KBlog Client Library
#include <wordpressbuggy.h>
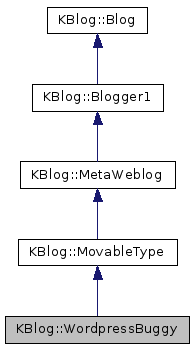
Public Member Functions | |
WordpressBuggy (const KUrl &server, QObject *parent=0) | |
virtual | ~WordpressBuggy () |
void | createPost (KBlog::BlogPost *post) |
QString | interfaceName () const |
void | modifyPost (KBlog::BlogPost *post) |
![]() | |
MovableType (const KUrl &server, QObject *parent=0) | |
virtual | ~MovableType () |
void | fetchPost (KBlog::BlogPost *post) |
void | listRecentPosts (int number) |
virtual void | listTrackBackPings (KBlog::BlogPost *post) |
![]() | |
MetaWeblog (const KUrl &server, QObject *parent=0) | |
virtual | ~MetaWeblog () |
virtual void | createMedia (KBlog::BlogMedia *media) |
virtual void | listCategories () |
![]() | |
Blogger1 (const KUrl &server, QObject *parent=0) | |
virtual | ~Blogger1 () |
virtual void | fetchUserInfo () |
virtual void | listBlogs () |
void | removePost (KBlog::BlogPost *post) |
void | setUrl (const KUrl &server) |
![]() | |
Blog (const KUrl &server, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
virtual | ~Blog () |
QString | blogId () const |
QString | password () const |
virtual void | setBlogId (const QString &blogId) |
virtual void | setPassword (const QString &password) |
virtual void | setTimeZone (const KTimeZone &timeZone) |
void | setUserAgent (const QString &applicationName, const QString &applicationVersion) |
virtual void | setUsername (const QString &username) |
KTimeZone | timeZone () |
KUrl | url () const |
QString | userAgent () const |
QString | username () const |
Protected Member Functions | |
WordpressBuggy (const KUrl &server, WordpressBuggyPrivate &dd, QObject *parent=0) | |
![]() | |
MovableType (const KUrl &server, MovableTypePrivate &dd, QObject *parent=0) | |
![]() | |
MetaWeblog (const KUrl &server, MetaWeblogPrivate &dd, QObject *parent=0) | |
![]() | |
Blogger1 (const KUrl &server, Blogger1Private &dd, QObject *parent=0) | |
![]() | |
Blog (const KUrl &server, BlogPrivate &dd, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
Additional Inherited Members | |
![]() | |
enum | ErrorType { XmlRpc, Atom, ParsingError, AuthenticationError, NotSupported, Other } |
![]() | |
void | listedTrackBackPings (KBlog::BlogPost *post, const QList< QMap< QString, QString > > &pings) |
![]() | |
void | createdMedia (KBlog::BlogMedia *media) |
void | listedCategories (const QList< QMap< QString, QString > > &categories) |
![]() | |
void | fetchedUserInfo (const QMap< QString, QString > &userInfo) |
void | listedBlogs (const QList< QMap< QString, QString > > &blogsList) |
![]() | |
void | createdPost (KBlog::BlogPost *post) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | errorComment (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | errorMedia (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | errorPost (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post) |
void | fetchedPost (KBlog::BlogPost *post) |
void | listedRecentPosts (const QList< KBlog::BlogPost > &posts) |
void | modifiedPost (KBlog::BlogPost *post) |
void | removedPost (KBlog::BlogPost *post) |
![]() | |
BlogPrivate *const | d_ptr |
Detailed Description
A class that can be used for access to blogs (Wordpress, Drupal <5.6 and most likely many more) which simply use the yyyyMMddThh:mm:ss dateTime.iso8601 format stated on http://www.xmlrpc.com.
This is only an example for an ISO-8601 compatible format, but many blogs seem to assume exactly this format. This class is needed because KXmlRpc::Client only has support for the extended format yyyy-MM-ddThh:mm:ss which is also standard conform and makes more sense than the mixture above. This class reimplements createPost and modifyPost from scratch to send the dateTime in a compatible format (yyyyMMddThh:mm:ss).
The rest of the code is inherited from MovableType, as it does not use the dateTime format. The name is because this problem was first discovered with Wordpress.
Definition at line 68 of file wordpressbuggy.h.
Constructor & Destructor Documentation
|
explicit |
Create an object for WordpressBuggy.
- Parameters
-
server is the url for the xmlrpc gateway. parent is the parent object.
Definition at line 38 of file wordpressbuggy.cpp.
|
virtual |
Destroy the object.
Definition at line 51 of file wordpressbuggy.cpp.
|
protected |
Constructor needed for private inheritance.
Definition at line 44 of file wordpressbuggy.cpp.
Member Function Documentation
|
virtual |
Create a new post on server.
- Parameters
-
post is send to the server.
Reimplemented from KBlog::MovableType.
Definition at line 56 of file wordpressbuggy.cpp.
|
virtual |
Returns the of the inherited object.
Reimplemented from KBlog::MovableType.
Definition at line 273 of file wordpressbuggy.cpp.
|
virtual |
Modify a post on server.
- Parameters
-
post The post to be modified on the server. You need to set its id correctly.
Reimplemented from KBlog::MovableType.
Definition at line 169 of file wordpressbuggy.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:56 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.