Analitza
#include <operator.h>
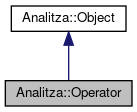
Public Types | |
enum | OperatorType { none =0, plus, times, minus, divide, quotient, power, root, factorial, _and, _or, _xor, _not, gcd, lcm, rem, factorof, max, min, lt, gt, eq, neq, leq, geq, implies, approx, abs, floor, ceiling, sin, cos, tan, sec, csc, cot, sinh, cosh, tanh, sech, csch, coth, arcsin, arccos, arctan, arccot, arccosh, arccsc, arccsch, arcsec, arcsech, arcsinh, arctanh, exp, ln, log, sum, product, diff, card, scalarproduct, selector, _union, forall, exists, map, filter, transpose, function, nOfOps } |
![]() | |
enum | ObjectType { none =0, value, variable, vector, list, apply, oper, container, matrix, matrixrow, custom } |
Public Member Functions | |
Operator (OperatorType t) | |
virtual | ~Operator () |
virtual QVariant | accept (AbstractExpressionVisitor *) const |
virtual Operator * | copy () const |
virtual bool | decorate (const QMap< QString, Object ** > &) |
Operator | inverse () const |
bool | isBounded () const |
bool | isCorrect () const |
bool | isTrigonometric () const |
virtual bool | matches (const Object *, QMap< QString, const Object * > *) const |
OperatorType | multiplicityOperator () const |
QString | name () const |
int | nparams () const |
bool | operator!= (OperatorType o) const |
bool | operator!= (const Operator &o) const |
Operator | operator= (const Operator &a) |
bool | operator== (const Operator &o) const |
bool | operator== (OperatorType o) const |
OperatorType | operatorType () const |
void | setOperator (OperatorType t) |
![]() | |
virtual | ~Object () |
bool | isApply () const |
bool | isContainer () const |
virtual bool | isZero () const |
QString | toString () const |
enum ObjectType | type () const |
Static Public Member Functions | |
static OperatorType | multiplicityOperator (const OperatorType &t) |
static OperatorType | toOperatorType (const QString &s) |
Static Public Attributes | |
static const char | words [nOfOps][14] |
Additional Inherited Members | |
![]() | |
Object (enum ObjectType t) | |
![]() | |
const ObjectType | m_type |
Detailed Description
Is the operator representation in the trees.
Definition at line 36 of file operator.h.
Member Enumeration Documentation
Specifies the type of an operator.
Definition at line 40 of file operator.h.
Constructor & Destructor Documentation
|
inlineexplicit |
|
inlinevirtual |
Destructor.
Definition at line 73 of file operator.h.
Member Function Documentation
|
virtual |
Returns some string depending on the visior.
Implements Analitza::Object.
|
virtual |
- Returns
- a new and equal instance of the tree.
Implements Analitza::Object.
Definition at line 123 of file operator.h.
Operator Analitza::Operator::inverse | ( | ) | const |
bool Analitza::Operator::isBounded | ( | ) | const |
Returns whether this operator needs bounding.
|
inline |
Returns whether it is a correct object.
Definition at line 112 of file operator.h.
|
inline |
Returns if it is a trigonometric operator.
Definition at line 106 of file operator.h.
|
virtual |
exp
is the tree that we will compare to, found
is where we will pass the variables store the results.
It will return whether the object follows the pattern
structure.
Implements Analitza::Object.
|
inline |
Returns the multiplicity operator.
e.g. 5+5+5+5=5*4 -> times is the multiplicityOperator of plus.
Definition at line 103 of file operator.h.
|
static |
Returns the multiplicity operator of an operator t
.
e.g. 5+5+5+5=5*4 -> times is the multiplicityOperator of plus.
QString Analitza::Operator::name | ( | ) | const |
Returns the string expression representation of the operator.
|
inline |
Returns the number of params expected for the operator type.
Definition at line 79 of file operator.h.
|
inline |
Returns whether o
is different to this operator.
Definition at line 94 of file operator.h.
|
inline |
Returns whether o
is different to this operator.
Definition at line 97 of file operator.h.
Makes this operator equal to a
.
Definition at line 100 of file operator.h.
|
inline |
Returns whether o
is equal to this operator.
Definition at line 88 of file operator.h.
|
inline |
Returns whether o
is equal to this operator.
Definition at line 91 of file operator.h.
|
inline |
Returns the operator type.
Definition at line 82 of file operator.h.
|
inline |
Sets an operator type to the object.
Definition at line 76 of file operator.h.
|
static |
Converts a s
operator tag to the operator.
Member Data Documentation
|
static |
Definition at line 128 of file operator.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:11:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.