kabc
#include <resource.h>
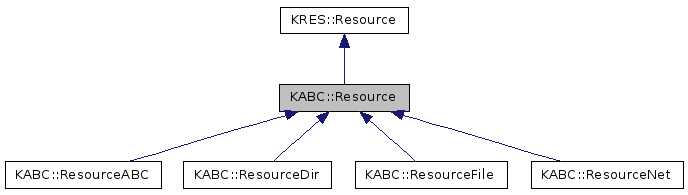
Classes | |
class | ConstIterator |
Signals | |
void | loadingError (Resource *resource, const QString &msg) |
void | loadingFinished (Resource *resource) |
void | savingError (Resource *resource, const QString &msg) |
void | savingFinished (Resource *resource) |
Public Member Functions | |
Resource () | |
Resource (const KConfigGroup &group) | |
virtual | ~Resource () |
AddressBook * | addressBook () |
virtual QStringList | allDistributionListNames () const |
virtual QList< DistributionList * > | allDistributionLists () |
virtual bool | asyncLoad () |
virtual bool | asyncSave (Ticket *ticket) |
virtual ConstIterator | begin () const |
virtual Iterator | begin () |
virtual void | clear () |
ConstIterator | constBegin () const |
ConstIterator | constEnd () const |
virtual ConstIterator | end () const |
virtual Iterator | end () |
virtual Addressee::List | findByCategory (const QString &category) |
virtual Addressee::List | findByEmail (const QString &email) |
virtual Addressee::List | findByName (const QString &name) |
virtual Addressee | findByUid (const QString &uid) |
virtual DistributionList * | findDistributionListByIdentifier (const QString &identifier) |
virtual DistributionList * | findDistributionListByName (const QString &name, Qt::CaseSensitivity caseSensitivity=Qt::CaseSensitive) |
virtual void | insertAddressee (const Addressee &addr) |
virtual void | insertDistributionList (DistributionList *list) |
virtual bool | load ()=0 |
virtual void | releaseSaveTicket (Ticket *ticket)=0 |
virtual void | removeAddressee (const Addressee &addr) |
virtual void | removeDistributionList (DistributionList *list) |
virtual Ticket * | requestSaveTicket ()=0 |
virtual bool | save (Ticket *ticket)=0 |
void | setAddressBook (AddressBook *addr) |
virtual void | writeConfig (KConfigGroup &group) |
![]() | |
Resource (const KConfigGroup &group) | |
void | close () |
virtual void | dump () const |
QString | identifier () const |
bool | isActive () const |
bool | isOpen () const |
bool | open () |
virtual bool | readOnly () const |
virtual QString | resourceName () const |
void | setActive (bool active) |
void | setIdentifier (const QString &identifier) |
virtual void | setReadOnly (bool value) |
virtual void | setResourceName (const QString &name) |
void | setType (const QString &type) |
QString | type () const |
Protected Member Functions | |
Ticket * | createTicket (Resource *) |
![]() | |
virtual void | doClose () |
virtual bool | doOpen () |
Protected Attributes | |
Addressee::Map | mAddrMap |
DistributionListMap | mDistListMap |
Detailed Description
Definition at line 64 of file resource.h.
Constructor & Destructor Documentation
Resource::Resource | ( | ) |
Default constructor.
Definition at line 218 of file resource.cpp.
Resource::Resource | ( | const KConfigGroup & | group | ) |
Constructor.
- Parameters
-
group The configuration group where the derived classes can read out their settings.
Definition at line 223 of file resource.cpp.
|
virtual |
Member Function Documentation
AddressBook * Resource::addressBook | ( | ) |
Returns a pointer to the addressbook.
Definition at line 274 of file resource.cpp.
|
virtual |
Returns a list of names of all distribution lists of this resource.
Convenience function, equal to iterate over the list returned by allDistributionLists()
Definition at line 420 of file resource.cpp.
|
virtual |
Returns a list of all distribution lists of this resource.
Definition at line 415 of file resource.cpp.
|
virtual |
Loads all addressees asyncronously.
You have to make sure that either the loadingFinished() or loadingError() signal is emitted from within this function.
The default implementation simply calls the synchronous load.
- Returns
- Whether the synchronous part of loading was successfully.
Reimplemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
Definition at line 433 of file resource.cpp.
|
virtual |
Saves all addressees asynchronously.
You have to make sure that either the savingFinished() or savingError() signal is emitted from within this function.
The default implementation simply calls the synchronous save.
- Parameters
-
ticket You have to release the ticket later with releaseSaveTicket() explicitly.
- Returns
- Whether the saving was successfully.
Reimplemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
Definition at line 445 of file resource.cpp.
|
virtual |
Returns an iterator pointing to the first addressee in the resource.
This iterator equals end() if the resource is empty.
Definition at line 242 of file resource.cpp.
|
virtual |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 234 of file resource.cpp.
|
virtual |
Removes all addressees and distribution lists from the resource.
Definition at line 354 of file resource.cpp.
Factory method, just creates and returns a new Ticket for the given resource.
Needed by subclasses since the constructor of Ticket is private and only this base class is a friend, effectively limiting "new Ticket(this)" to resource implementations.
Definition at line 279 of file resource.cpp.
|
virtual |
Returns an iterator pointing to the last addressee in the resource.
This iterator equals begin() if the resource is empty.
Definition at line 257 of file resource.cpp.
|
virtual |
This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 249 of file resource.cpp.
|
virtual |
Searches all addressees which belongs to the specified category.
- Parameters
-
category The category you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 339 of file resource.cpp.
|
virtual |
Searches all addressees which match the specified email address.
- Parameters
-
email The email address you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 320 of file resource.cpp.
|
virtual |
Searches all addressees which match the specified name.
- Parameters
-
name The name you are looking for.
- Returns
- A list of all matching addressees.
Definition at line 305 of file resource.cpp.
|
virtual |
Searches an addressee with the specified unique identifier.
- Parameters
-
uid The unique identifier you are looking for.
- Returns
- The addressee with the specified unique identifier or an empty addressee.
Definition at line 294 of file resource.cpp.
|
virtual |
Returns a distribution list for the given identifier
or 0
.
- Parameters
-
identifier The ID of the list for look for.
Definition at line 385 of file resource.cpp.
|
virtual |
Returns a distribution list with the given name
or 0
.
- Parameters
-
name The localized name of the list for look for. caseSensitivity Whether to do string matching case sensitive or case insensitive. Default is Qt::CaseSensitive
Definition at line 390 of file resource.cpp.
|
virtual |
Insert an addressee into the resource.
- Parameters
-
addr The addressee to add
Definition at line 284 of file resource.cpp.
|
virtual |
Adds a distribution list
into this resource.
- Parameters
-
list The list to insert.
Definition at line 366 of file resource.cpp.
|
pure virtual |
Loads all addressees synchronously.
- Returns
- Whether the loading was successfully.
Implemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
|
signal |
This signal is emitted when an error occurred during loading the addressees from the backend to the internal cache.
- Parameters
-
resource The pointer to the resource which emitted this signal. msg A translated error message.
|
signal |
This signal is emitted when the resource has finished the loading of all addressees from the backend to the internal cache.
- Parameters
-
resource The pointer to the resource which emitted this signal.
|
pure virtual |
Releases the ticket previousely requested with requestSaveTicket().
The resource has to remove its locks in this function. This function is also responsible for deleting the ticket.
- Parameters
-
ticket the save ticket acquired with requestSaveTicket()
Implemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
|
virtual |
Removes an addressee from resource.
- Parameters
-
addr The addressee to remove
Reimplemented in KABC::ResourceFile, and KABC::ResourceDir.
Definition at line 289 of file resource.cpp.
|
virtual |
Removes a distribution list
from this resource.
- Parameters
-
list The list to remove.
Definition at line 373 of file resource.cpp.
|
pure virtual |
Request a ticket, you have to pass through save() to allow locking.
The resource has to create its locks in this function.
Implemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
|
pure virtual |
Saves all addressees synchronously.
- Parameters
-
ticket You have to release the ticket later with releaseSaveTicket() explicitly.
- Returns
- Whether the saving was successfully.
Implemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
|
signal |
This signal is emitted when an error occurred during saving the addressees from the internal cache to the backend.
- Parameters
-
resource The pointer to the resource which emitted this signal. msg A translated error message.
|
signal |
This signal is emitted when the resource has finished the saving of all addressees from the internal cache to the backend.
- Parameters
-
resource The pointer to the resource which emitted this signal.
void Resource::setAddressBook | ( | AddressBook * | addr | ) |
Sets the address book of the resource.
- Parameters
-
addr The address book to use
Definition at line 269 of file resource.cpp.
|
virtual |
Writes the resource specific config to file.
- Parameters
-
group The config section to write into
Reimplemented from KRES::Resource.
Reimplemented in KABC::ResourceFile, KABC::ResourceNet, and KABC::ResourceDir.
Definition at line 264 of file resource.cpp.
Member Data Documentation
|
protected |
A mapping from KABC UIDs to the respective addressee.
Definition at line 527 of file resource.h.
|
protected |
A mapping from unique identifiers to the respective distribution list.
Definition at line 532 of file resource.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:01:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.