Solid
#include <genericinterface.h>
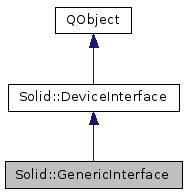
Public Types | |
enum | PropertyChange { PropertyModified, PropertyAdded, PropertyRemoved } |
![]() | |
enum | Type { Unknown = 0, GenericInterface = 1, Processor = 2, Block = 3, StorageAccess = 4, StorageDrive = 5, OpticalDrive = 6, StorageVolume = 7, OpticalDisc = 8, Camera = 9, PortableMediaPlayer = 10, NetworkInterface = 11, AcAdapter = 12, Battery = 13, Button = 14, AudioInterface = 15, DvbInterface = 16, Video = 17, SerialInterface = 18, SmartCardReader = 19, InternetGateway = 20, NetworkShare = 21, Last = 0xffff } |
Signals | |
void | conditionRaised (const QString &condition, const QString &reason) |
void | propertyChanged (const QMap< QString, int > &changes) |
Public Member Functions | |
virtual | ~GenericInterface () |
QMap< QString, QVariant > | allProperties () const |
QVariant | property (const QString &key) const |
bool | propertyExists (const QString &key) const |
![]() | |
virtual | ~DeviceInterface () |
bool | isValid () const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
Additional Inherited Members | |
![]() | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
Generic interface to deal with a device.
It exposes a set of properties and is organized as a key/value set.
Warning: Using this class could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
Definition at line 44 of file genericinterface.h.
Member Enumeration Documentation
This enum type defines the type of change that can occur to a GenericInterface property.
- PropertyModified : A property value has changed in the device
- PropertyAdded : A new property has been added to the device
- PropertyRemoved : A property has been removed from the device
Enumerator | |
---|---|
PropertyModified | |
PropertyAdded | |
PropertyRemoved |
Definition at line 60 of file genericinterface.h.
Constructor & Destructor Documentation
|
virtual |
Destroys a Processor object.
Definition at line 40 of file genericinterface.cpp.
Member Function Documentation
QMap< QString, QVariant > Solid::GenericInterface::allProperties | ( | ) | const |
Retrieves a key/value map of all the known properties for the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Returns
- all the properties of the device
Definition at line 51 of file genericinterface.cpp.
|
signal |
This signal is emitted when an event occurred in the device.
For example when a button is pressed.
- Parameters
-
condition the condition name reason a message explaining why the condition has been raised
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the GenericInterface device interface.
- Returns
- the Processor device interface type
- See also
- Solid::Ifaces::Enums::DeviceInterface::Type
Definition at line 86 of file genericinterface.h.
QVariant Solid::GenericInterface::property | ( | const QString & | key | ) | const |
Retrieves a property of the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Parameters
-
key the property key
- Returns
- the actual value of the property, or QVariant() if the property is unknown
Definition at line 45 of file genericinterface.cpp.
|
signal |
This signal is emitted when a property is changed in the device.
- Parameters
-
changes the map describing the property changes that occurred in the device, keys are property name and values describe the kind of change done on the device property (added/removed/modified), it's one of the type Solid::Device::PropertyChange
bool Solid::GenericInterface::propertyExists | ( | const QString & | key | ) | const |
Tests if a property exist in the device.
Warning: Using this method could expose some backend specific details and lead to non portable code. Use it at your own risk, or during transitional phases when the provided device interfaces don't provide the necessary methods.
- Parameters
-
key the property key
- Returns
- true if the property is available in the device, false otherwise
Definition at line 57 of file genericinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.