideoWidget::setStyleSheet | ( | const QString & | stylesheet | ) |
Sets the stylesheet used to control the visual display of this VideoWidget.
- Parameters
-
stylesheet a CSS string
Definition at line 555 of file videowidget.cpp.
void Plasma::VideoWidget::setTickInterval | ( | qint64 | interval | ) |
- Parameters
-
interval milliseconds the tick signal will be emitted
- Since
- 4.7.1
Definition at line 545 of file videowidget.cpp.
void Plasma::VideoWidget::setUrl | ( | const QString & | url | ) |
Load a certain url that can be a local file or a remote one.
- Parameters
-
path resource to play
Definition at line 283 of file videowidget.cpp.
void Plasma::VideoWidget::setUsedControls | ( | const Controls | controls | ) |
Set what control widgets to use.
- Parameters
-
controls bitwise OR combination of Controls flags
- See also
- Controls
Definition at line 304 of file videowidget.cpp.
|
slot |
Stop the current file.
Definition at line 499 of file videowidget.cpp.
QString Plasma::VideoWidget::styleSheet | ( | ) |
- Returns
- the stylesheet currently used with this widget
|
signal |
Emitted regularly when the playing is progressing.
- Parameters
-
time where we are
qint64 Plasma::VideoWidget::tickInterval | ( | ) | const |
qint64 Plasma::VideoWidget::totalTime | ( | ) | const |
- Returns
- the total playing time of the current media file
QString Plasma::VideoWidget::url | ( | ) | const |
- Returns
- the url (local or remote) we are playing
Controls Plasma::VideoWidget::usedControls | ( | ) | const |
- Returns
- the video controls that are used and shown
- See also
- Controls
Property Documentation
|
readwrite |
Definition at line 57 of file videowidget.h.
|
read |
Definition at line 53 of file videowidget.h.
|
read |
Definition at line 55 of file videowidget.h.
|
readwrite |
Definition at line 59 of file videowidget.h.
|
readwrite |
Definition at line 58 of file videowidget.h.
|
read |
Definition at line 54 of file videowidget.h.
|
readwrite |
Definition at line 52 of file videowidget.h.
|
readwrite |
Definition at line 56 of file videowidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.