kopete/libkopete
#include <kopetepassword.h>
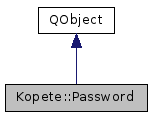
Public Types | |
enum | PasswordSource { FromConfigOrUser, FromUser } |
Public Slots | |
void | clear () |
void | set (const QString &pass=QString()) |
Public Member Functions | |
Password (const QString &configGroup, bool allowBlankPassword=false) | |
Password (const Password &other) | |
~Password () | |
bool | allowBlankPassword () |
QString | cachedValue () |
bool | isWrong () |
Password & | operator= (Password &other) |
bool | remembered () |
void | request (QObject *receiver, const char *slot, const QPixmap &image, const QString &prompt, PasswordSource source=FromConfigOrUser) |
void | requestWithoutPrompt (QObject *receiver, const char *slot) |
void | setWrong (bool bWrong=true) |
Static Public Member Functions | |
static int | preferredImageSize () |
Detailed Description
The Kopete::Password object is responsible for storing and retrieving a password for a plugin or account object.
If the KWallet is active, passwords will be stored in it, otherwise, they will be stored in the KConfig, in a slightly mangled form.
Definition at line 48 of file kopetepassword.h.
Member Enumeration Documentation
Type of password request to perform: FromConfigOrUser : get the password from the config file, or from the user if no password in config.
FromUser : always ask the user for a password (ie, if last password was wrong or you know the password has changed).
Enumerator | |
---|---|
FromConfigOrUser | |
FromUser |
Definition at line 98 of file kopetepassword.h.
Constructor & Destructor Documentation
|
explicit |
Create a new Kopete::Password object.
- Parameters
-
configGroup The configuration group to save passwords in. allowBlankPassword If this password is allowed to be blank name The name for this object
Definition at line 327 of file kopetepassword.cpp.
Kopete::Password::Password | ( | const Password & | other | ) |
Create a shallow copy of this object.
Definition at line 333 of file kopetepassword.cpp.
Kopete::Password::~Password | ( | ) |
Definition at line 338 of file kopetepassword.cpp.
Member Function Documentation
bool Kopete::Password::allowBlankPassword | ( | ) |
- Returns
- true if you are allowed to have a blank password
Definition at line 395 of file kopetepassword.cpp.
QString Kopete::Password::cachedValue | ( | ) |
When a password request succeeds, the password is cached.
This function returns the cached password, if there is one, or QString() if there is not.
Definition at line 428 of file kopetepassword.cpp.
|
slot |
Unconditionally clears the stored password.
Definition at line 448 of file kopetepassword.cpp.
bool Kopete::Password::isWrong | ( | ) |
Returns whether the password currently stored by this object is known to be incorrect.
This flag gets reset whenever the user enters a new password, and is expected to be set by the user of this class if it is detected that the password the user entered is wrong.
Definition at line 400 of file kopetepassword.cpp.
Kopete::Password & Kopete::Password::operator= | ( | Password & | other | ) |
Assignment operator for passwords: make this object represent a different password.
Definition at line 343 of file kopetepassword.cpp.
|
static |
Returns the preferred size for images passed to the retrieve and request functions.
Definition at line 390 of file kopetepassword.cpp.
bool Kopete::Password::remembered | ( | ) |
- Returns
- true if the password is remembered, false otherwise.
If it returns false, calling request() will pop up an Enter Password window.
Definition at line 454 of file kopetepassword.cpp.
void Kopete::Password::request | ( | QObject * | receiver, |
const char * | slot, | ||
const QPixmap & | image, | ||
const QString & | prompt, | ||
PasswordSource | source = FromConfigOrUser |
||
) |
Start an asynchronous call to get the password.
Causes a password entry dialog to appear if the password is not set. Triggers a provided slot when done, but not until after this function has returned (you don't need to worry about reentrancy or nested event loops).
- Parameters
-
receiver The object to notify when the password request finishes slot The slot on receiver to call at the end of the request. The signature of this function should be slot( const QString &password ). password will be the password if successful, or QString() if failed. image The icon to display in the dialog when asking for the password prompt The message to display to the user, asking for a password. Can be any Qt RichText string. source The source the password is taken from if a wrong or invalid password is entered or the password could not be found in the wallet
Definition at line 421 of file kopetepassword.cpp.
void Kopete::Password::requestWithoutPrompt | ( | QObject * | receiver, |
const char * | slot | ||
) |
Start an asynchronous password request without a prompt.
Starts an asynchronous password request. Does not pop up a password entry dialog if there is no password.
- See also
- request(QObject*,const char*,const QPixmap&,const QString&,bool,unsigned int) The password given to the provided slot will be NULL if no password could be retrieved for some reason, such as the user declining to open the wallet, or no password being found.
Definition at line 413 of file kopetepassword.cpp.
|
slot |
Set the password for this account.
- Parameters
-
pass If set to QString(), the password is forgotten unless you specified to allow blank passwords. Otherwise, sets the password to this value.
Note: this function is asynchronous; changes will not be instant.
Definition at line 433 of file kopetepassword.cpp.
void Kopete::Password::setWrong | ( | bool | bWrong = true | ) |
Flag the password as being incorrect.
- See also
- isWrong
Definition at line 405 of file kopetepassword.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.