kopete/libkopete
#include <videodevicepool.h>
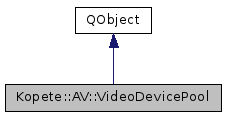
Signals | |
void | deviceRegistered (const QString &udi) |
void | deviceUnregistered (const QString &udi) |
Public Member Functions | |
~VideoDevicePool () | |
int | close () |
int | currentDevice () |
QString | currentDeviceUdi () |
int | currentInput () |
void | fillDeviceKComboBox (KComboBox *combobox) |
void | fillInputKComboBox (KComboBox *combobox) |
void | fillStandardKComboBox (KComboBox *combobox) |
int | getControlValue (quint32 ctrl_id, qint32 *value) |
int | getFrame () |
int | getImage (QImage *qimage) |
QList< ActionVideoControl > | getSupportedActionControls () |
QList< BooleanVideoControl > | getSupportedBooleanControls () |
QList< MenuVideoControl > | getSupportedMenuControls () |
QList< NumericVideoControl > | getSupportedNumericControls () |
int | height () |
int | inputs () |
bool | isOpen () |
int | maxHeight () |
int | maxWidth () |
int | minHeight () |
int | minWidth () |
int | open (int device=-1) |
void | saveCurrentDeviceConfig () |
int | selectInput (int newinput) |
int | setControlValue (quint32 ctrl_id, qint32 value) |
int | setImageSize (int newwidth, int newheight) |
int | size () |
int | startCapturing () |
int | stopCapturing () |
int | width () |
Static Public Member Functions | |
static VideoDevicePool * | self () |
Protected Slots | |
void | deviceAdded (const QString &udi) |
void | deviceRemoved (const QString &udi) |
Protected Member Functions | |
int | getSavedDevice () |
void | loadDeviceConfig () |
bool | registerDevice (Solid::Device &dev) |
int | showDeviceCapabilities (int device=-1) |
Protected Attributes | |
int | m_current_device |
QVector< VideoDevice * > | m_videodevices |
Detailed Description
This class allows kopete to check for the existence, open, configure, test, set parameters, grab frames from and close a given video capture card using the Video4Linux API.
Definition at line 46 of file videodevicepool.h.
Constructor & Destructor Documentation
Kopete::AV::VideoDevicePool::~VideoDevicePool | ( | ) |
Destructor of class VideoDevicePool.
Definition at line 89 of file videodevicepool.cpp.
Member Function Documentation
int Kopete::AV::VideoDevicePool::close | ( | ) |
Closes the device.
- Returns
- The success of the operation: EXIT_SUCCESS or EXIT_FAILURE
Definition at line 172 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::currentDevice | ( | ) |
Returns the index of the current device.
- Returns
- The index of the current device or -1 if no device available
Definition at line 215 of file videodevicepool.cpp.
QString Kopete::AV::VideoDevicePool::currentDeviceUdi | ( | ) |
Returns the unique device identifier (UDI) of the currently selected device.
- Returns
- The unique device identifier (UDI) of the currently selected device
Definition at line 228 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::currentInput | ( | ) |
Returns the index of the currently selected input.
- Returns
- The index of the currently selected input or -1, if no input is available
Definition at line 254 of file videodevicepool.cpp.
|
protectedslot |
Slot called when a new device is added to the system.
Checks the device with the specified UDI and adds to the device pool, if it is a valid video device.
- Parameters
-
udi Unique device identifier (UDI) of the device that has been connected
Definition at line 760 of file videodevicepool.cpp.
|
signal |
Provisional signatures, probably more useful to indicate which device was registered.
|
protectedslot |
Removes the device with the specified UDI from the device pool.
- Parameters
-
udi Unique device identifier (UDI) of the device that has been unplugged
Definition at line 779 of file videodevicepool.cpp.
|
signal |
void Kopete::AV::VideoDevicePool::fillDeviceKComboBox | ( | KComboBox * | combobox | ) |
Fills a combobox with the names of all available video devices.
- Parameters
-
combobox Pointer to a KComboBox object
Definition at line 485 of file videodevicepool.cpp.
void Kopete::AV::VideoDevicePool::fillInputKComboBox | ( | KComboBox * | combobox | ) |
Fills a combobox with the names of all available inputs for the currently selected device.
- Parameters
-
combobox Pointer to a KComboBox object
Definition at line 508 of file videodevicepool.cpp.
void Kopete::AV::VideoDevicePool::fillStandardKComboBox | ( | KComboBox * | combobox | ) |
Fills a combobox with the names of the available signal standards for the currently selected device.
- Parameters
-
combobox Pointer to a KComboBox object
Definition at line 535 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::getControlValue | ( | quint32 | ctrl_id, |
qint32 * | value | ||
) |
Reads the value of a video-control.
- Parameters
-
ctrl_id ID of the video-control value Pointer to the variable, which receives the value of the querried video-control. For boolean controls, the value is 0 or 1. For menu-controls, the value is the index of the currently selected option.
- Returns
- The result-code, currently EXIT_SUCCESS or EXIT_FAILURE
Definition at line 340 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::getFrame | ( | ) |
Definition at line 399 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::getImage | ( | QImage * | qimage | ) |
Definition at line 411 of file videodevicepool.cpp.
|
protected |
Returns the index of the saved device.
- Returns
- The index of the saved device or -1, if the saved device is not available
Definition at line 567 of file videodevicepool.cpp.
QList< ActionVideoControl > Kopete::AV::VideoDevicePool::getSupportedActionControls | ( | ) |
Returns the supported action-controls for the current input.
- Returns
- A list of all supported action-controls for the current input
Definition at line 323 of file videodevicepool.cpp.
QList< BooleanVideoControl > Kopete::AV::VideoDevicePool::getSupportedBooleanControls | ( | ) |
Returns the supported boolean controls for the current input.
- Returns
- A list of all supported boolean controls for the current input
Definition at line 297 of file videodevicepool.cpp.
QList< MenuVideoControl > Kopete::AV::VideoDevicePool::getSupportedMenuControls | ( | ) |
Returns the supported menu-controls for the current input.
- Returns
- A list of all supported menu-controls for the current input
Definition at line 310 of file videodevicepool.cpp.
QList< NumericVideoControl > Kopete::AV::VideoDevicePool::getSupportedNumericControls | ( | ) |
Returns the supported numeric controls for the current input.
- Returns
- A list of all supported numeric controls for the current input
Definition at line 284 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::height | ( | ) |
Definition at line 446 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::inputs | ( | ) |
Returns the number of available inputs of the currently selected device.
- Returns
- The number of inputs of the currently selected device
Definition at line 241 of file videodevicepool.cpp.
bool Kopete::AV::VideoDevicePool::isOpen | ( | ) |
Returns true if the currently selected device is open and false othwerise.
- Returns
- True if the device is open, false otherwise
Definition at line 103 of file videodevicepool.cpp.
|
protected |
Loads and applies the configuration for the currently selected device.
Loads the input and the values for all video-controls and applies them.
Definition at line 602 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::maxHeight | ( | ) |
Definition at line 462 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::maxWidth | ( | ) |
Definition at line 438 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::minHeight | ( | ) |
Definition at line 454 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::minWidth | ( | ) |
Definition at line 430 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::open | ( | int | device = -1 | ) |
Opens the video device with the specified index. The previously opened device is closed before.
- Parameters
-
device Index of the device that should be opened. If a negative index is passed (default), the default device will be opened, which is either the saved device (if available) or alternatively the device with index 0.
- Returns
- The result-code, EXIT_SUCCESS or EXIT_FAILURE
Definition at line 119 of file videodevicepool.cpp.
|
protected |
Checks if the given device is a valid video device and adds it do the device list.
- Parameters
-
device The solid device that should be registered
- Returns
- True, if the device has been registered; otherwise returns false
Definition at line 816 of file videodevicepool.cpp.
void Kopete::AV::VideoDevicePool::saveCurrentDeviceConfig | ( | ) |
Saves the current device configuration.
Saves the current device, the current input and the current values for all supported video-controls.
Definition at line 654 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::selectInput | ( | int | input | ) |
Selects the input of the current video device.
- Parameters
-
input Index of input to be selected
- Returns
- The result-code, EXIT_SUCCESS or EXIT_FAILURE
Definition at line 268 of file videodevicepool.cpp.
|
static |
Returns pointer to a common instance of the VideoDevicePool.
- Returns
- Pointer to the VideoDevicePool object
Definition at line 56 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::setControlValue | ( | quint32 | ctrl_id, |
qint32 | value | ||
) |
Sets the value of a video-control.
- Parameters
-
ctrl_id ID of the video-control value The value that should be set. For boolean controls, the value must be 0 or 1. For menu-controls, the value must be the index of the option. For action-controls, the value is ignored.
- Returns
- The result-code, currently EXIT_SUCCESS or EXIT_FAILURE
Definition at line 358 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::setImageSize | ( | int | newwidth, |
int | newheight | ||
) |
Definition at line 470 of file videodevicepool.cpp.
|
protected |
Definition at line 743 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::size | ( | ) |
Returns the number of available video devices.
- Returns
- The number of available video devices
Definition at line 205 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::startCapturing | ( | ) |
Starts capturing from the currently selected video device.
- Returns
- The result-code, EXIT_SUCCESS or EXIT_FAILURE
Definition at line 373 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::stopCapturing | ( | ) |
Starts capturing from the currently selected video device.
- Returns
- The result-code, EXIT_SUCCESS or EXIT_FAILURE
Definition at line 388 of file videodevicepool.cpp.
int Kopete::AV::VideoDevicePool::width | ( | ) |
Definition at line 422 of file videodevicepool.cpp.
Member Data Documentation
|
protected |
Definition at line 92 of file videodevicepool.h.
|
protected |
Vector of pointers to the available video devices
Definition at line 93 of file videodevicepool.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.