superkaramba
#include <textlabel.h>
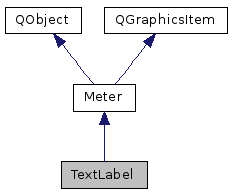
Public Types | |
enum | ScrollType { ScrollNone, ScrollNormal, ScrollBackAndForth, ScrollOnePass } |
Public Member Functions | |
TextLabel (Karamba *k, int x, int y, int w, int h) | |
TextLabel (Karamba *k) | |
~TextLabel () | |
void | allowClick (bool enable) |
void | attachClickArea (const QString &leftMouseButton, const QString &middleMouseButton, const QString &rightMouseButton) |
QRectF | boundingRect () const |
bool | clickable () |
QString | getAlignment () const |
QColor | getBGColor () const |
bool | getFixedPitch () const |
QString | getFont () const |
int | getFontSize () const |
int | getHeight () const |
int | getShadow () const |
QString | getStringValue () const |
int | getTextWidth () const |
int | getWidth () const |
int | getX () const |
int | getY () const |
virtual void | hide () |
bool | mouseEvent (QEvent *e) |
void | paint (QPainter *p, const QStyleOptionGraphicsItem *option, QWidget *widget) |
void | setAlignment (const QString &) |
void | setBGColor (QColor clr) |
void | setFixedPitch (bool) |
void | setFont (const QString &) |
void | setFontSize (int) |
void | setScroll (ScrollType type, QPoint speed, int gap, int pause) |
void | setScroll (const QString &type, const QPoint &speed, int gap, int pause) |
void | setShadow (int) |
void | setSize (int x, int y, int width, int height) |
void | setTextProps (TextField *) |
void | setValue (const QString &text) |
void | setValue (int) |
virtual void | show () |
![]() | |
Meter (Karamba *k, int ix, int iy, int iw, int ih) | |
Meter (Karamba *k) | |
virtual | ~Meter () |
virtual QColor | getColor () const |
virtual int | getMax () const |
virtual int | getMin () const |
virtual int | getValue () const |
bool | isEnabled () const |
virtual void | setColor (QColor color) |
void | setEnabled (bool enable) |
virtual void | setHeight (int) |
virtual void | setMax (int max) |
virtual void | setMin (int min) |
virtual void | setWidth (int) |
virtual void | setX (int) |
virtual void | setY (int) |
Additional Inherited Members | |
![]() | |
QRectF | m_boundingBox |
bool | m_clickable |
QColor | m_color |
bool | m_hidden |
Karamba * | m_karamba |
int | m_maxValue |
int | m_minValue |
Detailed Description
Definition at line 19 of file meters/textlabel.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ScrollNone | |
ScrollNormal | |
ScrollBackAndForth | |
ScrollOnePass |
Definition at line 23 of file meters/textlabel.h.
Constructor & Destructor Documentation
TextLabel::TextLabel | ( | Karamba * | k, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h | ||
) |
Definition at line 22 of file meters/textlabel.cpp.
TextLabel::TextLabel | ( | Karamba * | k | ) |
Definition at line 53 of file meters/textlabel.cpp.
TextLabel::~TextLabel | ( | ) |
Definition at line 69 of file meters/textlabel.cpp.
Member Function Documentation
void TextLabel::allowClick | ( | bool | enable | ) |
Definition at line 424 of file meters/textlabel.cpp.
void TextLabel::attachClickArea | ( | const QString & | leftMouseButton, |
const QString & | middleMouseButton, | ||
const QString & | rightMouseButton | ||
) |
Definition at line 434 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 445 of file meters/textlabel.cpp.
bool TextLabel::clickable | ( | ) |
Definition at line 429 of file meters/textlabel.cpp.
QString TextLabel::getAlignment | ( | ) | const |
Definition at line 203 of file meters/textlabel.cpp.
QColor TextLabel::getBGColor | ( | ) | const |
Definition at line 163 of file meters/textlabel.cpp.
bool TextLabel::getFixedPitch | ( | ) | const |
Definition at line 218 of file meters/textlabel.cpp.
QString TextLabel::getFont | ( | ) | const |
Definition at line 174 of file meters/textlabel.cpp.
int TextLabel::getFontSize | ( | ) | const |
Definition at line 185 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 475 of file meters/textlabel.cpp.
int TextLabel::getShadow | ( | ) | const |
Definition at line 228 of file meters/textlabel.cpp.
|
inlinevirtual |
Reimplemented from Meter.
Definition at line 34 of file meters/textlabel.h.
int TextLabel::getTextWidth | ( | ) | const |
Definition at line 450 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 466 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 456 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 461 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 78 of file meters/textlabel.cpp.
bool TextLabel::mouseEvent | ( | QEvent * | e | ) |
Definition at line 397 of file meters/textlabel.cpp.
|
virtual |
Implements Meter.
Definition at line 348 of file meters/textlabel.cpp.
void TextLabel::setAlignment | ( | const QString & | align | ) |
Definition at line 190 of file meters/textlabel.cpp.
void TextLabel::setBGColor | ( | QColor | clr | ) |
Definition at line 158 of file meters/textlabel.cpp.
void TextLabel::setFixedPitch | ( | bool | fp | ) |
Definition at line 213 of file meters/textlabel.cpp.
void TextLabel::setFont | ( | const QString & | f | ) |
Definition at line 168 of file meters/textlabel.cpp.
void TextLabel::setFontSize | ( | int | size | ) |
Definition at line 179 of file meters/textlabel.cpp.
void TextLabel::setScroll | ( | ScrollType | type, |
QPoint | speed, | ||
int | gap, | ||
int | pause | ||
) |
Definition at line 250 of file meters/textlabel.cpp.
void TextLabel::setScroll | ( | const QString & | type, |
const QPoint & | speed, | ||
int | gap, | ||
int | pause | ||
) |
Definition at line 233 of file meters/textlabel.cpp.
void TextLabel::setShadow | ( | int | s | ) |
Definition at line 223 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 484 of file meters/textlabel.cpp.
void TextLabel::setTextProps | ( | TextField * | field | ) |
Definition at line 84 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 144 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 151 of file meters/textlabel.cpp.
|
virtual |
Reimplemented from Meter.
Definition at line 72 of file meters/textlabel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.