Konsole
#include <Filter.h>
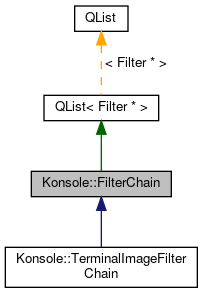
Public Member Functions | |
virtual | ~FilterChain () |
void | addFilter (Filter *filter) |
void | clear () |
Filter::HotSpot * | hotSpotAt (int line, int column) const |
QList< Filter::HotSpot * > | hotSpots () const |
QList< Filter::HotSpot > | hotSpotsAtLine (int line) const |
void | process () |
void | removeFilter (Filter *filter) |
void | reset () |
void | setBuffer (const QString *buffer, const QList< int > *linePositions) |
Additional Inherited Members | |
![]() | |
QList () | |
QList (const QList< T > &other) | |
QList (std::initializer_list< T > args) | |
~QList () | |
void | append (const T &value) |
void | append (const QList< T > &value) |
const T & | at (int i) const |
T & | back () |
const T & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const T &value) const |
int | count (const T &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const T &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const T &t) |
iterator | find (const T &t) |
const_iterator | find (const T &t) const |
const_iterator | find (const_iterator from, const T &t) const |
int | findIndex (const T &t) const |
T & | first () |
const T & | first () const |
T & | front () |
const T & | front () const |
int | indexOf (const T &value, int from) const |
void | insert (int i, const T &value) |
iterator | insert (iterator before, const T &value) |
bool | isEmpty () const |
T & | last () |
const T & | last () const |
int | lastIndexOf (const T &value, int from) const |
int | length () const |
QList< T > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< T > &other) const |
QList< T > | operator+ (const QList< T > &other) const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const T &value) |
QList< T > & | operator<< (const T &value) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
bool | operator== (const QList< T > &other) const |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const T &value) |
void | push_back (const T &value) |
void | push_front (const T &value) |
iterator | remove (iterator pos) |
int | remove (const T &t) |
int | removeAll (const T &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const T &value) |
void | replace (int i, const T &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const T &value) const |
void | swap (int i, int j) |
void | swap (QList< T > &other) |
T | takeAt (int i) |
T | takeFirst () |
T | takeLast () |
QSet< T > | toSet () const |
std::list< T > | toStdList () const |
QVector< T > | toVector () const |
T | value (int i, const T &defaultValue) const |
T | value (int i) const |
![]() | |
QList< T > | fromSet (const QSet< T > &set) |
QList< T > | fromStdList (const std::list< T > &list) |
QList< T > | fromVector (const QVector< T > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
A chain which allows a group of filters to be processed as one.
The chain owns the filters added to it and deletes them when the chain itself is destroyed.
Use addFilter() to add a new filter to the chain. When new text to be filtered arrives, use addLine() to add each additional line of text which needs to be processed and then after adding the last line, use process() to cause each filter in the chain to process the text.
After processing a block of text, the reset() method can be used to set the filter chain's internal cursor back to the first line.
The hotSpotAt() method will return the first hotspot which covers a given position.
The hotSpots() and hotSpotsAtLine() method return all of the hotspots in the text and on a given line respectively.
Constructor & Destructor Documentation
|
virtual |
Definition at line 40 of file Filter.cpp.
Member Function Documentation
void FilterChain::addFilter | ( | Filter * | filter | ) |
Adds a new filter to the chain.
The chain will delete this filter when it is destroyed
Definition at line 51 of file Filter.cpp.
void FilterChain::clear | ( | ) |
Removes all filters from the chain.
Definition at line 77 of file Filter.cpp.
Filter::HotSpot * FilterChain::hotSpotAt | ( | int | line, |
int | column | ||
) | const |
Returns the first hotspot which occurs at line
, column
or 0 if no hotspot was found.
Definition at line 81 of file Filter.cpp.
QList< Filter::HotSpot * > FilterChain::hotSpots | ( | ) | const |
Returns a list of all the hotspots in all the chain's filters.
Definition at line 95 of file Filter.cpp.
QList<Filter::HotSpot> Konsole::FilterChain::hotSpotsAtLine | ( | int | line | ) | const |
Returns a list of all hotspots at the given line in all the chain's filters.
void FilterChain::process | ( | ) |
Processes each filter in the chain.
Definition at line 71 of file Filter.cpp.
void FilterChain::removeFilter | ( | Filter * | filter | ) |
Removes a filter from the chain.
The chain will no longer delete the filter when destroyed
Definition at line 55 of file Filter.cpp.
void FilterChain::reset | ( | ) |
Resets each filter in the chain.
Definition at line 59 of file Filter.cpp.
Sets the buffer for each filter in the chain to process.
Definition at line 65 of file Filter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Sat May 9 2020 03:56:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.