superkaramba
#include <Python.h>
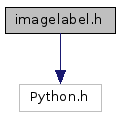
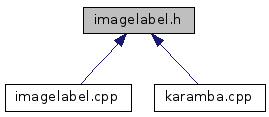
Go to the source code of this file.
Function Documentation
Image/addImageTooltip.
SYNOPSIS long addImageTooltip(widget, image, text) DESCRIPTION This creats a tooltip for image with tooltip_text.
Note:
- If you move the image, the tooltip does not move! It stays! Do not create a tooltip if the image is off-screen because you will not be able to ever see it. ARGUMENTS
- long widget – karamba
- long image – pointer to image
- string text – tooltip text RETURN VALUE 1 if successful
Definition at line 311 of file python/imagelabel.cpp.
Image/changeImageChannelIntensity.
SYNOPSIS long changeImageChannelIntensity(widget, image, ratio, channel, millisec) DESCRIPTION Changes the "intensity" of the image color channel, which is similar to it's brightness. ARGUMENTS
- long widget – karamba
- long image – pointer to image
- float ratio – -1.0 to 1.0 (dark to bright)
- string channel – color channel (red|green|blue)
- long millisec – milliseconds before the image is restored RETURN VALUE 1 if successful
Definition at line 211 of file python/imagelabel.cpp.
Image/changeImageIntensity.
SYNOPSIS long changeImageIntensity(widget, image, ratio, millisec) DESCRIPTION Changes the "intensity" of the image, which is similar to it's brightness. ratio is a floating point number from -1.0 to 1.0 that determines how much to brighten or darken the image. Millisec specifies how long in milliseconds before the image is restored to it's original form. This is useful for "mouse over" type animations. Using 0 for millisec disables this feature and leaves the image permanently affected. ARGUMENTS
- long widget – karamba
- long image – pointer to image
- float ratio – -1.0 to 1.0 (dark to bright)
- long millisec – milliseconds before the image is restored RETURN VALUE 1 if successful
Definition at line 197 of file python/imagelabel.cpp.
Image/changeImageToGray.
SYNOPSIS long changeImageToGray(widget, image, millisec) DESCRIPTION Turns the given image into a grayscale image. Millisec specifies how long in milliseconds before the image is restored to it's original form. This is useful for "mouse over" type animations. Using 0 for millisec disables this feature and leaves the image permanently affected. ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long millisec – milliseconds before the image is restored RETURN VALUE 1 if successful
Definition at line 226 of file python/imagelabel.cpp.
Image/createBackgroundImage.
SYNOPSIS long createBackgroundImage(widget, x, y, w, h, image) DESCRIPTION This creates an background image on your widget at x, y. The filename should be given as the path parameter. In theory the image could be local or could be a url. It works just like adding an image in your theme file. You will need to save the return value to be able to call other functions on your image, such as moveImage() ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- string image – image for the imagelabel RETURN VALUE Pointer to new image meter
Definition at line 69 of file python/imagelabel.cpp.
Image/createImage.
SYNOPSIS long createImage(widget, x, y, image) DESCRIPTION This creates an image on your widget at x, y. The filename should be given as the path parameter. In theory the image could be local or could be a url. It works just like adding an image in your theme file. You will need to save the return value to be able to call other functions on your image, such as moveImage() ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- string image – image for the imagelabel RETURN VALUE Pointer to new image meter
Definition at line 56 of file python/imagelabel.cpp.
Image/createTaskIcon.
SYNOPSIS long createTaskIcon(widget, x, y, ctask) DESCRIPTION This creates a task image at x,y. ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- long task – task RETURN VALUE Pointer to new image meter
Image/createTaskIcon.
- Parameters
-
ctask the window id of the task to create the icon for
Definition at line 88 of file python/imagelabel.cpp.
Image/deleteImage.
SYNOPSIS long deleteImage(widget, image) DESCRIPTION This removes image from memory. Please do not call functions on "image" after calling deleteImage, as it does not exist anymore and that could cause crashes in some cases. ARGUMENTS
- long widget – karamba
- long widget – image RETURN VALUE 1 if successful
Definition at line 123 of file python/imagelabel.cpp.
Image/getImageHeight.
SYNOPSIS long getImageSize(widget, image) DESCRIPTION This returns the height of an image. This is useful if you have rotated an image and its size changed, so you do not know how big it is anymore. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE height
Definition at line 262 of file python/imagelabel.cpp.
Image/getImagePos.
SYNOPSIS tuple getImagePos(widget, image) DESCRIPTION Given a reference to a image object, this will return a tuple containing the x and y coordinate of a image object. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE pos
Definition at line 141 of file python/imagelabel.cpp.
Image/getImageSensor.
SYNOPSIS string getImageSensor(widget, image) DESCRIPTION Get current sensor string ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE sensor string
Definition at line 176 of file python/imagelabel.cpp.
Image/getImageSize.
SYNOPSIS tuple getImageSize(widget, image) DESCRIPTION Given a reference to a image object, this will return a tuple containing the height and width of a image object. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE size
Definition at line 146 of file python/imagelabel.cpp.
Image/getImagePath.
SYNOPSIS string getImagePath(widget, image) DESCRIPTION Returns current image path. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE path
Definition at line 166 of file python/imagelabel.cpp.
Image/getImageWidth.
SYNOPSIS long getImageSize(widget, image) DESCRIPTION This returns the width of an image. This is useful if you have rotated an image and its size changed, so you do not know how big it is anymore. // ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE width
Definition at line 272 of file python/imagelabel.cpp.
Image/getThemeImage.
SYNOPSIS long getThemeImage(widget, name) DESCRIPTION You can reference an image in your python code that was created in the .theme file. Basically, you just add a NAME= value to the IMAGE line in the .theme file. Then if you want to use that object, instead of calling createImage, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- long widget – karamba
- string name – name of the image to get RETURN VALUE Pointer to image
Definition at line 136 of file python/imagelabel.cpp.
Image/hideImage.
SYNOPSIS long hideImage(widget, image) DESCRIPTION This hides an image. In other words, during subsequent calls to widgetUpdate(), this image will not be drawn. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE 1 if successful
Definition at line 156 of file python/imagelabel.cpp.
Image/moveImage.
SYNOPSIS long moveImage(widget, image, x, y) DESCRIPTION This moves an image to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. The imageToMove parameter is a reference to the image to move that you saved as the return value from createImage() ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long x – x coordinate
- long y – y coordinate RETURN VALUE 1 if successful
Definition at line 151 of file python/imagelabel.cpp.
Image/removeImageEffects.
SYNOPSIS long removeImageEffects(widget, image) DESCRIPTION If you have called image effect commands on your image (ex: changeImageIntensity), you can call this to restore your image to it's original form. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE 1 if successful
Definition at line 186 of file python/imagelabel.cpp.
Image/removeImageTransformations.
SYNOPSIS long removeImageTransformations(widget, image) DESCRIPTION If you have rotated or resized your image, you can call this to restore your image to it's original form. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE 1 if successful
Definition at line 239 of file python/imagelabel.cpp.
Image/resizeImage.
SYNOPSIS long resizeImage(widget, image, w, h) DESCRIPTION This resizes your image to width, height. The imageToResize parameter is a reference to an image that you saved as the return value from createImage() ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long w – width
- long h – height RETURN VALUE 1 if successful
Definition at line 298 of file python/imagelabel.cpp.
Image/resizeImageSmooth.
SYNOPSIS long resizeImageSmooth(widget, image, w, h) DESCRIPTION DEPRECATED: resizeImage now allows the user to pick whether to use fast or smooth resizing from the SuperKaramba menu - This resizes your image to width, height. The imageToResize parameter is a reference to an image that you saved as the return value from createImage() ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long w – width
- long h – height RETURN VALUE 1 if successful
Definition at line 282 of file python/imagelabel.cpp.
Image/rotateImage.
SYNOPSIS long rotateImage(widget, image, deg) DESCRIPTION This rotates your image to by the specified amount of degrees. The imageToRotate parameter is a reference to an image that you saved as the return value from createImage() ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long deg – degrees to rotate RETURN VALUE 1 if successful
Definition at line 250 of file python/imagelabel.cpp.
Image/setImageSensor.
SYNOPSIS long setImageSensor(widget, image, sensor) DESCRIPTION Get current sensor string ARGUMENTS
- long widget – karamba
- long image – pointer to image
- string sensor – new sensor as in theme files RETURN VALUE 1 if successful
Definition at line 181 of file python/imagelabel.cpp.
Image/setImagePath.
SYNOPSIS long setImagePath(widget, image, path) DESCRIPTION This will change image of a image widget. ARGUMENTS
- long widget – karamba
- long image – pointer to image
- long path – new path RETURN VALUE 1 if successful
Definition at line 171 of file python/imagelabel.cpp.
Image/showImage.
SYNOPSIS long showImage(widget, image) DESCRIPTION This shows a previously hidden image. It does not actually refresh the image on screen. That is what redrawWidget() does. ARGUMENTS
- long widget – karamba
- long image – pointer to image RETURN VALUE 1 if successful
Definition at line 161 of file python/imagelabel.cpp.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.