superkaramba
#include <Python.h>
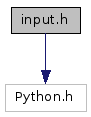
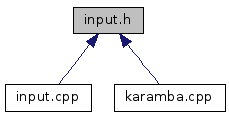
Go to the source code of this file.
Function Documentation
InputBox/clearInputFocus.
SYNOPSIS long clearInputFocus(widget, inputBox) DESCRIPTION releases the Input Focus from the Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE 1 if successful
Definition at line 326 of file python/input.cpp.
InputBox/createInputBox.
SYNOPSIS long createInputBox(widget, x, y, w, h, text) DESCRIPTION This creates a Input Box at x, y with width and height w, h. You need to save the return value of this function to call other functions on your Input Box field, such as changeInputBox(). The karamba widget is automatically set active, to allow user interactions. ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- long w – width
- long h – height
- string text – text for the Input Box RETURN VALUE Pointer to new Input Box
Definition at line 44 of file python/input.cpp.
InputBox/deleteInputBox.
SYNOPSIS long deleteInputBox(widget, inputBox) DESCRIPTION This removes a Input Box object from memory. Please do not call functions of the Input Box after calling deleteInputBox, as it does not exist anymore and that could cause crashes in some cases. The karamba widget is automatically set passive, when no more Input Boxes are on the karamba widget. ARGUMENTS
- long widget – karamba
- long widget – inputBox RETURN VALUE 1 if successful
Definition at line 63 of file python/input.cpp.
InputBox/getInputBoxBackgroundColor.
SYNOPSIS tuple getInputBoxBackgroundColor(widget, inputBox) DESCRIPTION Get current background color of a Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE (red, green, blue)
Definition at line 217 of file python/input.cpp.
InputBox/getInputBoxFont.
SYNOPSIS string getInputBoxFont(widget, inputBox) DESCRIPTION Get current Input Box font name ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE font name
Definition at line 137 of file python/input.cpp.
InputBox/getInputBoxFontColor.
SYNOPSIS tuple getInputBoxFontColor(widget, inputBox) DESCRIPTION Get current text color of a Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE (red, green, blue)
Definition at line 163 of file python/input.cpp.
InputBox/getInputBoxFontSize.
SYNOPSIS long getInputBoxFontSize(widget, inputBox) DESCRIPTION Get current text font size ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE text font size
Definition at line 299 of file python/input.cpp.
InputBox/getInputBoxFrameColor.
SYNOPSIS tuple getInputBoxFrameColor(widget, inputBox) DESCRIPTION Get current frame color of a Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE (red, green, blue)
Definition at line 244 of file python/input.cpp.
InputBox/getInputBoxPos.
SYNOPSIS tuple getInputBoxPos(widget, inputBox) DESCRIPTION Given a reference to a Input Box object, this will return a tuple containing the x and y coordinate of a Input Box object. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE pos
Definition at line 102 of file python/input.cpp.
InputBox/getInputBoxSelectedTextColor.
SYNOPSIS tuple getInputBoxSelectedTextColor(widget, inputBox) DESCRIPTION Get current selected text color of a Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE (red, green, blue)
Definition at line 271 of file python/input.cpp.
InputBox/getInputBoxSelectionColor.
SYNOPSIS tuple getInputBoxSelectionColor(widget, inputBox) DESCRIPTION Get current selection color of a Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE (red, green, blue)
Definition at line 190 of file python/input.cpp.
InputBox/getInputBoxSize.
SYNOPSIS tuple getInputBoxSize(widget, inputBox) DESCRIPTION Given a reference to a Input Box object, this will return a tuple containing the height and width of a Input Box object. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE size
Definition at line 112 of file python/input.cpp.
InputBox/getInputBoxValue.
SYNOPSIS string getInputBoxValue(widget, inputBox) DESCRIPTION Returns current Input Box text. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to text RETURN VALUE value
Definition at line 82 of file python/input.cpp.
InputBox/getInputFocus.
SYNOPSIS long getInputFocus(widget) DESCRIPTION Get the Input Box currently focused ARGUMENTS
- long widget – karamba RETURN VALUE the input box or 0
Definition at line 339 of file python/input.cpp.
InputBox/getThemeInputBox.
SYNOPSIS long getThemeInputBox(widget, name) DESCRIPTION You can reference text in your python code that was created in the theme file. Basically, you just add a NAME= value to the INPUT line in the .theme file. Then if you want to use that object, instead of calling createInputBox, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
Definition at line 77 of file python/input.cpp.
InputBox/hideInputBox.
SYNOPSIS long hideInputBox(widget, inputBox) DESCRIPTION Hides a Input Box that is visible. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE 1 if successful
Definition at line 92 of file python/input.cpp.
InputBox/moveInputBox.
SYNOPSIS long moveInputBox(widget, inputBox, x, y) DESCRIPTION This moves a Input Box object to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long x – x coordinate
- long y – y coordinate RETURN VALUE 1 if successful
Definition at line 107 of file python/input.cpp.
InputBox/resizeInputBox.
SYNOPSIS long resizeInputBox(widget, inputBox, w, h) DESCRIPTION This will resize Input Box to new height and width. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long w – new width
- long h – new height RETURN VALUE 1 if successful
Definition at line 117 of file python/input.cpp.
InputBox/changeInputBoxBackgroundColor.
SYNOPSIS long changeInputBoxBackgroundColor(widget, inputBox, r, g, b) DESCRIPTION This will change the background color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, and blue. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long red – red component of color
- long green – green component of color
- long blue – blue component of color RETURN VALUE 1 if successful
Definition at line 203 of file python/input.cpp.
InputBox/changeInputBoxFont.
SYNOPSIS long changeInputBoxFont(widget, inputBox, font) DESCRIPTION This will change the font of a Input Box widget. InputBox is the reference to the Input Box object to change. Font is a string with the name of the font to use. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to inputBox
- string font – font name RETURN VALUE 1 if successful
Definition at line 122 of file python/input.cpp.
InputBox/changeInputBoxFontColor.
SYNOPSIS long changeInputBoxFontColor(widget, inputBox, r, g, b) DESCRIPTION This will change the color of a text of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, and blue. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long red – red component of color
- long green – green component of color
- long blue – blue component of color RETURN VALUE 1 if successful
Definition at line 149 of file python/input.cpp.
InputBox/changeInputBoxFontSize.
SYNOPSIS long changeInputBoxFontSize(widget, text, size) DESCRIPTION This will change the font size of a Input Box widget. InputBox is the reference to the text object to change. Size is the new font point size. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long size – new size for text RETURN VALUE 1 if successful
Definition at line 284 of file python/input.cpp.
InputBox/changeInputBoxFrameColor.
SYNOPSIS long changeInputBoxFrameColor(widget, inputBox, r, g, b) DESCRIPTION This will change the frame color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, and blue. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long red – red component of color
- long green – green component of color
- long blue – blue component of color RETURN VALUE 1 if successful
Definition at line 230 of file python/input.cpp.
InputBox/changeInputBoxSelectedTextColor.
SYNOPSIS long changeInputBoxSelectedTextColor(widget, inputBox, r, g, b) DESCRIPTION This will change the selected text color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, and blue. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long red – red component of color
- long green – green component of color
- long blue – blue component of color RETURN VALUE 1 if successful
Definition at line 257 of file python/input.cpp.
InputBox/changeInputBoxSelectionColor.
SYNOPSIS long changeInputBoxSelectionColor(widget, inputBox, r, g, b) DESCRIPTION This will change the color of the selection of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, and blue. ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box
- long red – red component of color
- long green – green component of color
- long blue – blue component of color RETURN VALUE 1 if successful
Definition at line 176 of file python/input.cpp.
InputBox/changeInputBox.
SYNOPSIS long changeInputBox(widget, inputBox, value) DESCRIPTION This will change the contents of a input box widget. ARGUMENTS
- long widget – karamba
- long text – pointer to Input Box
- long value – new value RETURN VALUE 1 if successful
Definition at line 87 of file python/input.cpp.
InputBox/setInputFocus.
SYNOPSIS long setInputFocus(widget, inputBox) DESCRIPTION Sets the Input Focus to the Input Box ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE 1 if successful
Definition at line 311 of file python/input.cpp.
InputBox/showInputBox.
SYNOPSIS long showInputBox(widget, inputBox) DESCRIPTION Shows Input Box that has been hidden with hideInputBox() ARGUMENTS
- long widget – karamba
- long inputBox – pointer to Input Box RETURN VALUE 1 if successful
Definition at line 97 of file python/input.cpp.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.