kget
#include <filemodel.h>
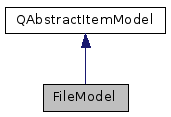
Public Slots | |
void | stopWatchCheckState () |
void | watchCheckState () |
Signals | |
void | checkStateChanged () |
void | fileFinished (const KUrl &file) |
void | rename (const KUrl &oldUrl, const KUrl &newUrl) |
Public Member Functions | |
FileModel (const QList< KUrl > &files, const KUrl &destDirectory, QObject *parent=0) | |
~FileModel () | |
int | columnCount (const QModelIndex &parent=QModelIndex()) const |
QVariant | data (const QModelIndex &index, int role) const |
bool | downloadFinished (const KUrl &file) |
QModelIndexList | fileIndexes (int column) const |
Qt::ItemFlags | flags (const QModelIndex &index) const |
KUrl | getUrl (const QModelIndex &index) |
QVariant | headerData (int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
QModelIndex | index (const KUrl &file, int column) |
bool | isFile (const QModelIndex &index) const |
QModelIndex | parent (const QModelIndex &index) const |
void | rename (const QModelIndex &file, const QString &newName) |
int | rowCount (const QModelIndex &parent=QModelIndex()) const |
void | setCustomStatusIcon (Job::Status status, const KIcon &icon) |
void | setCustomStatusText (Job::Status status, const QString &text) |
bool | setData (const QModelIndex &index, const QVariant &value, int role=Qt::EditRole) |
void | setDirectory (const KUrl &newDirectory) |
Detailed Description
This model represents the files that are being downloaded.
- Note
- whenever a method takes a url as argument use the url to the file destination on your hard disk, the file does not need to exist though
Definition at line 101 of file filemodel.h.
Constructor & Destructor Documentation
FileModel::FileModel | ( | const QList< KUrl > & | files, |
const KUrl & | destDirectory, | ||
QObject * | parent = 0 |
||
) |
Definition at line 277 of file filemodel.cpp.
FileModel::~FileModel | ( | ) |
Definition at line 288 of file filemodel.cpp.
Member Function Documentation
|
signal |
int FileModel::columnCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Definition at line 332 of file filemodel.cpp.
QVariant FileModel::data | ( | const QModelIndex & | index, |
int | role | ||
) | const |
Definition at line 344 of file filemodel.cpp.
bool FileModel::downloadFinished | ( | const KUrl & | file | ) |
Checks if the download for file has been finished.
Definition at line 622 of file filemodel.cpp.
|
signal |
QModelIndexList FileModel::fileIndexes | ( | int | column | ) | const |
Returns a list of pointers to all files of this model.
- Note
- it would be possible to directly interact with the model data that way, though that is discouraged
Definition at line 475 of file filemodel.cpp.
Qt::ItemFlags FileModel::flags | ( | const QModelIndex & | index | ) | const |
Definition at line 411 of file filemodel.cpp.
KUrl FileModel::getUrl | ( | const QModelIndex & | index | ) |
The url on the filesystem (no check if the file exists yet!) of index, it can be a folder or file.
Definition at line 544 of file filemodel.cpp.
QVariant FileModel::headerData | ( | int | section, |
Qt::Orientation | orientation, | ||
int | role = Qt::DisplayRole |
||
) | const |
Definition at line 426 of file filemodel.cpp.
QModelIndex FileModel::index | ( | int | row, |
int | column, | ||
const QModelIndex & | parent = QModelIndex() |
||
) | const |
Definition at line 436 of file filemodel.cpp.
QModelIndex FileModel::index | ( | const KUrl & | file, |
int | column | ||
) |
Definition at line 464 of file filemodel.cpp.
bool FileModel::isFile | ( | const QModelIndex & | index | ) | const |
Returns true if the index represents a file.
Definition at line 637 of file filemodel.cpp.
QModelIndex FileModel::parent | ( | const QModelIndex & | index | ) | const |
Definition at line 487 of file filemodel.cpp.
void FileModel::rename | ( | const QModelIndex & | file, |
const QString & | newName | ||
) |
Definition at line 649 of file filemodel.cpp.
|
signal |
int FileModel::rowCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Definition at line 506 of file filemodel.cpp.
void FileModel::setCustomStatusIcon | ( | Job::Status | status, |
const KIcon & | icon | ||
) |
Set a custom status icon for status.
- Note
- KIcon() removes the custom icon for status
void FileModel::setCustomStatusText | ( | Job::Status | status, |
const QString & | text | ||
) |
Set a custom status text for status.
- Note
- QString() removes the custom text for status
bool FileModel::setData | ( | const QModelIndex & | index, |
const QVariant & | value, | ||
int | role = Qt::EditRole |
||
) |
Definition at line 390 of file filemodel.cpp.
void FileModel::setDirectory | ( | const KUrl & | newDirectory | ) |
Set the url to the directory the files are stored in, the filemodel stores its entries as relative path to the base directory.
- Parameters
-
newDirectory the base directory for the model
Definition at line 538 of file filemodel.cpp.
|
slot |
Emits checkStateChanged if a CheckState of an entry changend.
Definition at line 689 of file filemodel.cpp.
|
slot |
Watches if the check state changes, the result of that will be emitted when stopWatchCheckState() is being called()
Definition at line 684 of file filemodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.