Kate
#include <kateundomanager.h>
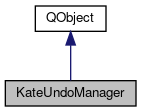
Public Slots | |
void | clearRedo () |
void | clearUndo () |
void | editEnd () |
void | editStart () |
void | endUndo () |
void | inputMethodEnd () |
void | inputMethodStart () |
void | redo () |
void | slotLineInserted (int line, const QString &s) |
void | slotLineRemoved (int line, const QString &s) |
void | slotLineUnWrapped (int line, int col, int length, bool lineRemoved) |
void | slotLineWrapped (int line, int col, int length, bool newLine) |
void | slotMarkLineAutoWrapped (int line, bool autowrapped) |
void | slotTextInserted (int line, int col, const QString &s) |
void | slotTextRemoved (int line, int col, const QString &s) |
void | startUndo () |
void | undo () |
Signals | |
void | isActiveChanged (bool enabled) |
void | redoEnd (KTextEditor::Document *) |
void | redoStart (KTextEditor::Document *) |
void | undoChanged () |
void | undoEnd (KTextEditor::Document *) |
void | undoStart (KTextEditor::Document *) |
Public Member Functions | |
KateUndoManager (KateDocument *doc) | |
~KateUndoManager () | |
KTextEditor::Document * | document () |
bool | isActive () const |
KTextEditor::Cursor | lastRedoCursor () const |
uint | redoCount () const |
void | setAllowComplexMerge (bool allow) |
void | setModified (bool modified) |
void | setUndoRedoCursorsOfLastGroup (const KTextEditor::Cursor undoCursor, const KTextEditor::Cursor redoCursor) |
uint | undoCount () const |
void | undoSafePoint () |
void | updateConfig () |
void | updateLineModifications () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KateUndoManager implements a document's history.
It is in either of the two states:
- the default state, which allows rolling back and forth the history of a document, and
- a state in which a new element is being added to the history.
The state of the KateUndomanager can be switched using editStart() and editEnd().
Definition at line 45 of file kateundomanager.h.
Constructor & Destructor Documentation
KateUndoManager::KateUndoManager | ( | KateDocument * | doc | ) |
Creates a clean undo history.
- Parameters
-
doc the document the KateUndoManager will belong to
Definition at line 27 of file kateundomanager.cpp.
KateUndoManager::~KateUndoManager | ( | ) |
Definition at line 44 of file kateundomanager.cpp.
Member Function Documentation
|
slot |
Definition at line 354 of file kateundomanager.cpp.
|
slot |
Definition at line 343 of file kateundomanager.cpp.
KTextEditor::Document * KateUndoManager::document | ( | ) |
Definition at line 55 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager about the end of an edit.
Definition at line 82 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager about the beginning of an edit.
Definition at line 65 of file kateundomanager.cpp.
|
slot |
Definition at line 133 of file kateundomanager.cpp.
|
slot |
Definition at line 121 of file kateundomanager.cpp.
|
slot |
Definition at line 115 of file kateundomanager.cpp.
|
inline |
Definition at line 90 of file kateundomanager.h.
|
signal |
KTextEditor::Cursor KateUndoManager::lastRedoCursor | ( | ) | const |
Returns the redo cursor of the last undo group.
Needed for the swap file recovery.
Definition at line 414 of file kateundomanager.cpp.
|
slot |
Redo the latest undo group.
Make sure isDefaultState() is true when calling this method.
Definition at line 251 of file kateundomanager.cpp.
uint KateUndoManager::redoCount | ( | ) | const |
Returns how many redo() actions can be performed.
- Returns
- the number of undo groups which can be redone
Definition at line 229 of file kateundomanager.cpp.
|
signal |
|
signal |
void KateUndoManager::setAllowComplexMerge | ( | bool | allow | ) |
Allows or disallows merging of "complex" undo groups.
When an undo group contains different types of undo items, it is considered a "complex" group.
- Parameters
-
allow whether complex merging is allowed
Definition at line 429 of file kateundomanager.cpp.
void KateUndoManager::setModified | ( | bool | modified | ) |
Definition at line 365 of file kateundomanager.cpp.
void KateUndoManager::setUndoRedoCursorsOfLastGroup | ( | const KTextEditor::Cursor | undoCursor, |
const KTextEditor::Cursor | redoCursor | ||
) |
Used by the swap file recovery, this function afterwards manipulates the undo/redo cursors of the last KateUndoGroup.
This function should not be used other than by Kate::SwapFile.
- Parameters
-
undoCursor the undo cursor redoCursor the redo cursor
Definition at line 403 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that a line was inserted.
Definition at line 169 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that a line was removed.
Definition at line 175 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that a line was un-wrapped.
Definition at line 163 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that a line was wrapped.
Definition at line 157 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that a line was marked as autowrapped.
Definition at line 151 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that text was inserted.
Definition at line 139 of file kateundomanager.cpp.
|
slot |
Notify KateUndoManager that text was removed.
Definition at line 145 of file kateundomanager.cpp.
|
slot |
Definition at line 127 of file kateundomanager.cpp.
|
slot |
Undo the latest undo group.
Make sure isDefaultState() is true when calling this method.
Definition at line 234 of file kateundomanager.cpp.
|
signal |
uint KateUndoManager::undoCount | ( | ) | const |
Returns how many undo() actions can be performed.
- Returns
- the number of undo groups which can be undone
Definition at line 224 of file kateundomanager.cpp.
|
signal |
void KateUndoManager::undoSafePoint | ( | ) |
Prevent latest KateUndoGroup from being merged with the next one.
Definition at line 190 of file kateundomanager.cpp.
|
signal |
void KateUndoManager::updateConfig | ( | ) |
Definition at line 424 of file kateundomanager.cpp.
void KateUndoManager::updateLineModifications | ( | ) |
Definition at line 382 of file kateundomanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:57:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.