Kate
#include <katescriptmanager.h>
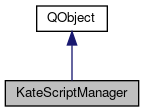
Public Slots | |
void | slotTemplateScriptOwnerDestroyed (QObject *owner) |
Signals | |
void | reloaded () |
Public Member Functions | |
virtual | ~KateScriptManager () |
const QStringList & | cmds () |
void | collect (bool force=false) |
const QVector < KateCommandLineScript * > & | commandLineScripts () |
bool | exec (KTextEditor::View *view, const QString &cmd, QString &errorMsg) |
bool | help (KTextEditor::View *view, const QString &cmd, QString &msg) |
KateIndentScript * | indentationScript (const QString &scriptname) |
KateIndentScript * | indentationScriptByIndex (int index) |
int | indentationScriptCount () |
KateIndentScript * | indenter (const QString &language) |
KTextEditor::TemplateScript * | registerTemplateScript (QObject *owner, const QString &script) |
void | reload () |
KateTemplateScript * | templateScript (KTextEditor::TemplateScript *templateScript) |
void | unregisterTemplateScript (KTextEditor::TemplateScript *templateScript) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | parseMetaInformation (const QString &url, QHash< QString, QString > &pairs) |
static KateScriptManager * | self () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Manage the scripts on disks – find them and query them.
Provides access to loaded scripts too.
Definition at line 43 of file katescriptmanager.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 52 of file katescriptmanager.cpp.
Member Function Documentation
const QStringList & KateScriptManager::cmds | ( | ) |
supported commands as prefixes
- Returns
- prefix list
Definition at line 366 of file katescriptmanager.cpp.
void KateScriptManager::collect | ( | bool | force = false | ) |
Find all of the scripts matching the wildcard directory
.
The resource file with the name resourceFile
is used for caching. If force
is true, then the cache will not be used. This populates the internal lists of scripts. This is automatically called by init so you shouldn't call it yourself unless you want to refresh the collected list.
now, we search all kinds of known scripts
remember type
Definition at line 89 of file katescriptmanager.cpp.
|
inline |
Get all scripts available in the command line.
Definition at line 54 of file katescriptmanager.h.
execute command
Kate::Command stuff.
- Parameters
-
view view to use for execution cmd cmd string errorMsg error to return if no success
- Returns
- success
Definition at line 333 of file katescriptmanager.cpp.
get help for a command
- Parameters
-
view view to use cmd cmd name msg help message
- Returns
- help available or not
Definition at line 353 of file katescriptmanager.cpp.
|
inline |
Definition at line 119 of file katescriptmanager.h.
|
inline |
Definition at line 122 of file katescriptmanager.h.
|
inline |
Definition at line 121 of file katescriptmanager.h.
KateIndentScript * KateScriptManager::indenter | ( | const QString & | language | ) |
Get an indentation script for the given language – if there is more than one result, this will return the script with the highest priority.
If both have the same priority, an arbitrary script will be returned. If no scripts are found, 0 is returned.
Definition at line 60 of file katescriptmanager.cpp.
|
static |
Extract the meta data from the script at url
and put in pairs
.
Returns true if metadata was found and extracted successfuly, false otherwise.
Definition at line 275 of file katescriptmanager.cpp.
KTextEditor::TemplateScript * KateScriptManager::registerTemplateScript | ( | QObject * | owner, |
const QString & | script | ||
) |
managing of scripts for the template handler.
The scripts are given as string content, not as files
Definition at line 378 of file katescriptmanager.cpp.
void KateScriptManager::reload | ( | ) |
explicitly reload all scripts
Definition at line 325 of file katescriptmanager.cpp.
|
signal |
this signal is emitted when all scripts are deleted and reloaded again.
|
inlinestatic |
Definition at line 92 of file katescriptmanager.h.
|
slot |
Definition at line 400 of file katescriptmanager.cpp.
KateTemplateScript * KateScriptManager::templateScript | ( | KTextEditor::TemplateScript * | templateScript | ) |
Definition at line 410 of file katescriptmanager.cpp.
void KateScriptManager::unregisterTemplateScript | ( | KTextEditor::TemplateScript * | templateScript | ) |
unregister a given script
Definition at line 389 of file katescriptmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:57:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.