KCalCore Library
#include <attendee.h>
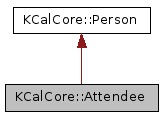
Public Types | |
typedef QVector< Ptr > | List |
enum | PartStat { NeedsAction, Accepted, Declined, Tentative, Delegated, Completed, InProcess, None } |
typedef QSharedPointer< Attendee > | Ptr |
enum | Role { ReqParticipant, OptParticipant, NonParticipant, Chair } |
Public Member Functions | |
Attendee (const QString &name, const QString &email, bool rsvp=false, PartStat status=None, Role role=ReqParticipant, const QString &uid=QString()) | |
Attendee (const Attendee &attendee) | |
~Attendee () | |
CustomProperties & | customProperties () |
const CustomProperties & | customProperties () const |
QString | delegate () const |
QString | delegator () const |
bool | operator!= (const Attendee &attendee) const |
Attendee & | operator= (const Attendee &attendee) |
bool | operator== (const Attendee &attendee) const |
Role | role () const |
bool | RSVP () const |
void | setCustomProperty (const QByteArray &xname, const QString &xvalue) |
void | setDelegate (const QString &delegate) |
void | setDelegator (const QString &delegator) |
void | setRole (Role role) |
void | setRSVP (bool rsvp) |
void | setStatus (PartStat status) |
void | setUid (const QString &uid) |
PartStat | status () const |
QString | uid () const |
Friends | |
KCALCORE_EXPORT QDataStream & | operator<< (QDataStream &s, const KCalCore::Attendee::Ptr &attendee) |
KCALCORE_EXPORT QDataStream & | operator>> (QDataStream &s, KCalCore::Attendee::Ptr &attendee) |
Detailed Description
Represents information related to an attendee of an Calendar Incidence, typically a meeting or task (to-do).
Attendees are people with a name and (optional) email address who are invited to participate in some way in a meeting or task. This class also tracks that status of the invitation: accepted; tentatively accepted; declined; delegated to another person; in-progress; completed.
Attendees may optionally be asked to RSVP ("Respond Please") to the invitation.
Note that each attendee be can optionally associated with a UID (unique identifier) derived from a Calendar Incidence, Email Message, or any other thing you want.
Definition at line 57 of file attendee.h.
Member Typedef Documentation
typedef QVector<Ptr> KCalCore::Attendee::List |
List of attendees.
Definition at line 99 of file attendee.h.
typedef QSharedPointer<Attendee> KCalCore::Attendee::Ptr |
A shared pointer to an Attendee object.
Definition at line 94 of file attendee.h.
Member Enumeration Documentation
The different types of participant status.
The meaning is specific to the incidence type in context.
Enumerator | |
---|---|
NeedsAction |
Event, to-do or journal needs action (default) |
Accepted |
Event, to-do or journal accepted. |
Declined |
Event, to-do or journal declined. |
Tentative |
Event or to-do tentatively accepted. |
Delegated |
Event or to-do delegated. |
Completed |
To-do completed. |
InProcess |
To-do in process of being completed. |
Definition at line 70 of file attendee.h.
The different types of participation roles.
Enumerator | |
---|---|
ReqParticipant |
Participation is required (default) |
OptParticipant |
Participation is optional. |
NonParticipant |
Non-Participant; copied for information purposes. |
Chair |
Chairperson. |
Definition at line 84 of file attendee.h.
Constructor & Destructor Documentation
Attendee::Attendee | ( | const QString & | name, |
const QString & | email, | ||
bool | rsvp = false , |
||
Attendee::PartStat | status = None , |
||
Attendee::Role | role = ReqParticipant , |
||
const QString & | uid = QString() |
||
) |
Constructs an attendee consisting of a Person name (name
) and email address (email
); invitation status and Role; an optional RSVP flag and UID.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
name is person name of the attendee. email is person email address of the attendee. rsvp if true, the attendee is requested to reply to invitations. status is the PartStat status of the attendee. role is the Role of the attendee. uid is the UID of the attendee.
Definition at line 58 of file attendee.cpp.
Attendee::Attendee | ( | const Attendee & | attendee | ) |
Constructs an attendee by copying another attendee.
- Parameters
-
attendee is the attendee to be copied.
Definition at line 70 of file attendee.cpp.
Attendee::~Attendee | ( | ) |
Destroys the attendee.
Definition at line 76 of file attendee.cpp.
Member Function Documentation
CustomProperties & Attendee::customProperties | ( | ) |
Returns a reference to the CustomProperties object.
Definition at line 176 of file attendee.cpp.
const CustomProperties & Attendee::customProperties | ( | ) | const |
Returns a const reference to the CustomProperties object.
Definition at line 181 of file attendee.cpp.
QString Attendee::delegate | ( | ) | const |
QString Attendee::delegator | ( | ) | const |
bool KCalCore::Attendee::operator!= | ( | const Attendee & | attendee | ) | const |
Compares this with attendee
for inequality.
- Parameters
-
attendee the attendee to compare.
Definition at line 93 of file attendee.cpp.
Sets this attendee equal to attendee
.
- Parameters
-
attendee is the attendee to copy.
Definition at line 98 of file attendee.cpp.
bool KCalCore::Attendee::operator== | ( | const Attendee & | attendee | ) | const |
Compares this with attendee
for equality.
- Parameters
-
attendee the attendee to compare.
Definition at line 81 of file attendee.cpp.
Attendee::Role Attendee::role | ( | ) | const |
bool Attendee::RSVP | ( | ) | const |
void Attendee::setCustomProperty | ( | const QByteArray & | xname, |
const QString & | xvalue | ||
) |
Adds a custom property.
If the property already exists it will be overwritten.
- Parameters
-
xname is the name of the property. xvalue is its value.
Definition at line 171 of file attendee.cpp.
void Attendee::setDelegate | ( | const QString & | delegate | ) |
Sets the delegate.
- Parameters
-
delegate is a string containing a MAILTO URI of those delegated to attend the meeting.
- See also
- delegate(), setDelegator().
Definition at line 151 of file attendee.cpp.
void Attendee::setDelegator | ( | const QString & | delegator | ) |
Sets the delegator.
- Parameters
-
delegator is a string containing a MAILTO URI of those who have delegated their meeting attendance.
- See also
- delegator(), setDelegate().
Definition at line 161 of file attendee.cpp.
void Attendee::setRole | ( | Attendee::Role | role | ) |
Sets the Role of the attendee to role
.
- Parameters
-
role is the Role to use for the attendee.
- See also
- role()
Definition at line 131 of file attendee.cpp.
void Attendee::setRSVP | ( | bool | rsvp | ) |
Sets the RSVP flag of the attendee to rsvp
.
- Parameters
-
rsvp if set (true), the attendee is requested to reply to invitations.
- See also
- RSVP()
Definition at line 111 of file attendee.cpp.
void Attendee::setStatus | ( | Attendee::PartStat | status | ) |
Sets the PartStat of the attendee to status
.
- Parameters
-
status is the PartStat to use for the attendee.
- See also
- status()
Definition at line 121 of file attendee.cpp.
void Attendee::setUid | ( | const QString & | uid | ) |
Sets the UID of the attendee to uid
.
- Parameters
-
uid is the UID to use for the attendee.
- See also
- uid()
Definition at line 141 of file attendee.cpp.
Attendee::PartStat Attendee::status | ( | ) | const |
Returns the PartStat of the attendee.
- See also
- setStatus()
Definition at line 126 of file attendee.cpp.
QString Attendee::uid | ( | ) | const |
Friends And Related Function Documentation
|
friend |
|
friend |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.