okteta
#include <numberrange.h>
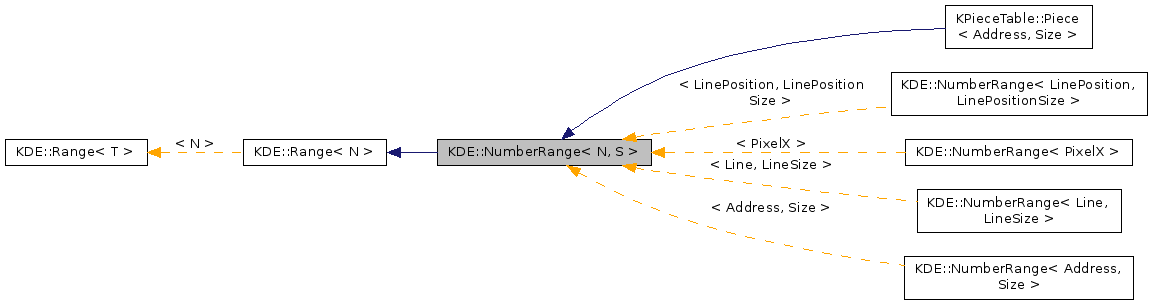
Public Member Functions | |
NumberRange (N startIndex, N endIndex) | |
NumberRange (const NumberRange &other) | |
NumberRange () | |
~NumberRange () | |
void | adaptToReplacement (N offset, S removedLength, S insertedLength) |
bool | append (const NumberRange &other) |
bool | isJoinable (const NumberRange &other) const |
N | localIndex (N index) const |
NumberRange | localRange (const NumberRange &other) const |
void | moveToEnd (N end) |
void | moveToStart (N other) |
N | nextBeforeStart () const |
N | nextBehindEnd () const |
NumberRange & | operator= (const NumberRange &other) |
bool | operator== (const NumberRange &other) const |
bool | prepend (const NumberRange &other) |
NumberRange | remove (const NumberRange &removeRange) |
NumberRange | removeLocal (const NumberRange &removeRange) |
void | restrictEndByWidth (S width) |
void | setByWidth (N other, S width) |
void | setEndByWidth (S width) |
void | setEndNextBefore (const NumberRange &other) |
void | setEndNextBefore (N index) |
void | setStartByWidth (S width) |
void | setStartNextBehind (const NumberRange &other) |
void | setStartNextBehind (N index) |
NumberRange | splitAt (N index) |
NumberRange | splitAtLocal (N index) |
N | startForInclude (const NumberRange &other) const |
NumberRange | subRange (const NumberRange &localRange) const |
S | width () const |
![]() | |
Range (NS, NE) | |
Range () | |
~Range () | |
N | end () const |
bool | endsBefore (NValue) const |
bool | endsBefore (const Range &R) const |
bool | endsBehind (NValue) const |
bool | endsBehind (const Range &R) const |
void | extendEndTo (NLimit) |
void | extendStartTo (NLimit) |
void | extendTo (const Range &Limit) |
bool | includes (NValue) const |
bool | includes (const Range &R) const |
bool | includesInside (NValue) const |
bool | includesInside (const Range &R) const |
bool | isEmpty () const |
bool | isValid () const |
void | moveBy (ND) |
void | moveEndBy (ND) |
void | moveStartBy (ND) |
Range & | operator= (const Range &R) |
bool | operator== (const Range &R) const |
bool | overlaps (const Range &R) const |
void | restrictEndTo (NLimit) |
void | restrictStartTo (NLimit) |
void | restrictTo (const Range &Limit) |
void | set (NS, NE) |
void | set (const Range &R) |
void | setEnd (NE) |
void | setStart (NS) |
N | start () const |
bool | startsBefore (NValue) const |
bool | startsBefore (const Range &R) const |
bool | startsBehind (NValue) const |
bool | startsBehind (const Range &R) const |
void | unset () |
Static Public Member Functions | |
static NumberRange | fromWidth (N startIndex, S width) |
static NumberRange | fromWidth (S width) |
Additional Inherited Members | |
![]() | |
const N | null () const |
![]() | |
N | End |
N | Start |
Detailed Description
template<typename N, typename S = N>
class KDE::NumberRange< N, S >
describes a range of numbers which have a distance of 1 each
Definition at line 37 of file numberrange.h.
Constructor & Destructor Documentation
|
inline |
constructs a range
- Parameters
-
startIndex starting index endIndex end index
Definition at line 53 of file numberrange.h.
|
inline |
Definition at line 54 of file numberrange.h.
|
inline |
Definition at line 55 of file numberrange.h.
|
inline |
Definition at line 57 of file numberrange.h.
Member Function Documentation
|
inline |
Definition at line 226 of file numberrange.h.
|
inline |
Definition at line 222 of file numberrange.h.
|
inlinestatic |
constructs a range by width
- Parameters
-
startIndex starting index width width of the range
Definition at line 130 of file numberrange.h.
|
inlinestatic |
Definition at line 132 of file numberrange.h.
|
inline |
- Returns
- true if both range . If one of both is invalid the behaviour is undefined
Definition at line 215 of file numberrange.h.
|
inline |
- Returns
- index relative to the start
Definition at line 199 of file numberrange.h.
|
inline |
Definition at line 201 of file numberrange.h.
|
inline |
moves the range defined by a new end.
If the range is invalid the behaviour is undefined
Definition at line 167 of file numberrange.h.
|
inline |
moves the range defined by a new start.
If the range is invalid the behaviour is undefined
Definition at line 165 of file numberrange.h.
|
inline |
Definition at line 143 of file numberrange.h.
|
inline |
Definition at line 145 of file numberrange.h.
|
inline |
Definition at line 138 of file numberrange.h.
|
inline |
Definition at line 135 of file numberrange.h.
|
inline |
Definition at line 219 of file numberrange.h.
|
inline |
Definition at line 184 of file numberrange.h.
|
inline |
Definition at line 191 of file numberrange.h.
|
inline |
restricts the end by width.
If the range is invalid the behaviour is undefined
Definition at line 162 of file numberrange.h.
|
inline |
Definition at line 148 of file numberrange.h.
|
inline |
sets the last index of the range's range to be width-1 behind the start If the range is invalid the behaviour is undefined
Definition at line 152 of file numberrange.h.
|
inline |
sets the first index of the range's range one behind the other's end If one of both is invalid or the other' start is 0 the behaviour is undefined
Definition at line 158 of file numberrange.h.
|
inline |
Definition at line 160 of file numberrange.h.
|
inline |
sets the first index of the range's range to be width-1 before the end If the range is invalid the behaviour is undefined
Definition at line 150 of file numberrange.h.
|
inline |
sets the first index of the range's range one behind the other's end If one of both is invalid the behaviour is undefined
Definition at line 154 of file numberrange.h.
|
inline |
Definition at line 156 of file numberrange.h.
|
inline |
Definition at line 170 of file numberrange.h.
|
inline |
Definition at line 177 of file numberrange.h.
|
inline |
- Returns
- the needed start so that other gets included, undefined if any is invalid
Definition at line 208 of file numberrange.h.
|
inline |
- Returns
- range given by local
Definition at line 204 of file numberrange.h.
|
inline |
- Returns
- the numbered of included indizes or 0, if the range is invalid
Definition at line 141 of file numberrange.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.