Kate
#include <katelayoutcache.h>
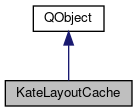
Public Member Functions | |
KateLayoutCache (KateRenderer *renderer, QObject *parent) | |
bool | acceptDirtyLayouts () |
void | clear () |
int | displayViewLine (const KTextEditor::Cursor &virtualCursor, bool limitToVisible=false) |
int | lastViewLine (int realLine) |
KateLineLayoutPtr | line (int realLine, int virtualLine=-1) |
KateLineLayoutPtr | line (const KTextEditor::Cursor &realCursor) |
void | relayoutLines (int startRealLine, int endRealLine) |
void | setAcceptDirtyLayouts (bool accept) |
void | setViewWidth (int width) |
void | setWrap (bool wrap) |
KateTextLayout | textLayout (const KTextEditor::Cursor &realCursor) |
KateTextLayout | textLayout (uint realLine, int viewLine) |
void | updateViewCache (const KTextEditor::Cursor &startPos, int newViewLineCount=-1, int viewLinesScrolled=0) |
void | viewCacheDebugOutput () const |
KTextEditor::Cursor | viewCacheEnd () const |
int | viewCacheLineCount () const |
KTextEditor::Cursor | viewCacheStart () const |
KateTextLayout & | viewLine (int viewLine) |
int | viewLine (const KTextEditor::Cursor &realCursor) |
int | viewLineCount (int realLine) |
int | viewWidth () const |
bool | wrap () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class handles Kate's caching of layouting information (in KateLineLayout and KateTextLayout).
This information is used primarily by both the view and the renderer.
We outsource the hardcore layouting logic to the renderer, but other than that, this class handles all manipulation of the layout objects.
This is separate from the renderer 1) for clarity 2) so you can have separate caches for separate views of the same document, even for view and printer (if the renderer is made to support rendering onto different targets).
Definition at line 77 of file katelayoutcache.h.
Constructor & Destructor Documentation
|
explicit |
connect to all possible editing primitives
Definition at line 140 of file katelayoutcache.cpp.
Member Function Documentation
bool KateLayoutCache::acceptDirtyLayouts | ( | ) |
Definition at line 560 of file katelayoutcache.cpp.
void KateLayoutCache::clear | ( | ) |
Definition at line 517 of file katelayoutcache.cpp.
int KateLayoutCache::displayViewLine | ( | const KTextEditor::Cursor & | virtualCursor, |
bool | limitToVisible = false |
||
) |
Definition at line 405 of file katelayoutcache.cpp.
int KateLayoutCache::lastViewLine | ( | int | realLine | ) |
Definition at line 466 of file katelayoutcache.cpp.
KateLineLayoutPtr KateLayoutCache::line | ( | int | realLine, |
int | virtualLine = -1 |
||
) |
Returns the KateLineLayout for the specified line.
If one does not exist, it will be created and laid out. Layouts which are not directly part of the view will be kept until the cache is full or until they are invalidated by other means (eg. the text changes).
- Parameters
-
realLine real line number of the layout to retrieve. virtualLine virtual line number. only needed if you think it may have changed (ie. basically internal to KateLayoutCache)
Definition at line 280 of file katelayoutcache.cpp.
KateLineLayoutPtr KateLayoutCache::line | ( | const KTextEditor::Cursor & | realCursor | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 332 of file katelayoutcache.cpp.
void KateLayoutCache::relayoutLines | ( | int | startRealLine, |
int | endRealLine | ||
) |
Definition at line 552 of file katelayoutcache.cpp.
void KateLayoutCache::setAcceptDirtyLayouts | ( | bool | accept | ) |
Definition at line 565 of file katelayoutcache.cpp.
void KateLayoutCache::setViewWidth | ( | int | width | ) |
Definition at line 524 of file katelayoutcache.cpp.
void KateLayoutCache::setWrap | ( | bool | wrap | ) |
Definition at line 546 of file katelayoutcache.cpp.
KateTextLayout KateLayoutCache::textLayout | ( | const KTextEditor::Cursor & | realCursor | ) |
Returns the layout describing the text line which is occupied by realCursor
.
Definition at line 337 of file katelayoutcache.cpp.
KateTextLayout KateLayoutCache::textLayout | ( | uint | realLine, |
int | viewLine | ||
) |
Returns the layout of the specified realLine + viewLine.
if viewLine is -1, return the last.
Definition at line 347 of file katelayoutcache.cpp.
void KateLayoutCache::updateViewCache | ( | const KTextEditor::Cursor & | startPos, |
int | newViewLineCount = -1 , |
||
int | viewLinesScrolled = 0 |
||
) |
Definition at line 159 of file katelayoutcache.cpp.
void KateLayoutCache::viewCacheDebugOutput | ( | ) | const |
Definition at line 480 of file katelayoutcache.cpp.
KTextEditor::Cursor KateLayoutCache::viewCacheEnd | ( | ) | const |
Definition at line 374 of file katelayoutcache.cpp.
int KateLayoutCache::viewCacheLineCount | ( | ) | const |
Definition at line 364 of file katelayoutcache.cpp.
KTextEditor::Cursor KateLayoutCache::viewCacheStart | ( | ) | const |
Definition at line 369 of file katelayoutcache.cpp.
KateTextLayout & KateLayoutCache::viewLine | ( | int | viewLine | ) |
Returns the layout of the corresponding line in the view.
Definition at line 358 of file katelayoutcache.cpp.
int KateLayoutCache::viewLine | ( | const KTextEditor::Cursor & | realCursor | ) |
This returns the view line upon which realCursor is situated.
The view line is the number of lines in the view from the first line The supplied cursor should be in real lines.
Definition at line 389 of file katelayoutcache.cpp.
int KateLayoutCache::viewLineCount | ( | int | realLine | ) |
Definition at line 475 of file katelayoutcache.cpp.
int KateLayoutCache::viewWidth | ( | ) | const |
Definition at line 379 of file katelayoutcache.cpp.
bool KateLayoutCache::wrap | ( | ) | const |
Definition at line 541 of file katelayoutcache.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:57:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.