kget
#include <transferdatasource.h>
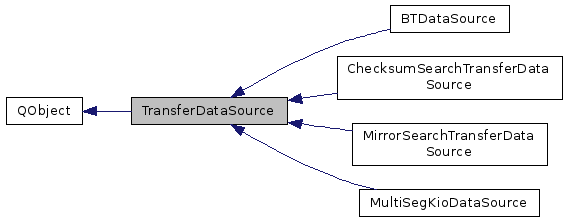
Public Types | |
enum | Error { Unknown, WrongDownloadSize, NotResumeable } |
Signals | |
void | broken (TransferDataSource *source, TransferDataSource::Error error) |
void | brokenSegments (TransferDataSource *source, QPair< int, int > segmentRange) |
void | capabilitiesChanged () |
void | data (KIO::fileoffset_t offset, const QByteArray &data, bool &worked) |
void | data (const QList< KUrl > &data) |
void | data (const QString type, const QString checksum) |
void | finished () |
void | finishedDownload (TransferDataSource *source, KIO::filesize_t fileSize) |
void | finishedSegment (TransferDataSource *source, int segmentNumber, bool connectionFinished=true) |
void | foundFileSize (TransferDataSource *source, KIO::filesize_t fileSize, const QPair< int, int > &segmentRange) |
void | freeSegments (TransferDataSource *source, QPair< int, int > segmentRange) |
void | log (const QString &message, Transfer::LogLevel logLevel) |
void | speed (ulong speed) |
void | urlChanged (const KUrl &old, const KUrl &newUrl) |
Public Member Functions | |
TransferDataSource (const KUrl &srcUrl, QObject *parent) | |
virtual | ~TransferDataSource () |
virtual void | addSegments (const QPair< KIO::fileoffset_t, KIO::fileoffset_t > &segmentSize, const QPair< int, int > &segmentRange)=0 |
virtual QList< QPair< int, int > > | assignedSegments () const |
Transfer::Capabilities | capabilities () const |
virtual int | changeNeeded () const |
virtual int | countUnfinishedSegments () const |
virtual int | currentSegments () const |
ulong | currentSpeed () const |
virtual void | findFileSize (KIO::fileoffset_t segmentSize) |
virtual int | paralellSegments () const |
virtual QPair< int, int > | removeConnection () |
virtual void | setParalellSegments (int paralellSegments) |
virtual void | setSupposedSize (KIO::filesize_t supposedSize) |
KUrl | sourceUrl () const |
virtual QPair< int, int > | split () |
virtual void | start ()=0 |
virtual void | stop ()=0 |
Protected Member Functions | |
void | setCapabilities (Transfer::Capabilities capabilities) |
Protected Attributes | |
int | m_currentSegments |
int | m_paralellSegments |
KUrl | m_sourceUrl |
ulong | m_speed |
KIO::filesize_t | m_supposedSize |
Detailed Description
This Class is an interface for inter-plugins data change.
allowing to use already implemented features from others plugins
Definition at line 26 of file transferdatasource.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Unknown | |
WrongDownloadSize | |
NotResumeable |
Definition at line 33 of file transferdatasource.h.
Constructor & Destructor Documentation
TransferDataSource::TransferDataSource | ( | const KUrl & | srcUrl, |
QObject * | parent | ||
) |
Definition at line 15 of file transferdatasource.cpp.
|
virtual |
Definition at line 27 of file transferdatasource.cpp.
Member Function Documentation
|
pure virtual |
Adds multiple continuous segments that should be downloaded by this TransferDataSource.
- Parameters
-
segmentSize first is always the general segmentSize, second the segmentSize of the last segment in the range. If just one (the last) segment was assigned, then first would not equal second, this is to ensure that first can be used to calculate the offset TransferDataSources have to handle all that internally. segmentRange first the beginning, second the end
Implemented in ChecksumSearchTransferDataSource, BTDataSource, MultiSegKioDataSource, and MirrorSearchTransferDataSource.
|
virtual |
Returns the assignedSegments to this TransferDataSource Each connection is represented by a QPair, where the first int is the beginning segment and the last the ending segment.
- Note
- an empty list is returned by default, the elements can also be (-1, -1)
Reimplemented in MultiSegKioDataSource.
Definition at line 53 of file transferdatasource.cpp.
|
signal |
Alert that datasource is no able to send any data.
- Parameters
-
source the datasource, sending the signal
|
signal |
emitted when an assigned segment is broken
- Parameters
-
source the source that emmited this signal segmentRange the range of the segments e.g. (1,1,) or (0, 10)
Transfer::Capabilities TransferDataSource::capabilities | ( | ) | const |
Returns the capabilities this TransferDataSource supports.
Definition at line 32 of file transferdatasource.cpp.
|
signal |
Emitted when the capabilities of the TransferDataSource change.
|
virtual |
Returns the missmatch of paralellSegments() and currentSegments()
- Returns
- the number of segments to add/remove e.g. -1 means one segment to remove
Definition at line 83 of file transferdatasource.cpp.
|
virtual |
Returns the number of unfinished Segments of the connection with the most unfinished segments Each TransferDataSource can have multiple connections and each connection can have multiple segments assigned.
- Note
- default implemention returns 0
Reimplemented in MultiSegKioDataSource.
Definition at line 58 of file transferdatasource.cpp.
|
virtual |
- Returns
- the number of paralell segments this DataSources currently uses
Reimplemented in MultiSegKioDataSource.
Definition at line 78 of file transferdatasource.cpp.
|
inline |
returns the current speed of this data source
- Returns
- the speed
Definition at line 82 of file transferdatasource.h.
|
signal |
Returns data in the forms of chucks.
- Note
- if the receiver set worked to wrong the TransferDataSource should cache the data
- Parameters
-
offset the offset in the file data the downloaded data worked if the receiver could handle the data, if not, the sender should cache the data
|
signal |
Returns data in the forms of URL List.
- Parameters
-
data in form of KUrl list
|
signal |
Returns found checksums with their type.
- Parameters
-
type the type of the checksum checksum the checksum
|
virtual |
Tries to find the filesize if this capability is supported, if successfull it emits foundFileSize(TransferDataSource*,KIO::filesize_t,QPair<int,int>) and assigns all segements to itself if not succesfull it will try to download the file nevertheless.
- Note
- if stop is called and no size is found yet then this is aborted, i.e. needs to be called again if start is later called
- Parameters
-
segmentSize the segments should have
Reimplemented in MultiSegKioDataSource.
Definition at line 43 of file transferdatasource.cpp.
|
signal |
emitted when there is no more data
- Parameters
-
source the datasource, sending the signal
|
signal |
Emitted when the TransferDataSource finished the download on its own, e.g.
when findFileSize is being called but no fileSize is found and instead the download finishes
- Parameters
-
source the source that emmited this signal fileSize the fileSize of the finished file (calculated by the downloaded bytes)
|
signal |
emitted when an assigned segment finishes
- Parameters
-
source the source that emmited this signal segmentNumber the number of the segment, to identify it connectionFinished true if all segments of this connection have been finished, if one segement (instead of a group of segments) has been asigned this is always true
|
signal |
Emitted after findFileSize is called successfully.
- Parameters
-
source that foudn the filesize fileSize that was found segmentRange that was calculated based on the segmentSize and that was assigned to source automatically
|
signal |
Emitted when a Datasource itself decides to not download a specific segmentRange, e.g.
when there are too many connections for this TransferDataSource
|
signal |
|
virtual |
- Returns
- the number of paralell segments this DataSource is allowed to use, default is 1
Definition at line 68 of file transferdatasource.cpp.
|
virtual |
Removes one connection, useful when setMaximumParalellDownloads was called with a lower number.
- Returns
- the segments that are removed (unassigned) now
Reimplemented in MultiSegKioDataSource.
Definition at line 48 of file transferdatasource.cpp.
|
protected |
Sets the capabilities and automatically emits capabilitiesChanged.
Definition at line 37 of file transferdatasource.cpp.
|
virtual |
Sets the number of paralell segments this DataSource is allowed to use.
Definition at line 73 of file transferdatasource.cpp.
|
inlinevirtual |
Set the size the server used for downloading should report.
- Parameters
-
supposedSize the size the file should have
Reimplemented in MultiSegKioDataSource.
Definition at line 88 of file transferdatasource.h.
|
inline |
Definition at line 76 of file transferdatasource.h.
|
signal |
The speed of the download.
- Parameters
-
speed speed of the download
|
virtual |
If a connection of this TransferDataSource is assigned multiple (continuous) segments, then this method will split them (the unfinished ones) in half, it returns the beginning and the end of the now unassigned segments; (-1, -1) if there are none.
- Note
- if only one segment is assigned to a connection split will also return (-1, -1)
Reimplemented in MultiSegKioDataSource.
Definition at line 63 of file transferdatasource.cpp.
|
pure virtual |
Implemented in ChecksumSearchTransferDataSource, BTDataSource, MultiSegKioDataSource, and MirrorSearchTransferDataSource.
|
pure virtual |
Implemented in ChecksumSearchTransferDataSource, BTDataSource, MultiSegKioDataSource, and MirrorSearchTransferDataSource.
|
signal |
Emitted when the filename of a url changes, e.g.
when a link redirects
Member Data Documentation
|
protected |
Definition at line 246 of file transferdatasource.h.
|
protected |
Definition at line 245 of file transferdatasource.h.
|
protected |
Definition at line 242 of file transferdatasource.h.
|
protected |
Definition at line 243 of file transferdatasource.h.
|
protected |
Definition at line 244 of file transferdatasource.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.