krdc
#include <remoteview.h>
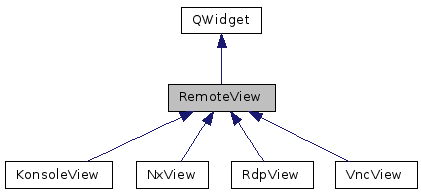
Public Types | |
enum | DotCursorState { CursorOn, CursorOff, CursorAuto } |
enum | ErrorCode { None = 0, Internal, Connection, Protocol, IO, Name, NoServer, ServerBlocked, Authentication } |
enum | Quality { Unknown, High, Medium, Low } |
enum | RemoteStatus { Connecting = 0, Authenticating = 1, Preparing = 2, Connected = 3, Disconnecting = -1, Disconnected = -2 } |
Public Slots | |
virtual void | enableScaling (bool scale) |
virtual void | keyEvent (QKeyEvent *event) |
virtual void | scaleResize (int w, int h) |
virtual void | setGrabAllKeys (bool grabAllKeys) |
virtual void | setViewOnly (bool viewOnly) |
virtual void | switchFullscreen (bool on) |
Signals | |
void | connected () |
void | disconnected () |
void | disconnectedError () |
void | errorMessage (const QString &title, const QString &message) |
void | framebufferSizeChanged (int w, int h) |
void | mouseStateChanged (int x, int y, int buttonMask) |
void | showingPasswordDialog (bool b) |
void | statusChanged (RemoteView::RemoteStatus s) |
Public Member Functions | |
virtual | ~RemoteView () |
virtual DotCursorState | dotCursorState () const |
virtual QSize | framebufferSize () |
virtual bool | grabAllKeys () |
virtual QString | host () |
virtual HostPreferences * | hostPreferences ()=0 |
virtual bool | isQuitting () |
virtual int | port () |
virtual bool | scaling () const |
virtual void | showDotCursor (DotCursorState state) |
virtual bool | start ()=0 |
virtual void | startQuitting () |
RemoteStatus | status () |
virtual bool | supportsLocalCursor () const |
virtual bool | supportsScaling () const |
virtual QPixmap | takeScreenshot () |
virtual void | updateConfiguration () |
KUrl | url () |
virtual bool | viewOnly () |
Protected Member Functions | |
RemoteView (QWidget *parent=0) | |
void | focusInEvent (QFocusEvent *event) |
void | focusOutEvent (QFocusEvent *event) |
QCursor | localDotCursor () const |
QString | readWalletPassword (bool fromUserNameOnly=false) |
void | saveWalletPassword (const QString &password, bool fromUserNameOnly=false) |
virtual void | setStatus (RemoteStatus s) |
Protected Attributes | |
DotCursorState | m_dotCursorState |
bool | m_grabAllKeys |
QString | m_host |
bool | m_keyboardIsGrabbed |
int | m_port |
bool | m_scale |
RemoteStatus | m_status |
KUrl | m_url |
bool | m_viewOnly |
KWallet::Wallet * | m_wallet |
Detailed Description
Generic widget that displays a remote framebuffer.
Implement this if you want to add another backend.
Things to take care of:
- The RemoteView is responsible for its size. In non-scaling mode, set the fixed size of the widget to the remote resolution. In scaling mode, set the maximum size to the remote size and minimum size to the smallest resolution that your scaler can handle.
- if you override mouseMoveEvent() you must ignore the QEvent, because the KRDC widget will need it for stuff like toolbar auto-hide and bump scrolling. If you use x11Event(), make sure that MotionNotify events will be forwarded.
Definition at line 59 of file remoteview.h.
Member Enumeration Documentation
Describes the state of a local cursor, if there is such a concept in the backend.
With local cursors, there are two cursors: the cursor on the local machine (client), and the cursor on the remote machine (server). Because there is usually some lag, some backends show both cursors simultanously. In the VNC backend the local cursor is a dot and the remote cursor is the 'real' cursor, usually an arrow.
Definition at line 84 of file remoteview.h.
Enumerator | |
---|---|
None | |
Internal | |
Connection | |
Protocol | |
IO | |
Name | |
NoServer | |
ServerBlocked | |
Authentication |
Definition at line 119 of file remoteview.h.
enum RemoteView::Quality |
Enumerator | |
---|---|
Unknown | |
High | |
Medium | |
Low |
Definition at line 67 of file remoteview.h.
State of the connection.
The state of the connection is returned by RemoteView::status().
Not every state transition is allowed. You are only allowed to transition a state to the following state, with three exceptions:
- You can move from every state directly to Disconnected
- You can move from every state except Disconnected to Disconnecting
- You can move from Disconnected to Connecting
RemoteView::setStatus() will follow this rules for you. (If you add/remove a state here, you must adapt it)
Enumerator | |
---|---|
Connecting | |
Authenticating | |
Preparing | |
Connected | |
Disconnecting | |
Disconnected |
Definition at line 108 of file remoteview.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 50 of file remoteview.cpp.
|
protected |
Definition at line 34 of file remoteview.cpp.
Member Function Documentation
|
signal |
Emitted when the view connected successfully.
|
signal |
Emitted when the view disconnected without error.
|
signal |
Emitted when the view disconnected with error.
|
virtual |
Returns the state of the local cursor.
The default implementation returns always CursorOff.
- Returns
- true if local cursors are supported/known
- See also
- showDotCursor()
- supportsLocalCursor()
Definition at line 170 of file remoteview.cpp.
|
virtualslot |
Called to enable or disable scaling.
Ignored if supportsScaling() is false. The default implementation does nothing.
- Parameters
-
s true to enable, false to disable.
- See also
- supportsScaling()
- scaling()
Reimplemented in VncView.
Definition at line 180 of file remoteview.cpp.
|
signal |
Emitted when the view has a specific error.
|
protected |
Definition at line 263 of file remoteview.cpp.
|
protected |
Definition at line 273 of file remoteview.cpp.
|
virtual |
Returns the resolution of the remote framebuffer.
It should return a null QSize when the size is not known. The backend must also emit a framebufferSizeChanged() when the size of the framebuffer becomes available for the first time or the size changed.
- Returns
- the remote framebuffer size, a null QSize if unknown
Reimplemented in RdpView, NxView, VncView, and KonsoleView.
Definition at line 106 of file remoteview.cpp.
|
signal |
Emitted when the size of the remote screen changes.
Also called when the size is known for the first time.
- Parameters
-
x the width of the screen y the height of the screen
|
virtual |
Checks whether grabbing all possible keys is enabled.
Definition at line 143 of file remoteview.cpp.
|
virtual |
- Returns
- the host the view is connected to
Definition at line 101 of file remoteview.cpp.
|
pure virtual |
Returns the current host preferences of this view.
Implemented in RdpView, VncView, NxView, and KonsoleView.
|
virtual |
Checks whether the view is currently quitting.
- Returns
- true if it is quitting
- See also
- startQuitting()
- setStatus()
Reimplemented in RdpView, VncView, KonsoleView, and NxView.
Definition at line 115 of file remoteview.cpp.
|
virtualslot |
Sends a QKeyEvent to the remote server.
- Parameters
-
event the key to send
Definition at line 129 of file remoteview.cpp.
|
protected |
Definition at line 250 of file remoteview.cpp.
|
signal |
Emitted when the mouse on the remote side has been moved.
- Parameters
-
x the new x coordinate y the new y coordinate buttonMask the mask of mouse buttons (bit 0 for first mouse button, 1 for second button etc)a
|
virtual |
- Returns
- the port the view is connected to
Definition at line 120 of file remoteview.cpp.
|
protected |
Definition at line 199 of file remoteview.cpp.
|
protected |
Definition at line 235 of file remoteview.cpp.
|
virtualslot |
Called when the visible place changed so remote view can resize itself.
Definition at line 189 of file remoteview.cpp.
|
virtual |
Checks whether the widget is in scale mode.
The default implementation always returns false.
- Returns
- true if scaling is activated. Must always be false if supportsScaling() returns false
- See also
- supportsScaling()
Definition at line 175 of file remoteview.cpp.
|
virtualslot |
Enables/disables grabbing all possible keys.
- Parameters
-
grabAllKeys true to enable, false to disable. Default is false.
- See also
- grabAllKeys()
Reimplemented in RdpView, and NxView.
Definition at line 148 of file remoteview.cpp.
|
protectedvirtual |
Set the status of the connection.
Emits a statusChanged() signal. Note that the states need to be set in a certain order, see Status. setStatus() will try to do this transition automatically, so if you are in Connecting and call setStatus(Preparing), setStatus() will emit a Authenticating and then Preparing. If you transition backwards, it will emit a Disconnected before doing the transition.
- Parameters
-
s the new status
Definition at line 62 of file remoteview.cpp.
|
virtualslot |
Enables/disables the view-only mode.
Ignored if supportsScaling() is false. The default implementation does nothing.
- Parameters
-
viewOnly true to enable, false to disable.
- See also
- supportsScaling()
- viewOnly()
Reimplemented in VncView.
Definition at line 138 of file remoteview.cpp.
|
virtual |
Sets the state of the dot cursor, if supported by the backend.
The default implementation does nothing.
- Parameters
-
state the new state (CursorOn, CursorOff or CursorAuto)
Reimplemented in VncView.
Definition at line 165 of file remoteview.cpp.
|
signal |
Emitted when the password dialog is shown or hidden.
- Parameters
-
b true when the dialog is shown, false when it has been hidden
|
pure virtual |
Initialize the view (for example by showing configuration dialogs to the user) and start connecting.
Should not block without running the event loop (so displaying a dialog is ok). When the view starts connecting the application must call setStatus() with the status Connecting.
- Returns
- true if successful (so far), false otherwise
Implemented in RdpView, VncView, KonsoleView, and NxView.
|
virtual |
Initiate the disconnection.
This doesn't need to happen immediately. The call must not block.
- See also
- isQuitting()
Reimplemented in RdpView, VncView, and NxView.
Definition at line 111 of file remoteview.cpp.
RemoteView::RemoteStatus RemoteView::status | ( | ) |
Returns the current status of the connection.
- Returns
- the status of the connection
- See also
- setStatus()
Definition at line 57 of file remoteview.cpp.
|
signal |
Emitted when the status of the view changed.
- Parameters
-
s the new status
|
virtual |
Checks whether the backend supports the concept of local cursors.
The default implementation returns false.
- Returns
- true if local cursors are supported/known
Reimplemented in VncView.
Definition at line 96 of file remoteview.cpp.
|
virtual |
Checks whether the backend supports scaling.
The default implementation returns false.
- Returns
- true if scaling is supported
- See also
- scaling()
Reimplemented in VncView.
Definition at line 91 of file remoteview.cpp.
|
virtualslot |
Called to let the backend know it when we switch from/to fullscreen.
- Parameters
-
on true when switching to fullscreen, false when switching from fullscreen.
Definition at line 185 of file remoteview.cpp.
|
virtual |
- Returns
- screenshot of the view
Reimplemented in RdpView.
Definition at line 160 of file remoteview.cpp.
|
virtual |
Called when the configuration is changed.
The default implementation does nothing.
Reimplemented in VncView.
Definition at line 125 of file remoteview.cpp.
KUrl RemoteView::url | ( | ) |
- Returns
- the current url
Definition at line 193 of file remoteview.cpp.
|
virtual |
Checks whether the view is in view-only mode.
This means that all input is ignored.
Definition at line 133 of file remoteview.cpp.
Member Data Documentation
|
protected |
Definition at line 412 of file remoteview.h.
|
protected |
Definition at line 401 of file remoteview.h.
|
protected |
Definition at line 398 of file remoteview.h.
|
protected |
Definition at line 403 of file remoteview.h.
|
protected |
Definition at line 399 of file remoteview.h.
|
protected |
Definition at line 402 of file remoteview.h.
|
protected |
The status of the remote view.
Definition at line 380 of file remoteview.h.
|
protected |
Definition at line 404 of file remoteview.h.
|
protected |
Definition at line 400 of file remoteview.h.
|
protected |
Definition at line 409 of file remoteview.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:54:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.