Kate
#include <kateviinputmodemanager.h>
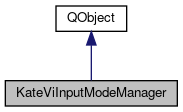
Classes | |
class | Completion |
Public Member Functions | |
KateViInputModeManager (KateView *view, KateViewInternal *viewInternal) | |
~KateViInputModeManager () | |
void | addJump (KTextEditor::Cursor cursor) |
void | addMark (KateDocument *doc, const QChar &mark, const KTextEditor::Cursor &pos, const bool moveoninsert=true, const bool showmark=true) |
void | appendKeyEventToLog (const QKeyEvent &e) |
void | changeViMode (ViMode newMode) |
void | clearCurrentChangeLog () |
void | doNotLogCurrentKeypress () |
void | feedKeyPresses (const QString &keyPresses) const |
void | finishRecordingMacro () |
ViMode | getCurrentViMode () const |
KateViModeBase * | getCurrentViModeHandler () const |
const QString | getLastSearchPattern () const |
KTextEditor::Cursor | getMarkPosition (const QChar &mark) const |
QString | getMarksOnTheLine (int line) |
KTextEditor::Cursor | getNextJump (KTextEditor::Cursor cursor) |
ViMode | getPreviousViMode () const |
KTextEditor::Cursor | getPrevJump (KTextEditor::Cursor cursor) |
bool | getTemporaryNormalMode () |
const QString | getVerbatimKeys () const |
KateViInsertMode * | getViInsertMode () |
KateViNormalMode * | getViNormalMode () |
KateViReplaceMode * | getViReplaceMode () |
KateViVisualMode * | getViVisualMode () |
bool | handleKeypress (const QKeyEvent *e) |
bool | isAnyVisualMode () const |
bool | isHandlingKeypress () const |
bool | isRecordingMacro () |
bool | isReplayingLastChange () const |
bool | isReplayingMacro () |
KateViKeyMapper * | keyMapper () |
bool | lastSearchBackwards () const |
bool | lastSearchCaseSensitive () |
bool | lastSearchPlacesCursorAtEndOfMatch () |
void | logCompletionEvent (const Completion &completion) |
Completion | nextLoggedCompletion () |
void | PrintJumpList () |
void | readSessionConfig (const KConfigGroup &config) |
void | repeatLastChange () |
void | replayMacro (QChar macroRegister) |
void | reset () |
void | setLastSearchBackwards (bool b) |
void | setLastSearchCaseSensitive (bool caseSensitive) |
void | setLastSearchPattern (const QString &p) |
void | setLastSearchPlacesCursorAtEndOfMatch (bool b) |
void | setTemporaryNormalMode (bool b) |
void | startRecordingMacro (QChar macroRegister) |
void | storeLastChangeCommand () |
void | syncViMarksAndBookmarks () |
void | viEnterInsertMode () |
void | viEnterNormalMode () |
void | viEnterReplaceMode () |
void | viEnterVisualMode (ViMode visualMode=VisualMode) |
void | writeSessionConfig (KConfigGroup &config) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static QString | modeToString (ViMode mode) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Definition at line 68 of file kateviinputmodemanager.h.
Constructor & Destructor Documentation
KateViInputModeManager::KateViInputModeManager | ( | KateView * | view, |
KateViewInternal * | viewInternal | ||
) |
Definition at line 50 of file kateviinputmodemanager.cpp.
KateViInputModeManager::~KateViInputModeManager | ( | ) |
Definition at line 104 of file kateviinputmodemanager.cpp.
Member Function Documentation
void KateViInputModeManager::addJump | ( | KTextEditor::Cursor | cursor | ) |
Definition at line 668 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::addMark | ( | KateDocument * | doc, |
const QChar & | mark, | ||
const KTextEditor::Cursor & | pos, | ||
const bool | moveoninsert = true , |
||
const bool | showmark = true |
||
) |
Add a mark to the document.
By default, the mark is made visible in the document by highlighting its line, and it moves when inserting text at it.
- Parameters
-
doc the document to insert the mark into mark the mark's name pos the mark's position moveoninsert whether the mark should move or stay behind when inserting text at it showmark whether to highlight the mark's line
Definition at line 737 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::appendKeyEventToLog | ( | const QKeyEvent & | e | ) |
append a QKeyEvent to the key event log
Definition at line 271 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::changeViMode | ( | ViMode | newMode | ) |
changes the current vi mode to the given mode
Definition at line 445 of file kateviinputmodemanager.cpp.
|
inline |
clear the key event log
Definition at line 173 of file kateviinputmodemanager.h.
void KateViInputModeManager::doNotLogCurrentKeypress | ( | ) |
Definition at line 411 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::feedKeyPresses | ( | const QString & | keyPresses | ) | const |
feed key the given list of key presses to the key handling code, one by one
Definition at line 172 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::finishRecordingMacro | ( | ) |
Definition at line 334 of file kateviinputmodemanager.cpp.
ViMode KateViInputModeManager::getCurrentViMode | ( | ) | const |
- Returns
- The current vi mode
Definition at line 451 of file kateviinputmodemanager.cpp.
KateViModeBase * KateViInputModeManager::getCurrentViModeHandler | ( | ) | const |
- Returns
- one of getViNormalMode(), getViVisualMode(), etc, depending on getCurrentViMode().
Definition at line 466 of file kateviinputmodemanager.cpp.
const QString KateViInputModeManager::getLastSearchPattern | ( | ) | const |
The current search pattern.
This is set by the last search.
- Returns
- the search pattern or the empty string if not set
Definition at line 422 of file kateviinputmodemanager.cpp.
Cursor KateViInputModeManager::getMarkPosition | ( | const QChar & | mark | ) | const |
Definition at line 781 of file kateviinputmodemanager.cpp.
QString KateViInputModeManager::getMarksOnTheLine | ( | int | line | ) |
Definition at line 872 of file kateviinputmodemanager.cpp.
Cursor KateViInputModeManager::getNextJump | ( | KTextEditor::Cursor | cursor | ) |
Definition at line 687 of file kateviinputmodemanager.cpp.
ViMode KateViInputModeManager::getPreviousViMode | ( | ) | const |
- Returns
- the previous vi mode
Definition at line 456 of file kateviinputmodemanager.cpp.
Cursor KateViInputModeManager::getPrevJump | ( | KTextEditor::Cursor | cursor | ) |
Definition at line 704 of file kateviinputmodemanager.cpp.
|
inline |
Definition at line 239 of file kateviinputmodemanager.h.
const QString KateViInputModeManager::getVerbatimKeys | ( | ) | const |
Definition at line 566 of file kateviinputmodemanager.cpp.
KateViInsertMode * KateViInputModeManager::getViInsertMode | ( | ) |
- Returns
- the KateViInsertMode instance
Definition at line 551 of file kateviinputmodemanager.cpp.
KateViNormalMode * KateViInputModeManager::getViNormalMode | ( | ) |
- Returns
- the KateViNormalMode instance
Definition at line 546 of file kateviinputmodemanager.cpp.
KateViReplaceMode * KateViInputModeManager::getViReplaceMode | ( | ) |
- Returns
- the KateViReplaceMode instance
Definition at line 561 of file kateviinputmodemanager.cpp.
KateViVisualMode * KateViInputModeManager::getViVisualMode | ( | ) |
- Returns
- the KateViVisualMode instance
Definition at line 556 of file kateviinputmodemanager.cpp.
bool KateViInputModeManager::handleKeypress | ( | const QKeyEvent * | e | ) |
feed key the given key press to the command parser
- Returns
- true if keypress was is [part of a] command, false otherwise
Definition at line 113 of file kateviinputmodemanager.cpp.
bool KateViInputModeManager::isAnyVisualMode | ( | ) | const |
- Returns
- true if and only if the current mode is one of VisualMode, VisualBlockMode or VisualLineMode.
Definition at line 461 of file kateviinputmodemanager.cpp.
bool KateViInputModeManager::isHandlingKeypress | ( | ) | const |
Determines whether we are currently processing a Vi keypress.
- Returns
- true if we are still in a call to handleKeypress, false otherwise
Definition at line 266 of file kateviinputmodemanager.cpp.
bool KateViInputModeManager::isRecordingMacro | ( | ) |
Definition at line 341 of file kateviinputmodemanager.cpp.
|
inline |
- Returns
- true if running replaying the last change due to pressing "."
Definition at line 163 of file kateviinputmodemanager.h.
bool KateViInputModeManager::isReplayingMacro | ( | ) |
Definition at line 369 of file kateviinputmodemanager.cpp.
KateViKeyMapper * KateViInputModeManager::keyMapper | ( | ) |
Definition at line 913 of file kateviinputmodemanager.cpp.
|
inline |
get search direction of last search.
(true if backwards, false if forwards)
Definition at line 224 of file kateviinputmodemanager.h.
|
inline |
Definition at line 235 of file kateviinputmodemanager.h.
|
inline |
Definition at line 237 of file kateviinputmodemanager.h.
void KateViInputModeManager::logCompletionEvent | ( | const Completion & | completion | ) |
Definition at line 374 of file kateviinputmodemanager.cpp.
convert mode to string representation for user
- Parameters
-
mode mode enum value
- Returns
- user visible string
Definition at line 886 of file kateviinputmodemanager.cpp.
KateViInputModeManager::Completion KateViInputModeManager::nextLoggedCompletion | ( | ) |
Definition at line 388 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::PrintJumpList | ( | ) |
Definition at line 722 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::readSessionConfig | ( | const KConfigGroup & | config | ) |
Definition at line 588 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::repeatLastChange | ( | ) |
repeat last change by feeding the contents of m_lastChange to feedKeys()
Definition at line 315 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::replayMacro | ( | QChar | macroRegister | ) |
Definition at line 346 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::reset | ( | ) |
Definition at line 663 of file kateviinputmodemanager.cpp.
|
inline |
set search direction of last search.
(true if backwards, false if forwards)
Definition at line 229 of file kateviinputmodemanager.h.
|
inline |
Definition at line 231 of file kateviinputmodemanager.h.
void KateViInputModeManager::setLastSearchPattern | ( | const QString & | p | ) |
Set the current search pattern.
This is used by the "n" and "N" motions.
- Parameters
-
p the search pattern
Definition at line 434 of file kateviinputmodemanager.cpp.
|
inline |
Definition at line 233 of file kateviinputmodemanager.h.
|
inline |
Definition at line 241 of file kateviinputmodemanager.h.
void KateViInputModeManager::startRecordingMacro | ( | QChar | macroRegister | ) |
Definition at line 323 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::storeLastChangeCommand | ( | ) |
copy the contents of the key events log to m_lastChange so that it can be repeated
Definition at line 279 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::syncViMarksAndBookmarks | ( | ) |
Definition at line 820 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::viEnterInsertMode | ( | ) |
set insert mode to be the active vi mode and perform the needed setup work
Definition at line 511 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::viEnterNormalMode | ( | ) |
set normal mode to be the active vi mode and perform the needed setup work
Definition at line 484 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::viEnterReplaceMode | ( | ) |
set replace mode to be the active vi mode and make the needed setup work
Definition at line 538 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::viEnterVisualMode | ( | ViMode | visualMode = VisualMode | ) |
set visual mode to be the active vi mode and make the needed setup work
Definition at line 526 of file kateviinputmodemanager.cpp.
void KateViInputModeManager::writeSessionConfig | ( | KConfigGroup & | config | ) |
Definition at line 624 of file kateviinputmodemanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:57:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.