superkaramba
#include <graph.h>
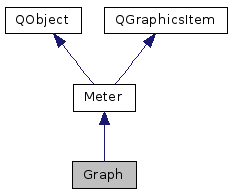
Public Member Functions | |
Graph (Karamba *k, int ix, int iy, int iw, int ih, int nbrPoints) | |
Graph () | |
~Graph () | |
QColor | getFillColor () const |
QString | getPlotDirection () const |
QString | getScrollDirection () const |
int | getValue () const |
void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
void | setFillColor (QColor) |
void | setPlotDirection (const QString &) |
void | setScrollDirection (const QString &) |
void | setValue (int) |
void | setValue (const QString &) |
bool | shouldFill () const |
void | shouldFill (bool b) |
![]() | |
Meter (Karamba *k, int ix, int iy, int iw, int ih) | |
Meter (Karamba *k) | |
virtual | ~Meter () |
virtual QRectF | boundingRect () const |
virtual QColor | getColor () const |
virtual int | getHeight () const |
virtual int | getMax () const |
virtual int | getMin () const |
virtual QString | getStringValue () const |
virtual int | getWidth () const |
virtual int | getX () const |
virtual int | getY () const |
virtual void | hide () |
bool | isEnabled () const |
virtual void | setColor (QColor color) |
void | setEnabled (bool enable) |
virtual void | setHeight (int) |
virtual void | setMax (int max) |
virtual void | setMin (int min) |
virtual void | setSize (int x, int y, int width, int height) |
virtual void | setWidth (int) |
virtual void | setX (int) |
virtual void | setY (int) |
virtual void | show () |
Additional Inherited Members | |
![]() | |
QRectF | m_boundingBox |
bool | m_clickable |
QColor | m_color |
bool | m_hidden |
Karamba * | m_karamba |
int | m_maxValue |
int | m_minValue |
Detailed Description
Definition at line 19 of file meters/graph.h.
Constructor & Destructor Documentation
Graph::Graph | ( | Karamba * | k, |
int | ix, | ||
int | iy, | ||
int | iw, | ||
int | ih, | ||
int | nbrPoints | ||
) |
Definition at line 13 of file meters/graph.cpp.
Graph::Graph | ( | ) |
Graph::~Graph | ( | ) |
Definition at line 30 of file meters/graph.cpp.
Member Function Documentation
QColor Graph::getFillColor | ( | ) | const |
Definition at line 33 of file meters/graph.cpp.
QString Graph::getPlotDirection | ( | ) | const |
Definition at line 112 of file meters/graph.cpp.
QString Graph::getScrollDirection | ( | ) | const |
Definition at line 98 of file meters/graph.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 57 of file meters/graph.cpp.
|
virtual |
Notes on graphing rewrite - jwm.
Regardless of scroll direction, data is kept in m_values in order of most recent value first trailing off to the oldest value getting dropped from the list when it is at an index of nbrPoints: V1, V2, V3, ... ,Vn where n=nbrPoints It is handier to use zero indexes, so we'll use n = nbrPoints - 1 and the following value list definition: V0, V1, V2, ... , Vn where n = nbrPoint - 1
Scroll: (NOTE: when talking about scroll right or scroll left, I'm not sure which is more intuitive...to talk about which way the old data moves off of the screen or which way a "virtual" scroll bar would move...in the end I don't really care.
Paint: During the creation of the polygon if the graph scrolls "left" that means that the newest values appear to be coming in off of the left (or a "virtual" scroll bar is being moved to the left). The oldest data will move off to the right and eventually drop off of the graph. This matches the natural order of the underlying data as represented above going from V0 to Vn. For left scrolling, this order must be reversed. When one primes the vector to be the right size and filled with 0's, the logic is simpler and more consistent. Indexes into the value array and the position on the polygon vector become functions of each other at all times. This eliminates the need for the previous approach of tracking pixel positions less than the current count of data points to graph and it also eliminates the need for all of the translation calls that were happening. Original graph code translated the polygon points to shift them over one 'step' before appending the next value. The approach below should be considerably less compute intensive especially as the number of data points grows.
Invariants with scroll direction: m_values V0, V1, V2, ... Vn horizontal step move right by (pixel width count / data point count - 1) simplisticly, if the graph is 100 wide and there are 5 data points, the step value is 25 vertical step factor: This is the distance between each data point and is given by: (pixel height count / (# possible int data points)) so if the graph is 20 tall, and has a min of -10 and a max of 10, the absolute distance is max - min, but the number of points that involves is max - min + 1 n: n = nbrPoints - 1 (since n is zero based)
SCROLL_LEFT: Polygon { P0(V0), P1(V1), P2(V2), ... Pn(Vn) }
- i goes from 0 to n, k also goes from o to n at the same time
- append P to the end of the polygon making it Pk(step * i, Vi) where k == i
SCROLL_RIGHT: Polygon { P0(Vn), P1(Vn-1), P2(Vn-2), ... Pn(V0) }
- i goes from n to 0, k goes from 0 to n (same as before)
- append P to the end of the polygon making it Pk(step * k, Vi) where k = n - i (which is the same as i = n - k)
Implements Meter.
Definition at line 172 of file meters/graph.cpp.
void Graph::setFillColor | ( | QColor | c | ) |
Definition at line 37 of file meters/graph.cpp.
void Graph::setPlotDirection | ( | const QString & | dir | ) |
Definition at line 105 of file meters/graph.cpp.
void Graph::setScrollDirection | ( | const QString & | dir | ) |
Definition at line 90 of file meters/graph.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 62 of file meters/graph.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 79 of file meters/graph.cpp.
bool Graph::shouldFill | ( | ) | const |
Definition at line 52 of file meters/graph.cpp.
void Graph::shouldFill | ( | bool | b | ) |
Definition at line 44 of file meters/graph.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.