kget
#include <verifier.h>
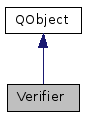
Public Types | |
enum | ChecksumStrength { Weak, Strong, Strongest } |
enum | VerificationStatus { NoResult, NotVerified, Verified } |
Signals | |
void | brokenPieces (const QList< KIO::fileoffset_t > &offsets, KIO::filesize_t length) |
void | verified (bool verified) |
Public Member Functions | |
Verifier (const KUrl &dest, QObject *parent=0) | |
~Verifier () | |
void | addChecksum (const QString &type, const QString &checksum, int verified=0) |
void | addChecksums (const QHash< QString, QString > &checksums) |
void | addPartialChecksums (const QString &type, KIO::filesize_t length, const QStringList &checksums) |
Checksum | availableChecksum (ChecksumStrength strength) const |
QList< Checksum > | availableChecksums () const |
QPair< QString, PartialChecksums * > | availablePartialChecksum (Verifier::ChecksumStrength strength) const |
void | brokenPieces () const |
QString | dBusObjectPath () const |
KUrl | destination () const |
bool | isVerifyable () const |
bool | isVerifyable (const QModelIndex &index) const |
void | load (const QDomElement &e) |
VerificationModel * | model () |
KIO::filesize_t | partialChunkLength () const |
void | save (const QDomElement &element) |
void | setDestination (const KUrl &destination) |
VerificationStatus | status () const |
void | verify (const QModelIndex &index=QModelIndex()) |
Static Public Member Functions | |
static QString | checksum (const KUrl &dest, const QString &type, bool *abortPtr) |
static QString | cleanChecksumType (const QString &type) |
static int | diggestLength (const QString &type) |
static bool | isChecksum (const QString &type, const QString &checksum) |
static PartialChecksums | partialChecksums (const KUrl &dest, const QString &type, KIO::filesize_t length=0, bool *abortPtr=0) |
static QStringList | supportedVerficationTypes () |
Detailed Description
Definition at line 68 of file verifier.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Weak | |
Strong | |
Strongest |
Definition at line 83 of file verifier.h.
Enumerator | |
---|---|
NoResult | |
NotVerified | |
Verified |
Definition at line 76 of file verifier.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 163 of file verifier.cpp.
Verifier::~Verifier | ( | ) |
Definition at line 186 of file verifier.cpp.
Member Function Documentation
void Verifier::addChecksum | ( | const QString & | type, |
const QString & | checksum, | ||
int | verified = 0 |
||
) |
Add a checksum that is later used in the verification process.
- Note
- only one checksum per type can be added (one MD5, one SHA1 etc.), the newer overwrites the older and a checksum can only be added if it is supported by the verifier
- Parameters
-
type the type of the checksum checksum the checksum verified if the file has been verified using this checksum
- Note
- uses VerificationModel internally
- See also
- VerificationModel
Definition at line 514 of file verifier.cpp.
void Verifier::addChecksums | ( | const QHash< QString, QString > & | checksums | ) |
Add multiple checksums that will later be used in the verification process.
- Note
- only one checksum per type can be added (one MD5, one SHA1 etc.), the newer overwrites the older and a checksum can only be added if it is supported by the verifier
- Parameters
-
checksums <type, checksum>
- Note
- uses VerificationModel internally
- See also
- VerificationModel
Definition at line 519 of file verifier.cpp.
void Verifier::addPartialChecksums | ( | const QString & | type, |
KIO::filesize_t | length, | ||
const QStringList & | checksums | ||
) |
Add partial checksums that can be used as repairinformation.
- Note
- only one checksum per type can be added (one MD5, one SHA1 etc.), the newer overwrites the older and a checksum can only be added if it is supported by the verifier
- Parameters
-
type the type of the checksums length the length of each piece checksums the checksums, first entry is piece number 0
Definition at line 524 of file verifier.cpp.
Checksum Verifier::availableChecksum | ( | Verifier::ChecksumStrength | strength | ) | const |
Returns a checksum and a type.
- Parameters
-
strength the strength the checksum-type should have; weak is md5 > md4 (md5 preferred), strong is sha1 > ripmed160 > sha256 > sha384 > sha512 (sha1 preferred), strongest is sha512 > sha384 > sha256 .... < (sha512 preferred) If the category does not match then any checksum is taken
Definition at line 298 of file verifier.cpp.
QList< Checksum > Verifier::availableChecksums | ( | ) | const |
Returns all set checksums.
Definition at line 324 of file verifier.cpp.
QPair< QString, PartialChecksums * > Verifier::availablePartialChecksum | ( | Verifier::ChecksumStrength | strength | ) | const |
Returns a PartialChecksum and a type.
- Parameters
-
strength the strength the checksum-type should have; weak is md5 > md4 > ... (md5 preferred), strong is sha1 > ripmed160 > sha256 > sha384 > sha512 > ... (sha1 preferred), strongest is sha512 > sha384 > sha256 ... (sha512 preferred) If the category does not match then any checksum is taken
Definition at line 337 of file verifier.cpp.
void Verifier::brokenPieces | ( | ) | const |
Call this method after calling verify() with a negative result, it will emit a list of the broken pieces, if PartialChecksums were defined, otherwise and in case of any error an empty list will be emitted.
Definition at line 386 of file verifier.cpp.
|
signal |
Emitted when brokenPiecesThreaded finishes, the list can be empty, while length will be always set.
|
static |
Creates the checksum type of the file dest.
- Parameters
-
abortPtr makes it possible to abort the calculation of the checksum from another thread
Definition at line 398 of file verifier.cpp.
|
static |
Cleans the checksum type, that it should match the official name, i.e.
upper case e.g. SHA-1 instead of sha1
Definition at line 269 of file verifier.cpp.
QString Verifier::dBusObjectPath | ( | ) | const |
- Returns
- the object path that will be shown in the DBUS interface
Definition at line 191 of file verifier.cpp.
KUrl Verifier::destination | ( | ) | const |
Definition at line 196 of file verifier.cpp.
|
static |
Returns the diggest length of type.
- Parameters
-
type the checksum type for which to get the diggest length
- Returns
- the length the diggest should have
Definition at line 239 of file verifier.cpp.
|
static |
Tries to check if the checksum is a checksum and if it is supported it compares the diggestLength and checks if there are only alphanumerics in checksum.
- Parameters
-
type of the checksum checksum the checksum you want to check
Definition at line 256 of file verifier.cpp.
bool Verifier::isVerifyable | ( | ) | const |
- Note
- only call verify() when this function returns true
- Returns
- true if the downloaded file exists and a supported checksum is set
Definition at line 279 of file verifier.cpp.
bool Verifier::isVerifyable | ( | const QModelIndex & | index | ) | const |
Convenience function if only a row of the model should be checked.
- Note
- only call verify() when this function returns true
- Parameters
-
row the row in the model of the checksum
- Returns
- true if the downloaded file exists and a supported checksum is set
Definition at line 284 of file verifier.cpp.
void Verifier::load | ( | const QDomElement & | e | ) |
Definition at line 584 of file verifier.cpp.
VerificationModel * Verifier::model | ( | ) |
- Returns
- the model that stores the hash-types and checksums
Definition at line 211 of file verifier.cpp.
|
static |
Create partial checksums of type for file dest.
- Parameters
-
abortPtr makes it possible to abort the calculation of the checksums from another thread
- Note
- the length of the partial checksum (if not defined = 0) is not less than 512 kb and there won't be more partial checksums than 101
Definition at line 448 of file verifier.cpp.
KIO::filesize_t Verifier::partialChunkLength | ( | ) | const |
Returns the length of the "best" partialChecksums.
Definition at line 532 of file verifier.cpp.
void Verifier::save | ( | const QDomElement & | element | ) |
Definition at line 547 of file verifier.cpp.
void Verifier::setDestination | ( | const KUrl & | destination | ) |
Definition at line 201 of file verifier.cpp.
Verifier::VerificationStatus Verifier::status | ( | ) | const |
Definition at line 206 of file verifier.cpp.
|
static |
Returns the supported verification types.
- Returns
- the supported verification types (e.g. MD5, SHA1 ...)
Definition at line 216 of file verifier.cpp.
|
signal |
Emitted when the verification of a file finishes.
void Verifier::verify | ( | const QModelIndex & | index = QModelIndex() | ) |
Call this method if you want to verify() in its own thread, then signals with the result are emitted.
- Parameters
-
row of the model should be checked, if not defined the a checkum defined by Verifier::ChecksumStrength will be used
Definition at line 364 of file verifier.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.