libkleo
stl_util.h File Reference
#include <boost/range.hpp>
#include <boost/iterator/filter_iterator.hpp>
#include <boost/iterator/transform_iterator.hpp>
#include <boost/call_traits.hpp>
#include <boost/version.hpp>
#include <algorithm>
#include <numeric>
#include <utility>
#include <iterator>
#include <functional>
Include dependency graph for stl_util.h:

This graph shows which files directly or indirectly include this file:
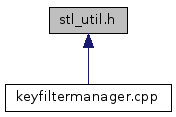
Go to the source code of this file.
Classes | |
struct | kdtools::identity |
struct | kdtools::nodelete |
struct | kdtools::select1st< Pair > |
struct | kdtools::select1st< std::pair< U, V > > |
struct | kdtools::select2nd< Pair > |
struct | kdtools::select2nd< std::pair< U, V > > |
Namespaces | |
kdtools | |
Functions | |
template<typename Value , typename InputIterator , typename UnaryPredicate > | |
Value | kdtools::accumulate_if (InputIterator first, InputIterator last, UnaryPredicate filter, const Value &value=Value()) |
template<typename Value , typename InputIterator , typename UnaryPredicate , typename BinaryOperation > | |
Value | kdtools::accumulate_if (InputIterator first, InputIterator last, UnaryPredicate filter, const Value &value, BinaryOperation op) |
template<typename Value , typename InputIterator , typename UnaryFunction > | |
Value | kdtools::accumulate_transform (InputIterator first, InputIterator last, UnaryFunction map, const Value &value=Value()) |
template<typename Value , typename InputIterator , typename UnaryFunction , typename BinaryOperation > | |
Value | kdtools::accumulate_transform (InputIterator first, InputIterator last, UnaryFunction map, const Value &value, BinaryOperation op) |
template<typename Value , typename InputIterator , typename UnaryFunction , typename UnaryPredicate > | |
Value | kdtools::accumulate_transform_if (InputIterator first, InputIterator last, UnaryFunction map, UnaryPredicate pred, const Value &value=Value()) |
template<typename Value , typename InputIterator , typename UnaryFunction , typename UnaryPredicate , typename BinaryOperation > | |
Value | kdtools::accumulate_transform_if (InputIterator first, InputIterator last, UnaryFunction map, UnaryPredicate filter, const Value &value, BinaryOperation op) |
template<typename InputIterator > | |
bool | kdtools::all (InputIterator first, InputIterator last) |
template<typename InputIterator , typename UnaryPredicate > | |
bool | kdtools::all (InputIterator first, InputIterator last, UnaryPredicate pred) |
template<typename C > | |
bool | kdtools::all (const C &c) |
template<typename C , typename P > | |
bool | kdtools::all (const C &c, P p) |
template<typename InputIterator > | |
bool | kdtools::any (InputIterator first, InputIterator last) |
template<typename InputIterator , typename UnaryPredicate > | |
bool | kdtools::any (InputIterator first, InputIterator last, UnaryPredicate pred) |
template<typename C > | |
bool | kdtools::any (const C &c) |
template<typename C , typename P > | |
bool | kdtools::any (const C &c, P p) |
template<typename InputIterator , typename OutputIterator > | |
OutputIterator | kdtools::copy_1st (InputIterator first, InputIterator last, OutputIterator dest) |
template<typename InputIterator , typename OutputIterator , typename Predicate > | |
OutputIterator | kdtools::copy_1st_if (InputIterator first, InputIterator last, OutputIterator dest, Predicate pred) |
template<typename InputIterator , typename OutputIterator > | |
OutputIterator | kdtools::copy_2nd (InputIterator first, InputIterator last, OutputIterator dest) |
template<typename InputIterator , typename OutputIterator , typename Predicate > | |
OutputIterator | kdtools::copy_2nd_if (InputIterator first, InputIterator last, OutputIterator dest, Predicate pred) |
template<typename InputIterator , typename OutputIterator , typename UnaryPredicate > | |
OutputIterator | kdtools::copy_if (InputIterator first, InputIterator last, OutputIterator dest, UnaryPredicate pred) |
template<typename InputIterator , typename BinaryOperation > | |
BinaryOperation | kdtools::for_each_adjacent_pair (InputIterator first, InputIterator last, BinaryOperation op) |
template<typename C , typename B > | |
B | kdtools::for_each_adjacent_pair (const C &c, B b) |
template<typename C , typename B > | |
B | kdtools::for_each_adjacent_pair (C &c, B b) |
template<typename ForwardIterator , typename UnaryPredicate , typename UnaryFunction > | |
UnaryFunction | kdtools::for_each_if (ForwardIterator first, ForwardIterator last, UnaryPredicate pred, UnaryFunction func) |
template<typename C , typename P , typename F > | |
P | kdtools::for_each_if (const C &c, P p, F f) |
template<typename C , typename P , typename F > | |
P | kdtools::for_each_if (C &c, P p, F f) |
template<typename InputIterator > | |
bool | kdtools::none_of (InputIterator first, InputIterator last) |
template<typename InputIterator , typename UnaryPredicate > | |
bool | kdtools::none_of (InputIterator first, InputIterator last, UnaryPredicate pred) |
template<typename C > | |
bool | kdtools::none_of (const C &c) |
template<typename C , typename P > | |
bool | kdtools::none_of (const C &c, P p) |
template<typename InputIterator , typename OutputIterator1 , typename OutputIterator2 , typename UnaryPredicate > | |
std::pair< OutputIterator1, OutputIterator2 > | kdtools::separate_if (InputIterator first, InputIterator last, OutputIterator1 dest1, OutputIterator2 dest2, UnaryPredicate pred) |
template<typename ForwardIterator , typename ForwardIterator2 , typename BinaryPredicate > | |
bool | kdtools::set_intersects (ForwardIterator first1, ForwardIterator last1, ForwardIterator2 first2, ForwardIterator2 last2, BinaryPredicate pred) |
template<typename C > | |
void | kdtools::sort (C &c) |
template<typename C , typename P > | |
void | kdtools::sort (C &c, P p) |
template<typename C > | |
C | kdtools::sorted (const C &c) |
template<typename C , typename P > | |
C | kdtools::sorted (const C &c, P p) |
template<typename OutputIterator , typename InputIterator , typename UnaryFunction > | |
OutputIterator | kdtools::transform_1st (InputIterator first, InputIterator last, OutputIterator dest, UnaryFunction func) |
template<typename OutputIterator , typename InputIterator , typename UnaryFunction > | |
OutputIterator | kdtools::transform_2nd (InputIterator first, InputIterator last, OutputIterator dest, UnaryFunction func) |
template<typename OutputIterator , typename InputIterator , typename UnaryFunction , typename UnaryPredicate > | |
OutputIterator | kdtools::transform_if (InputIterator first, InputIterator last, OutputIterator dest, UnaryPredicate pred, UnaryFunction filter) |
template<typename ForwardIterator , typename ForwardIterator2 , typename OutputIterator , typename BinaryPredicate > | |
OutputIterator | kdtools::set_intersection (ForwardIterator first1, ForwardIterator last1, ForwardIterator2 first2, ForwardIterator2 last2, OutputIterator result) |
template<typename ForwardIterator , typename ForwardIterator2 , typename OutputIterator , typename BinaryPredicate > | |
OutputIterator | kdtools::set_intersection (ForwardIterator first1, ForwardIterator last1, ForwardIterator2 first2, ForwardIterator2 last2, OutputIterator result, BinaryPredicate pred) |
template<typename C , typename V > | |
boost::range_iterator< C >::type | kdtools::find (C &c, const V &v) |
template<typename C , typename V > | |
boost::range_const_iterator< C > ::type | kdtools::find (const C &c, const V &v) |
template<typename C , typename P > | |
boost::range_iterator< C >::type | kdtools::find_if (C &c, P p) |
template<typename C , typename P > | |
boost::range_const_iterator< C > ::type | kdtools::find_if (const C &c, P p) |
template<typename C , typename V > | |
bool | kdtools::contains (const C &c, const V &v) |
template<typename C , typename P > | |
bool | kdtools::contains_if (const C &c, P p) |
template<typename C , typename V > | |
bool | kdtools::binary_search (const C &c, const V &v) |
template<typename C , typename V > | |
size_t | kdtools::count (const C &c, const V &v) |
template<typename C , typename P > | |
size_t | kdtools::count_if (const C &c, P p) |
template<typename O , typename I , typename P > | |
O | kdtools::transform (const I &i, P p) |
template<typename I , typename OutputIterator , typename P > | |
OutputIterator | kdtools::transform (const I &i, OutputIterator out, P p) |
template<typename O , typename I , typename P , typename F > | |
O | kdtools::transform_if (const I &i, P p, F f) |
template<typename V , typename I , typename F > | |
V | kdtools::accumulate_if (const I &i, F f, V v=V()) |
template<typename V , typename I , typename F , typename B > | |
V | kdtools::accumulate_if (const I &i, F f, V v, B b) |
template<typename V , typename I , typename F > | |
V | kdtools::accumulate_transform (const I &i, F f, V v=V()) |
template<typename V , typename I , typename F , typename B > | |
V | kdtools::accumulate_transform (const I &i, F f, V v, B b) |
template<typename V , typename I , typename F , typename P > | |
V | kdtools::accumulate_transform_if (const I &i, F f, P p, V v=V()) |
template<typename V , typename I , typename F , typename P , typename B > | |
V | kdtools::accumulate_transform_if (const I &i, F f, P p, V v, B b) |
template<typename O , typename I > | |
O | kdtools::copy (const I &i) |
template<typename O , typename I , typename P > | |
O | kdtools::copy_if (const I &i, P p) |
template<typename I , typename P > | |
P | kdtools::for_each (const I &i, P p) |
template<typename I , typename P > | |
P | kdtools::for_each (I &i, P p) |
template<typename C1 , typename C2 > | |
bool | kdtools::equal (const C1 &c1, const C2 &c2) |
template<typename C1 , typename C2 , typename P > | |
bool | kdtools::equal (const C1 &c1, const C2 &c2, P p) |
template<typename C , typename O1 , typename O2 , typename P > | |
std::pair< O1, O2 > | kdtools::separate_if (const C &c, O1 o1, O2 o2, P p) |
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:57:49 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:57:49 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.