KTextEditor
#include <plugin.h>
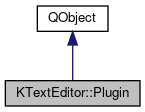
Public Member Functions | |
Plugin (QObject *parent) | |
virtual | ~Plugin () |
virtual void | addDocument (Document *document) |
virtual void | addView (View *view) |
virtual void | removeDocument (Document *document) |
virtual void | removeView (View *view) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KTextEditor Plugin interface.
Topics:
Introduction
The Plugin class provides methods to create loadable plugins for all KTextEditor implementations. A plugin can handle several documents and views. For every document the plugin should handle addDocument() is called and for every view addView().
Configuration Management
- Todo:
write docu about config pages (new with kpluginmanager)
write docu about save/load settings (related to config page)
Session Management
As an extension a Plugin can implement the SessionConfigInterface. This interface provides functions to read and write session related settings. If you have session dependent data additionally derive your Plugin from this interface and implement the session related functions, for example:
Plugin Architecture
After the plugin is loaded the editor implementation should call addDocument() and addView() for all documents and views the plugin should handle. If your plugin has a GUI it is common to add an extra class, like:
Your KTextEditor::Plugin derived class then will create a new PluginView for every View, i.e. for every call of addView().
The method removeView() will be called whenever a View is removed/closed. If you have a PluginView for every view this is the place to remove it.
Constructor & Destructor Documentation
Plugin::Plugin | ( | QObject * | parent | ) |
Constructor.
Create a new plugin.
- Parameters
-
parent parent object
Definition at line 206 of file ktexteditor.cpp.
|
virtual |
Virtual destructor.
Definition at line 211 of file ktexteditor.cpp.
Member Function Documentation
|
inlinevirtual |
Add a new document
to the plugin.
This method is called whenever the plugin should handle another document
.
For every call of addDocument() will finally follow a call of removeDocument(), i.e. the number of calls are identic.
- Parameters
-
document new document to handle
- See also
- removeDocument(), addView()
|
inlinevirtual |
This method is called whenever the plugin should add its GUI to view
.
The process for the Editor can be roughly described as follows:
- add documents the plugin should handle via addDocument()
- for every document doc call addView() for every view for doc.
For every call of addView() will finally follow a call of removeView(), i.e. the number of calls are identic.
- Note
- As addView() is called for every view in which the plugin's GUI should be visible you must not add the GUI by iterating over all Document::views() yourself neither use the signal Document::viewCreated().
- Parameters
-
view view to hang the gui in
- See also
- removeView(), addDocument()
|
inlinevirtual |
Remove the document
from the plugin.
This method is called whenever the plugin should stop handling the document
.
For every call of addDocument() will finally follow a call of removeDocument(), i.e. the number of calls are identic.
- Parameters
-
document document to hang the gui out from
- See also
- addDocument(), removeView()
|
inlinevirtual |
This method is called whenever the plugin should remove its GUI from view
.
For every call of addView() will finally follow a call of removeView(), i.e. the number of calls are identic.
- Parameters
-
view view to hang the gui out from
- See also
- addView(), removeDocument()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:56:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.