kopete/libkopete
#include <kopetemessagehandler.h>
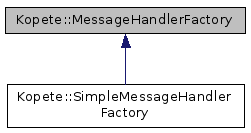
Public Types | |
typedef QLinkedList < MessageHandlerFactory * > | FactoryList |
enum | InboundStage { InStageStart = 0, InStageToSent = 2000, InStageToDesired = 5000, InStageFormat = 7000, InStageEnd = 10000 } |
enum | InternalStage { IntStageStart = 0, IntStageEnd = 10000 } |
enum | Offset { OffsetBefore = -90, OffsetVeryEarly = -60, OffsetEarly = -30, OffsetNormal = 0, OffsetLate = 30, OffsetVeryLate = 60, OffsetAfter = 90 } |
enum | OutboundStage { OutStageStart = 0, OutStageParse = 2000, OutStageToDesired = 4000, OutStageFormat = 6000, OutStageToSent = 8000, OutStageEnd = 10000 } |
enum | SpecialStage { StageDoNotCreate = -10000, StageStart = 0, StageEnd = 10000 } |
Public Member Functions | |
MessageHandlerFactory () | |
virtual | ~MessageHandlerFactory () |
virtual MessageHandler * | create (ChatSession *manager, Message::MessageDirection direction)=0 |
virtual int | filterPosition (ChatSession *manager, Message::MessageDirection direction)=0 |
Static Public Member Functions | |
static FactoryList | messageHandlerFactories () |
Detailed Description
A factory for creating MessageHandlers. Instantiate a class derived from MessageHandlerFactory in order to make your MessageHandler be automatically added to the list of handlers used when constructing handler chains.
- Note
- If you construct a handler for an Inbound chain, it may still be asked to process Outbound messages. This is because when a message is being sent it first passes through the Outbound chain to the protocol, then (when it has been delivered) it passes back through the Inbound chain to the chat window to be displayed.
Definition at line 109 of file kopetemessagehandler.h.
Member Typedef Documentation
typedef QLinkedList<MessageHandlerFactory*> Kopete::MessageHandlerFactory::FactoryList |
Definition at line 126 of file kopetemessagehandler.h.
Member Enumeration Documentation
Processing stages for handlers in inbound message handler chains.
Definition at line 153 of file kopetemessagehandler.h.
Processing stages for handlers in internal message handler chains.
Enumerator | |
---|---|
IntStageStart |
some component just created the message |
IntStageEnd |
message ready for display |
Definition at line 178 of file kopetemessagehandler.h.
Offsets within a processing stage.
Using these values allows finer control over where in a chain a message handler will be added. Add one of these values to values from the various Stage enumerations to form a filter position.
Enumerator | |
---|---|
OffsetBefore | |
OffsetVeryEarly | |
OffsetEarly | |
OffsetNormal | |
OffsetLate | |
OffsetVeryLate | |
OffsetAfter |
Definition at line 190 of file kopetemessagehandler.h.
Processing stages for handlers in outbound message handler chains.
Definition at line 165 of file kopetemessagehandler.h.
Special stages usable with any message direction.
Enumerator | |
---|---|
StageDoNotCreate |
do not create a filter for this stage |
StageStart |
start of processing |
StageEnd |
end of processing |
Definition at line 143 of file kopetemessagehandler.h.
Constructor & Destructor Documentation
Kopete::MessageHandlerFactory::MessageHandlerFactory | ( | ) |
Constructs a MessageHandlerFactory, and adds it to the list of factories considered when creating a MessageHandlerChain for a ChatSession.
- Note
- Since the factory is added to the list of possible factories before the object is finished being constructed, it is not safe to call any function from a derived class's constructor which may cause a MessageHandlerChain to be created.
Definition at line 88 of file kopetemessagehandler.cpp.
|
virtual |
Destroys the MessageHandlerFactory and removes it from the list of factories.
Definition at line 94 of file kopetemessagehandler.cpp.
Member Function Documentation
|
pure virtual |
Creates a message handler for a given manager in a given direction.
- Parameters
-
manager The manager whose message handler chain the message handler is for direction The direction of the chain that is being created.
- Returns
- the MessageHandler object to put in the chain, or 0 if none is needed.
Implemented in Kopete::SimpleMessageHandlerFactory.
|
pure virtual |
Returns the position in the message handler chain to put this factory's handlers.
- Parameters
-
manager The manager whose message handler chain the message handler is for direction The direction of the chain that is being created.
- Returns
- a member of the InboundStage, OutboundStage or InternalStage enumeration, as appropriate, optionally combined with a member of the Offset enumeration.
- Return values
-
StageDoNotCreate No filter should be created for this chain.
Implemented in Kopete::SimpleMessageHandlerFactory.
|
static |
- Returns
- the list of registered message handler factories
Definition at line 102 of file kopetemessagehandler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.