Solid
#include <opticaldrive.h>
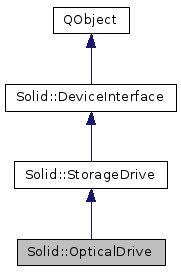
Public Types | |
enum | MediumType { Cdr =0x00001, Cdrw =0x00002, Dvd =0x00004, Dvdr =0x00008, Dvdrw =0x00010, Dvdram =0x00020, Dvdplusr =0x00040, Dvdplusrw =0x00080, Dvdplusdl =0x00100, Dvdplusdlrw =0x00200, Bd =0x00400, Bdr =0x00800, Bdre =0x01000, HdDvd =0x02000, HdDvdr =0x04000, HdDvdrw =0x08000 } |
![]() | |
enum | Bus { Ide, Usb, Ieee1394, Scsi, Sata, Platform } |
enum | DriveType { HardDisk, CdromDrive, Floppy, Tape, CompactFlash, MemoryStick, SmartMedia, SdMmc, Xd } |
![]() | |
enum | Type { Unknown = 0, GenericInterface = 1, Processor = 2, Block = 3, StorageAccess = 4, StorageDrive = 5, OpticalDrive = 6, StorageVolume = 7, OpticalDisc = 8, Camera = 9, PortableMediaPlayer = 10, NetworkInterface = 11, AcAdapter = 12, Battery = 13, Button = 14, AudioInterface = 15, DvbInterface = 16, Video = 17, SerialInterface = 18, SmartCardReader = 19, InternetGateway = 20, NetworkShare = 21, Last = 0xffff } |
Signals | |
void | ejectDone (Solid::ErrorType error, QVariant errorData, const QString &udi) |
void | ejectPressed (const QString &udi) |
void | ejectRequested (const QString &udi) |
Public Member Functions | |
virtual | ~OpticalDrive () |
bool | eject () |
int | readSpeed () const |
MediumTypes | supportedMedia () const |
int | writeSpeed () const |
QList< int > | writeSpeeds () const |
![]() | |
virtual | ~StorageDrive () |
Bus | bus () const |
DriveType | driveType () const |
bool | isHotpluggable () const |
bool | isInUse () const |
bool | isRemovable () const |
qulonglong | size () const |
![]() | |
virtual | ~DeviceInterface () |
bool | isValid () const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
Properties | |
int | readSpeed |
MediumTypes | supportedMedia |
int | writeSpeed |
QList< int > | writeSpeeds |
![]() | |
Bus | bus |
DriveType | driveType |
bool | hotpluggable |
bool | inUse |
bool | removable |
qulonglong | size |
Additional Inherited Members | |
![]() | |
StorageDrive (StorageDrivePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
This device interface is available on CD-R*,DVD*,Blu-Ray,HD-DVD drives.
An OpticalDrive is a storage that can handle optical discs.
Definition at line 42 of file opticaldrive.h.
Member Enumeration Documentation
This enum type defines the type of medium an optical drive supports.
- Cdr : A Recordable Compact Disc (CD-R)
- Cdrw : A ReWritable Compact Disc (CD-RW)
- Dvd : A Digital Versatile Disc (DVD)
- Dvdr : A Recordable Digital Versatile Disc (DVD-R)
- Dvdrw : A ReWritable Digital Versatile Disc (DVD-RW)
- Dvdram : A Random Access Memory Digital Versatile Disc (DVD-RAM)
- Dvdplusr : A Recordable Digital Versatile Disc (DVD+R)
- Dvdplusrw : A ReWritable Digital Versatile Disc (DVD+RW)
- Dvdplusdl : A Dual Layer Digital Versatile Disc (DVD+R DL)
- Dvdplusdlrw : A Dual Layer Digital Versatile Disc (DVD+RW DL)
- Bd : A Blu-ray Disc (BD)
- Bdr : A Blu-ray Disc Recordable (BD-R)
- Bdre : A Blu-ray Disc Recordable and Eraseable (BD-RE)
- HdDvd : A High Density Digital Versatile Disc (HD DVD)
- HdDvdr : A High Density Digital Versatile Disc Recordable (HD DVD-R)
- HdDvdrw : A High Density Digital Versatile Disc ReWritable (HD DVD-RW)
Enumerator | |
---|---|
Cdr | |
Cdrw | |
Dvd | |
Dvdr | |
Dvdrw | |
Dvdram | |
Dvdplusr | |
Dvdplusrw | |
Dvdplusdl | |
Dvdplusdlrw | |
Bd | |
Bdr | |
Bdre | |
HdDvd | |
HdDvdr | |
HdDvdrw |
Definition at line 75 of file opticaldrive.h.
Constructor & Destructor Documentation
|
virtual |
Destroys an OpticalDrive object.
Definition at line 38 of file opticaldrive.cpp.
Member Function Documentation
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the OpticalDrive device interface.
- Returns
- the OpticalDrive device interface type
- See also
- Solid::Ifaces::Enums::DeviceInterface::Type
Definition at line 111 of file opticaldrive.h.
bool Solid::OpticalDrive::eject | ( | ) |
Ejects any disc that could be contained in this drive.
If this drive is empty, but has a tray it'll be opened.
- Returns
- the status of the eject operation
Definition at line 67 of file opticaldrive.cpp.
|
signal |
This signal is emitted when the attempted eject process on this drive is completed.
The signal might be spontaneous, i.e. it can be triggered by another process.
- Parameters
-
error type of error that occurred, if any errorData more information about the error, if any udi the UDI of the volume
|
signal |
This signal is emitted when the eject button is pressed on the drive.
Please note that some (broken) drives doesn't report this event.
- Parameters
-
udi the UDI of the drive
|
signal |
This signal is emitted when eject on this drive is requested.
The signal might be spontaneous, i.e. it can be triggered by another process.
- Parameters
-
udi the UDI of the volume
int Solid::OpticalDrive::readSpeed | ( | ) | const |
Retrieves the maximum read speed of this drive in kilobytes per second.
- Returns
- the maximum read speed
MediumTypes Solid::OpticalDrive::supportedMedia | ( | ) | const |
Retrieves the medium types this drive supports.
- Returns
- the flag set indicating the supported medium types
int Solid::OpticalDrive::writeSpeed | ( | ) | const |
Retrieves the maximum write speed of this drive in kilobytes per second.
- Returns
- the maximum write speed
QList<int> Solid::OpticalDrive::writeSpeeds | ( | ) | const |
Retrieves the list of supported write speeds of this drive in kilobytes per second.
- Returns
- the list of supported write speeds
Property Documentation
|
read |
Definition at line 48 of file opticaldrive.h.
|
read |
Definition at line 47 of file opticaldrive.h.
|
read |
Definition at line 49 of file opticaldrive.h.
|
read |
Definition at line 50 of file opticaldrive.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.