KBlog Client Library
#include <gdata.h>
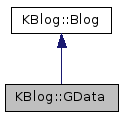
Signals | |
void | createdComment (const KBlog::BlogPost *post, const KBlog::BlogComment *comment) |
void | fetchedProfileId (const QString &profileId) |
void | listedAllComments (const QList< KBlog::BlogComment > &commentsList) |
void | listedBlogs (const QList< QMap< QString, QString > > &blogsList) |
void | listedComments (KBlog::BlogPost *post, const QList< KBlog::BlogComment > &comments) |
void | removedComment (const KBlog::BlogPost *post, const KBlog::BlogComment *comment) |
![]() | |
void | createdPost (KBlog::BlogPost *post) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | errorComment (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | errorMedia (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | errorPost (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post) |
void | fetchedPost (KBlog::BlogPost *post) |
void | listedRecentPosts (const QList< KBlog::BlogPost > &posts) |
void | modifiedPost (KBlog::BlogPost *post) |
void | removedPost (KBlog::BlogPost *post) |
Public Member Functions | |
GData (const KUrl &server, QObject *parent=0) | |
~GData () | |
virtual void | createComment (KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | createPost (KBlog::BlogPost *post) |
void | fetchPost (KBlog::BlogPost *post) |
void | fetchProfileId () |
QString | fullName () const |
QString | interfaceName () const |
virtual void | listAllComments () |
virtual void | listBlogs () |
virtual void | listComments (KBlog::BlogPost *post) |
void | listRecentPosts (int number) |
virtual void | listRecentPosts (const QStringList &label=QStringList(), int number=0, const KDateTime &upMinTime=KDateTime(), const KDateTime &upMaxTime=KDateTime(), const KDateTime &pubMinTime=KDateTime(), const KDateTime &pubMaxTime=KDateTime()) |
void | modifyPost (KBlog::BlogPost *post) |
QString | profileId () const |
virtual void | removeComment (KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | removePost (KBlog::BlogPost *post) |
virtual void | setFullName (const QString &fullName) |
virtual void | setProfileId (const QString &pid) |
![]() | |
Blog (const KUrl &server, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
virtual | ~Blog () |
QString | blogId () const |
QString | password () const |
virtual void | setBlogId (const QString &blogId) |
virtual void | setPassword (const QString &password) |
virtual void | setTimeZone (const KTimeZone &timeZone) |
virtual void | setUrl (const KUrl &url) |
void | setUserAgent (const QString &applicationName, const QString &applicationVersion) |
virtual void | setUsername (const QString &username) |
KTimeZone | timeZone () |
KUrl | url () const |
QString | userAgent () const |
QString | username () const |
Protected Member Functions | |
GData (const KUrl &server, GDataPrivate &dd, QObject *parent=0) | |
![]() | |
Blog (const KUrl &server, BlogPrivate &dd, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
Additional Inherited Members | |
![]() | |
enum | ErrorType { XmlRpc, Atom, ParsingError, AuthenticationError, NotSupported, Other } |
![]() | |
BlogPrivate *const | d_ptr |
Detailed Description
A class that can be used for access to GData blogs.
The new blogspot.com accounts ( August 2007 ) exclusively support GData API which is a standard based on Atom API. Compared to Blogger 1.0, which is based on Xml-Rpc and less secure, it adds new functionality like titles and comments.
Constructor & Destructor Documentation
|
explicit |
|
protected |
Overloaded for private inheritance handling.
Member Function Documentation
|
virtual |
Create a comment on the server.
- Parameters
-
post This is the post with its id set correctly. comment This is the comment to create.
- See also
- BlogPost::setPostId( const QString& )
- createdComment( KBlog::BlogPost*, KBlog::BlogComment* )
|
signal |
This signal is emitted when a comment has been created on the blogging server.
- Parameters
-
post This is the corresponding post. comment This is the created comment.
|
virtual |
|
signal |
This signal is emitted when the profile id has been fetched.
- Parameters
-
profileId This is the fetched id. On error it is QString()
- See also
- fetchProfileId()
|
virtual |
Fetch the Post with a specific id.
- Parameters
-
post This is the post with its id set correctly.
Implements KBlog::Blog.
void GData::fetchProfileId | ( | ) |
Get information about the profile from the blog.
Sets the profileId automatically for the blog it is called from.
QString GData::fullName | ( | ) | const |
Returns the full name of user of the blog.
- See also
- setFullName()
|
virtual |
Returns the of the inherited object.
Implements KBlog::Blog.
|
virtual |
List the all comments available for this authentication on the server.
- See also
- void listedAllComments( const QList<KBlog::BlogComment>& )
|
virtual |
List the blogs available for this authentication on the server.
- See also
- void listedBlogs( const QList<QMap<QString,QString>>& )
|
virtual |
List the comments available for this post on the server.
- Parameters
-
post The post, which posts should be listed.
- See also
- void listedComments( KBlog::BlogPost*, const QList<KBlog::BlogComment>& )
|
signal |
This signal is emitted when a list of all comments has been fetched from the blogging server.
- Parameters
-
commentsList The list of comments.
- See also
- listAllComments()
|
signal |
This signal is emitted when a list of blogs has been fetched from the blogging server.
- Parameters
-
blogsList The list of blogs.
- See also
- listBlogs()
|
signal |
This signal is emitted when a list of comments has been fetched from the blogging server.
- Parameters
-
post This is the corresponding post. comments The list of comments.
- See also
- listComments( KBlog::BlogPost* )
|
virtual |
List recent posts on the server.
The status of the posts will be Fetched.
- Parameters
-
number The number of posts to fetch. The order is newest first.
- See also
- void listedPosts( const QList<KBlog::BlogPost>& )
- void fetchPost( KBlog::BlogPost* )
- BlogPost::Status
Implements KBlog::Blog.
|
virtual |
List recent posts on the server depending on meta information about the post.
- Parameters
-
label The lables of posts to fetch. number The number of posts to fetch. The order is newest first. upMinTime The oldest upload time of the posts to fetch. upMaxTime The newest upload time of the posts to fetch. pubMinTime The oldest publication time of the posts to fetch. pubMaxTime The newest publication time of the posts to fetch.
- See also
- void listedPosts( const QList<KBlog::BlogPost>& )
- void fetchPost( KBlog::BlogPost* )
|
virtual |
Modify a post on server.
- Parameters
-
post This is used to send the modified post including the correct id.
Implements KBlog::Blog.
QString GData::profileId | ( | ) | const |
Returns the profile id of the blog.
This is used for rss paths internally.
- Returns
- The profile id.
- See also
- setProfileId( const QString& )
|
virtual |
Remove a comment from the server.
- Parameters
-
post This is the post with its id set correctly. comment This is the comment to remove.
- See also
- BlogPost::setPostId( const QString& )
- removedComment( KBlog::BlogPost*, KBlog::BlogComment* )
|
signal |
This signal is emitted when a comment has been removed from the blogging server.
- Parameters
-
post This is the corresponding post. comment This is the removed comment.
|
virtual |
Remove a post from the server.
- Parameters
-
post This is the post with its id set correctly.
Implements KBlog::Blog.
|
virtual |
Sets the user's name for the blog.
Username is only the E-Mail address of the user. This is used in createPost and modifyPost.
- Parameters
-
fullName is a QString containing the blog username.
- See also
- username()
- createPost( KBlog::BlogPost* )
- modifiyPost( KBlog::BlogPost* )
|
virtual |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:56 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.