Solid
#include <battery.h>
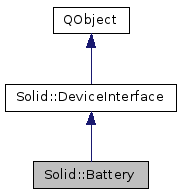
Public Types | |
enum | BatteryType { UnknownBattery, PdaBattery, UpsBattery, PrimaryBattery, MouseBattery, KeyboardBattery, KeyboardMouseBattery, CameraBattery, PhoneBattery, MonitorBattery } |
enum | ChargeState { NoCharge, Charging, Discharging } |
![]() | |
enum | Type { Unknown = 0, GenericInterface = 1, Processor = 2, Block = 3, StorageAccess = 4, StorageDrive = 5, OpticalDrive = 6, StorageVolume = 7, OpticalDisc = 8, Camera = 9, PortableMediaPlayer = 10, NetworkInterface = 11, AcAdapter = 12, Battery = 13, Button = 14, AudioInterface = 15, DvbInterface = 16, Video = 17, SerialInterface = 18, SmartCardReader = 19, InternetGateway = 20, NetworkShare = 21, Last = 0xffff } |
Signals | |
void | capacityChanged (int value, const QString &udi) |
void | chargePercentChanged (int value, const QString &udi) |
void | chargeStateChanged (int newState, const QString &udi) |
void | plugStateChanged (bool newState, const QString &udi) |
void | powerSupplyStateChanged (bool newState, const QString &udi) |
Public Member Functions | |
virtual | ~Battery () |
int | capacity () const |
int | chargePercent () const |
ChargeState | chargeState () const |
bool | isPlugged () const |
bool | isPowerSupply () const |
bool | isRechargeable () const |
BatteryType | type () const |
![]() | |
virtual | ~DeviceInterface () |
bool | isValid () const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
Properties | |
int | capacity |
int | chargePercent |
ChargeState | chargeState |
bool | plugged |
bool | powerSupply |
bool | rechargeable |
BatteryType | type |
Additional Inherited Members | |
![]() | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
Member Enumeration Documentation
This enum type defines the type of the device holding the battery.
- PdaBattery : A battery in a Personal Digital Assistant
- UpsBattery : A battery in an Uninterruptible Power Supply
- PrimaryBattery : A primary battery for the system (for example laptop battery)
- MouseBattery : A battery in a mouse
- KeyboardBattery : A battery in a keyboard
- KeyboardMouseBattery : A battery in a combined keyboard and mouse
- CameraBattery : A battery in a camera
- PhoneBattery : A battery in a phone
- MonitorBattery : A battery in a monitor
- UnknownBattery : A battery in an unknown device
Enumerator | |
---|---|
UnknownBattery | |
PdaBattery | |
UpsBattery | |
PrimaryBattery | |
MouseBattery | |
KeyboardBattery | |
KeyboardMouseBattery | |
CameraBattery | |
PhoneBattery | |
MonitorBattery |
Constructor & Destructor Documentation
|
virtual |
Destroys a Battery object.
Definition at line 46 of file battery.cpp.
Member Function Documentation
int Solid::Battery::capacity | ( | ) | const |
Retrieves the battery capacity normalised to percent, meaning how much energy can it hold compared to what it is designed to.
The capacity of the battery will reduce with age. A capacity value less than 75% is usually a sign that you should renew your battery.
- Since
- 4.11
- Returns
- the battery capacity normalised to percent
|
signal |
This signal is emitted when the capacity of this battery has changed.
- Parameters
-
value the new capacity of the battery udi the UDI of the battery with the new capacity
- Since
- 4.11
int Solid::Battery::chargePercent | ( | ) | const |
Retrieves the current charge level of the battery normalised to percent.
- Returns
- the current charge level normalised to percent
|
signal |
This signal is emitted when the charge percent value of this battery has changed.
- Parameters
-
value the new charge percent value of the battery udi the UDI of the battery with the new charge percent
ChargeState Solid::Battery::chargeState | ( | ) | const |
Retrieves the current charge state of the battery.
It can be in a stable state (no charge), charging or discharging.
- Returns
- the current battery charge state
- See also
- Solid::Battery::ChargeState
|
signal |
This signal is emitted when the charge state of this battery has changed.
- Parameters
-
newState the new charge state of the battery, it's one of the type Solid::Battery::ChargeState
- See also
- Solid::Battery::ChargeState
- Parameters
-
udi the UDI of the battery with the new charge state
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the Battery device interface.
- Returns
- the Battery device interface type
- See also
- Solid::DeviceInterface::Type
bool Solid::Battery::isPlugged | ( | ) | const |
Indicates if this battery is plugged.
- Returns
- true if the battery is plugged, false otherwise
Definition at line 51 of file battery.cpp.
bool Solid::Battery::isPowerSupply | ( | ) | const |
Indicates if this battery is powering the machine or from an attached deviced.
- Since
- 4.11
- Returns
- true the battery is a powersupply, false otherwise
Definition at line 57 of file battery.cpp.
bool Solid::Battery::isRechargeable | ( | ) | const |
Indicates if the battery is rechargeable.
- Returns
- true if the battery is rechargeable, false otherwise (one time usage)
Definition at line 81 of file battery.cpp.
|
signal |
This signal is emitted if the battery get plugged in/out of the battery bay.
- Parameters
-
newState the new plugging state of the battery, type is boolean udi the UDI of the battery with the new plugging state
|
signal |
This signal is emitted when the power supply state of the battery changes.
- Parameters
-
newState the new power supply state, type is boolean udi the UDI of the battery with the new power supply state
- Since
- 4.11
BatteryType Solid::Battery::type | ( | ) | const |
Retrieves the type of device holding this battery.
- Returns
- the type of device holding this battery
- See also
- Solid::Battery::BatteryType
Property Documentation
|
read |
|
read |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.