KMIME Library
#include <kmime_message.h>
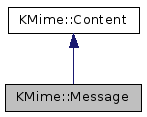
Public Types | |
typedef QList< KMime::Message * > | List |
typedef boost::shared_ptr < Message > | Ptr |
![]() | |
typedef QList< KMime::Content * > | List |
Public Member Functions | |
Message () | |
~Message () | |
virtual KMime::Headers::Bcc * | bcc (bool create=true) |
virtual KMime::Headers::Cc * | cc (bool create=true) |
virtual void | clear () |
virtual KMime::Headers::Date * | date (bool create=true) |
virtual KMime::Headers::From * | from (bool create=true) |
virtual KMIME_DEPRECATED KMime::Headers::Base * | getHeaderByType (const char *type) |
virtual KMime::Headers::Base * | headerByType (const char *type) |
virtual KMime::Headers::InReplyTo * | inReplyTo (bool create=true) |
virtual bool | isTopLevel () const |
Content * | mainBodyPart (const QByteArray &type=QByteArray()) |
virtual KMime::Headers::MessageID * | messageID (bool create=true) |
virtual KMime::Headers::Organization * | organization (bool create=true) |
virtual void | parse () |
virtual KMime::Headers::References * | references (bool create=true) |
virtual bool | removeHeader (const char *type) |
virtual KMime::Headers::ReplyTo * | replyTo (bool create=true) |
virtual KMime::Headers::Sender * | sender (bool create=true) |
virtual void | setHeader (KMime::Headers::Base *h) |
virtual KMime::Headers::Subject * | subject (bool create=true) |
virtual KMime::Headers::To * | to (bool create=true) |
virtual KMime::Headers::UserAgent * | userAgent (bool create=true) |
![]() | |
Content () | |
Content (Content *parent) | |
Content (const QByteArray &head, const QByteArray &body) | |
Content (const QByteArray &head, const QByteArray &body, Content *parent) | |
virtual | ~Content () |
void | addContent (Content *content, bool prepend=false) |
void | appendHeader (Headers::Base *h) |
virtual void | assemble () |
List | attachments (bool incAlternatives=false) |
QByteArray | body () const |
boost::shared_ptr< Message > | bodyAsMessage () const |
bool | bodyIsMessage () const |
void | changeEncoding (Headers::contentEncoding e) |
void | clearContents (bool del=true) |
Content * | content (const ContentIndex &index) const |
Headers::ContentDescription * | contentDescription (bool create=true) |
Headers::ContentDisposition * | contentDisposition (bool create=true) |
Headers::ContentID * | contentID (bool create=true) |
Headers::ContentLocation * | contentLocation (bool create=true) |
List | contents () const |
Headers::ContentTransferEncoding * | contentTransferEncoding (bool create=true) |
Headers::ContentType * | contentType (bool create=true) |
QByteArray | decodedContent () |
QString | decodedText (bool trimText=false, bool removeTrailingNewlines=false) |
QByteArray | defaultCharset () const |
QByteArray | encodedBody () |
QByteArray | encodedContent (bool useCrLf=false) |
QByteArray | epilogue () const |
bool | forceDefaultCharset () const |
void | fromUnicodeString (const QString &s) |
KMIME_DEPRECATED Headers::Generic * | getNextHeader (QByteArray &head) |
bool | hasContent () const |
bool | hasHeader (const char *type) |
QByteArray | head () const |
template<typename T > | |
T * | header (bool create=false) |
template<class T > | |
T * | headerInstance (T *, bool create) |
virtual QList< Headers::Base * > | headersByType (const char *type) |
ContentIndex | index () const |
ContentIndex | indexForContent (Content *content) const |
bool | isFrozen () const |
int | lineCount () const |
KMIME_DEPRECATED Headers::Generic * | nextHeader (QByteArray &head) |
Content * | parent () const |
QByteArray | preamble () const |
void | prependHeader (Headers::Base *h) |
void | removeContent (Content *content, bool del=false) |
void | setBody (const QByteArray &body) |
void | setContent (const QList< QByteArray > &l) |
void | setContent (const QByteArray &s) |
void | setDefaultCharset (const QByteArray &cs) |
void | setEpilogue (const QByteArray &epilogue) |
virtual void | setForceDefaultCharset (bool b) |
void | setFrozen (bool frozen=true) |
void | setHead (const QByteArray &head) |
void | setParent (Content *parent) |
void | setPreamble (const QByteArray &preamble) |
int | size () |
int | storageSize () const |
Content * | textContent () |
Content * | topLevel () const |
void | toStream (QTextStream &ts, bool scrambleFromLines=false) |
Static Public Member Functions | |
static QString | mimeType () |
Protected Member Functions | |
virtual QByteArray | assembleHeaders () |
![]() | |
bool | decodeText () |
template<class T > | |
KMIME_DEPRECATED T * | headerInstance (T *ptr, bool create) |
KMIME_DEPRECATED QByteArray | rawHeader (const char *name) const |
KMIME_DEPRECATED QList < QByteArray > | rawHeaders (const char *name) const |
Additional Inherited Members | |
![]() | |
Headers::Base::List | h_eaders |
Detailed Description
Represents a (email) message.
Sample how to create a multipart message:
Definition at line 81 of file kmime_message.h.
Member Typedef Documentation
typedef QList<KMime::Message*> KMime::Message::List |
A list of messages.
Definition at line 87 of file kmime_message.h.
typedef boost::shared_ptr<Message> KMime::Message::Ptr |
A shared pointer to a message object.
Definition at line 92 of file kmime_message.h.
Constructor & Destructor Documentation
KMime::Message::Message | ( | ) |
Creates an empty Message.
Definition at line 34 of file kmime_message.cpp.
KMime::Message::~Message | ( | ) |
Destroys this Message.
Definition at line 44 of file kmime_message.cpp.
Member Function Documentation
|
protectedvirtual |
Reimplement this method if you need to assemble additional headers in a derived class.
Don't forget to call the implementation of the base class.
- Returns
- The raw, assembled headers.
Reimplemented from KMime::Content.
Definition at line 54 of file kmime_message.cpp.
|
virtual |
Returns the Bcc header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns the Cc header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Clears the content, deleting all headers and sub-Contents.
Reimplemented from KMime::Content.
Definition at line 68 of file kmime_message.cpp.
|
virtual |
Returns the Date header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns the From header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Tries to find a type
header in the Content and returns it.
- Parameters
-
type the header type to find
Reimplemented from KMime::Content.
Definition at line 74 of file kmime_message.cpp.
|
virtual |
Returns the first header of type type
, if it exists.
Otherwise returns 0. Note that the returned header may be empty.
- Parameters
-
type the header type to find
- Since
- 4.2
Reimplemented from KMime::Content.
Definition at line 80 of file kmime_message.cpp.
|
virtual |
Returns the In-Reply-To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns true if this is the top-level node in the MIME tree.
The top-level node is always a Message or NewsArticle. However, a node can be a Message without being a top-level node when it is an encapsulated message.
Reimplemented from KMime::Content.
Definition at line 98 of file kmime_message.cpp.
Content * KMime::Message::mainBodyPart | ( | const QByteArray & | type = QByteArray() | ) |
Returns the first main body part of a given type, taking multipart/mixed and multipart/alternative nodes into consideration.
Eg. bodyPart
("text/html") will return a html content object if that is provided in a multipart/alternative node, but not if it's the non-first child node of a multipart/mixed node (ie. an attachment).
- Parameters
-
type The mimetype of the body part, if not given, the first body part will be returned, regardless of it's type.
Definition at line 103 of file kmime_message.cpp.
|
virtual |
Returns the Message-ID header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
static |
Returns the MIME type used for Messages.
Definition at line 140 of file kmime_message.cpp.
|
virtual |
Returns the Organization header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Parses the Content.
This means the broken-down object representation of the Content is updated from the string representation of the Content.
Call this if you want to access or change headers, sub-Contents or the encapsulated message.
- Note
- Calling parse() twice will not work for multipart contents or for contents of which the body is an encapsulated message. The reason is that the first parse() will delete the body, so there is no body to work on for the second call of parse().
- Calling this will reset the message returned by bodyAsMessage(), as the message is re-parsed as well. Also, all old sub-contents will be deleted, so any old Content pointer will become invalid.
Reimplemented from KMime::Content.
Definition at line 48 of file kmime_message.cpp.
|
virtual |
Returns the References header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Searches for the first header of type type
, and deletes it, removing it from this Content.
- Parameters
-
type The type of the header to look for.
- Returns
- true if a header was found and removed.
Reimplemented from KMime::Content.
Definition at line 92 of file kmime_message.cpp.
|
virtual |
Returns the Reply-To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns the Sender header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Sets the specified header to this Content.
Any previous header of the same type is removed. If you need multiple headers of the same type, use appendHeader() or prependHeader().
- Parameters
-
h The header to set.
- See also
- appendHeader()
- removeHeader()
- Since
- 4.4
Reimplemented from KMime::Content.
Definition at line 86 of file kmime_message.cpp.
|
virtual |
Returns the Subject header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns the To header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
|
virtual |
Returns the User-Agent header.
- Parameters
-
create If true, create the header if it doesn't exist yet.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.