Solid
#include <audiointerface.h>
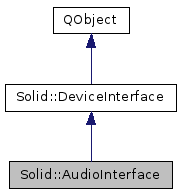
Public Types | |
enum | AudioDriver { Alsa, OpenSoundSystem, UnknownAudioDriver } |
enum | AudioInterfaceType { UnknownAudioInterfaceType = 0, AudioControl = 1, AudioInput = 2, AudioOutput = 4 } |
enum | SoundcardType { InternalSoundcard, UsbSoundcard, FirewireSoundcard, Headset, Modem } |
![]() | |
enum | Type { Unknown = 0, GenericInterface = 1, Processor = 2, Block = 3, StorageAccess = 4, StorageDrive = 5, OpticalDrive = 6, StorageVolume = 7, OpticalDisc = 8, Camera = 9, PortableMediaPlayer = 10, NetworkInterface = 11, AcAdapter = 12, Battery = 13, Button = 14, AudioInterface = 15, DvbInterface = 16, Video = 17, SerialInterface = 18, SmartCardReader = 19, InternetGateway = 20, NetworkShare = 21, Last = 0xffff } |
Public Member Functions | |
virtual | ~AudioInterface () |
AudioInterfaceTypes | deviceType () const |
AudioDriver | driver () const |
QVariant | driverHandle () const |
QString | name () const |
SoundcardType | soundcardType () const |
![]() | |
virtual | ~DeviceInterface () |
bool | isValid () const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
Properties | |
AudioInterfaceTypes | deviceType |
AudioDriver | driver |
QVariant | driverHandle |
QString | name |
SoundcardType | soundcardType |
Additional Inherited Members | |
![]() | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
This device interface is available on interfaces exposed by sound cards.
Definition at line 37 of file audiointerface.h.
Member Enumeration Documentation
This enum type defines the type of driver required to interact with the device.
Enumerator | |
---|---|
Alsa |
An Advanced Linux Sound Architecture (ALSA) driver device. |
OpenSoundSystem |
An Open Sound System (OSS) driver device. |
UnknownAudioDriver |
An unknown driver device. |
Definition at line 55 of file audiointerface.h.
This enum type defines the type of audio interface this device expose.
Enumerator | |
---|---|
UnknownAudioInterfaceType |
An unknown audio interface. |
AudioControl |
A control/mixer interface. |
AudioInput |
An audio source. |
AudioOutput |
An audio sink. |
Definition at line 75 of file audiointerface.h.
This type stores an OR combination of AudioInterfaceType values.
This enum defines the type of soundcard of this device.
Definition at line 103 of file audiointerface.h.
Constructor & Destructor Documentation
|
virtual |
Destroys an AudioInterface object.
Definition at line 34 of file audiointerface.cpp.
Member Function Documentation
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the AudioInterface device interface.
- Returns
- the AudioInterface device interface type
- See also
- Solid::DeviceInterface::Type
Definition at line 156 of file audiointerface.h.
AudioInterfaceTypes Solid::AudioInterface::deviceType | ( | ) | const |
Retrieves the type of this audio interface (in/out/control).
- Returns
- the type of this audio interface
AudioDriver Solid::AudioInterface::driver | ( | ) | const |
Retrieves the audio driver that should be used to access the device.
- Returns
- the driver needed to access the device
QVariant Solid::AudioInterface::driverHandle | ( | ) | const |
Retrieves a driver specific handle to access the device.
For Alsa devices it is a list with (card, device, subdevice).
The card entry sometimes can be converted to an integer, but it may just as well be the textual id for the card. So don't rely on it to work with QVariant::toInt().
For OSS devices it is simply a string like "/dev/dsp". Use QVariant::toString() to retrieve the string.
- Returns
- the driver specific data to handle this device
QString Solid::AudioInterface::name | ( | ) | const |
Retrieves the name of this audio interface.
The product name of the parent device is normally better suited for the user to identify the soundcard. If the soundcard has multiple devices, though you need to add this name to differentiate between the devices.
- Returns
- the name of the audio interface if available, QString() otherwise
SoundcardType Solid::AudioInterface::soundcardType | ( | ) | const |
Retrieves the type of soundcard (internal/headset/...).
- Returns
- the type of soundcard
Property Documentation
|
read |
Definition at line 45 of file audiointerface.h.
|
read |
Definition at line 42 of file audiointerface.h.
|
read |
Definition at line 43 of file audiointerface.h.
|
read |
Definition at line 44 of file audiointerface.h.
|
read |
Definition at line 46 of file audiointerface.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.