kig
#include <equation.h>
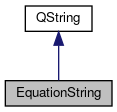
Public Member Functions | |
EquationString (const QString &string) | |
void | addTerm (double coeff, const QString &unknowns, bool &needsign) |
void | prettify (void) |
double | trunc (double) |
const QString | x () const |
const QString | x2 () const |
const QString | x2y () const |
const QString | x3 () const |
const QString | xnym (int n, int m) const |
const QString | xy () const |
const QString | xy2 () const |
const QString | y () const |
const QString | y2 () const |
const QString | y3 () const |
![]() | |
QString () | |
QString (const QChar *unicode, int size) | |
QString (QChar ch) | |
QString (int size, QChar ch) | |
QString (const char *str) | |
QString (const QByteArray &ba) | |
QString (const QChar *unicode) | |
QString (const QLatin1String &str) | |
QString (const QString &other) | |
~QString () | |
QString & | append (const char *str) |
QString & | append (const QByteArray &ba) |
QString & | append (QChar ch) |
QString & | append (const QString &str) |
QString & | append (const QStringRef &reference) |
QString & | append (const QLatin1String &str) |
QString | arg (qlonglong a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (qulonglong a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (long a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (ulong a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (int a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (uint a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (short a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (ushort a, int fieldWidth, int base, const QChar &fillChar) const |
QString | arg (double a, int fieldWidth, char format, int precision, const QChar &fillChar) const |
QString | arg (char a, int fieldWidth, const QChar &fillChar) const |
QString | arg (const QString &a, int fieldWidth, const QChar &fillChar) const |
QString | arg (QChar a, int fieldWidth, const QChar &fillChar) const |
QString | arg (const QString &a1, const QString &a2) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5, const QString &a6) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5, const QString &a6, const QString &a7) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5, const QString &a6, const QString &a7, const QString &a8, const QString &a9) const |
QString | arg (const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5, const QString &a6, const QString &a7, const QString &a8) const |
const char * | ascii () const |
const QChar | at (int position) const |
iterator | begin () |
const_iterator | begin () const |
int | capacity () const |
void | chop (int n) |
void | clear () |
int | compare (const QString &other) const |
int | compare (const QString &other, Qt::CaseSensitivity cs) const |
int | compare (const QLatin1String &other, Qt::CaseSensitivity cs) const |
int | compare (const QStringRef &ref, Qt::CaseSensitivity cs) const |
const_iterator | constBegin () const |
const QChar * | constData () const |
const_iterator | constEnd () const |
QChar | constref (uint i) const |
bool | contains (QChar ch, Qt::CaseSensitivity cs) const |
bool | contains (const QString &str, Qt::CaseSensitivity cs) const |
bool | contains (const QStringRef &str, Qt::CaseSensitivity cs) const |
bool | contains (const QRegExp &rx) const |
bool | contains (QRegExp &rx) const |
bool | contains (QChar c, bool cs) const |
bool | contains (const QString &s, bool cs) const |
QString | copy () const |
int | count (QChar ch, Qt::CaseSensitivity cs) const |
int | count (const QString &str, Qt::CaseSensitivity cs) const |
int | count (const QStringRef &str, Qt::CaseSensitivity cs) const |
int | count (const QRegExp &rx) const |
int | count () const |
QChar * | data () |
const QChar * | data () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const QString &s, Qt::CaseSensitivity cs) const |
bool | endsWith (const QStringRef &s, Qt::CaseSensitivity cs) const |
bool | endsWith (const QChar &c, Qt::CaseSensitivity cs) const |
bool | endsWith (const QLatin1String &s, Qt::CaseSensitivity cs) const |
bool | endsWith (const QString &s, bool cs) const |
QString & | fill (QChar ch, int size) |
int | find (QChar c, int i, bool cs) const |
int | find (const QString &s, int i, bool cs) const |
int | find (const QRegExp &rx, int i) const |
int | findRev (const QString &s, int i, bool cs) const |
int | findRev (QChar c, int i, bool cs) const |
int | findRev (const QRegExp &rx, int i) const |
int | indexOf (const QRegExp &rx, int from) const |
int | indexOf (QRegExp &rx, int from) const |
int | indexOf (const QStringRef &str, int from, Qt::CaseSensitivity cs) const |
int | indexOf (QChar ch, int from, Qt::CaseSensitivity cs) const |
int | indexOf (const QString &str, int from, Qt::CaseSensitivity cs) const |
int | indexOf (const QLatin1String &str, int from, Qt::CaseSensitivity cs) const |
QString & | insert (int position, QChar ch) |
QString & | insert (int position, const QChar *unicode, int size) |
QString & | insert (int position, const QString &str) |
QString & | insert (int position, const QLatin1String &str) |
bool | isEmpty () const |
bool | isNull () const |
bool | isRightToLeft () const |
int | lastIndexOf (const QRegExp &rx, int from) const |
int | lastIndexOf (QRegExp &rx, int from) const |
int | lastIndexOf (const QString &str, int from, Qt::CaseSensitivity cs) const |
int | lastIndexOf (QChar ch, int from, Qt::CaseSensitivity cs) const |
int | lastIndexOf (const QLatin1String &str, int from, Qt::CaseSensitivity cs) const |
int | lastIndexOf (const QStringRef &str, int from, Qt::CaseSensitivity cs) const |
const char * | latin1 () const |
QString | left (int n) const |
QString | leftJustified (int width, QChar fill, bool truncate) const |
QString | leftJustify (int width, QChar fill, bool trunc) const |
QStringRef | leftRef (int n) const |
int | length () const |
QByteArray | local8Bit () const |
int | localeAwareCompare (const QString &other) const |
int | localeAwareCompare (const QStringRef &other) const |
QString | lower () const |
QString | mid (int position, int n) const |
QStringRef | midRef (int position, int n) const |
QString | normalized (NormalizationForm mode) const |
QString | normalized (NormalizationForm mode, QChar::UnicodeVersion version) const |
operator const char * () const | |
bool | operator!= (const QString &other) const |
bool | operator!= (const QLatin1String &other) const |
bool | operator!= (const char *other) const |
bool | operator!= (const QByteArray &other) const |
QString & | operator+= (char ch) |
QString & | operator+= (const char *str) |
QString & | operator+= (const QByteArray &ba) |
QString & | operator+= (const QStringRef &str) |
QString & | operator+= (QChar ch) |
QString & | operator+= (const QString &other) |
QString & | operator+= (const QLatin1String &str) |
bool | operator< (const QString &other) const |
bool | operator< (const QLatin1String &other) const |
bool | operator< (const char *other) const |
bool | operator< (const QByteArray &other) const |
bool | operator<= (const QString &other) const |
bool | operator<= (const QLatin1String &other) const |
bool | operator<= (const char *other) const |
bool | operator<= (const QByteArray &other) const |
QString & | operator= (QChar ch) |
QString & | operator= (const QString &other) |
QString & | operator= (const char *str) |
QString & | operator= (const QByteArray &ba) |
QString & | operator= (char ch) |
QString & | operator= (const QLatin1String &str) |
bool | operator== (const QByteArray &other) const |
bool | operator== (const char *other) const |
bool | operator== (const QString &other) const |
bool | operator== (const QLatin1String &other) const |
bool | operator> (const QByteArray &other) const |
bool | operator> (const QString &other) const |
bool | operator> (const QLatin1String &other) const |
bool | operator> (const char *other) const |
bool | operator>= (const QString &other) const |
bool | operator>= (const QLatin1String &other) const |
bool | operator>= (const char *other) const |
bool | operator>= (const QByteArray &other) const |
const QChar | operator[] (uint position) const |
QCharRef | operator[] (uint position) |
const QChar | operator[] (int position) const |
QCharRef | operator[] (int position) |
QString & | prepend (const char *str) |
QString & | prepend (const QByteArray &ba) |
QString & | prepend (QChar ch) |
QString & | prepend (const QLatin1String &str) |
QString & | prepend (const QString &str) |
void | push_back (const QString &other) |
void | push_back (QChar ch) |
void | push_front (const QString &other) |
void | push_front (QChar ch) |
QChar & | ref (uint i) |
QString & | remove (const QRegExp &rx) |
QString & | remove (const QString &str, Qt::CaseSensitivity cs) |
QString & | remove (const QString &s, bool cs) |
QString & | remove (int position, int n) |
QString & | remove (QChar ch, Qt::CaseSensitivity cs) |
QString & | remove (QChar c, bool cs) |
QString | repeated (int times) const |
QString & | replace (QChar ch, const QString &after, Qt::CaseSensitivity cs) |
QString & | replace (const QRegExp &rx, const QString &after) |
QString & | replace (QChar before, QChar after, Qt::CaseSensitivity cs) |
QString & | replace (QChar c, const QLatin1String &after, Qt::CaseSensitivity cs) |
QString & | replace (const QString &before, const QString &after, bool cs) |
QString & | replace (const QLatin1String &before, const QLatin1String &after, Qt::CaseSensitivity cs) |
QString & | replace (char c, const QString &after, Qt::CaseSensitivity cs) |
QString & | replace (int position, int n, const QChar *unicode, int size) |
QString & | replace (int position, int n, const QString &after) |
QString & | replace (QChar c, const QString &after, bool cs) |
QString & | replace (char c, const QString &after, bool cs) |
QString & | replace (const QChar *before, int blen, const QChar *after, int alen, Qt::CaseSensitivity cs) |
QString & | replace (const QString &before, const QString &after, Qt::CaseSensitivity cs) |
QString & | replace (int position, int n, QChar after) |
QString & | replace (const QLatin1String &before, const QString &after, Qt::CaseSensitivity cs) |
QString & | replace (const QString &before, const QLatin1String &after, Qt::CaseSensitivity cs) |
void | reserve (int size) |
void | resize (int size) |
QString | right (int n) const |
QString | rightJustified (int width, QChar fill, bool truncate) const |
QString | rightJustify (int width, QChar fill, bool trunc) const |
QStringRef | rightRef (int n) const |
QString | section (QChar sep, int start, int end, QFlags< QString::SectionFlag > flags) const |
QString | section (const QString &sep, int start, int end, QFlags< QString::SectionFlag > flags) const |
QString | section (const QRegExp ®, int start, int end, QFlags< QString::SectionFlag > flags) const |
QString & | setAscii (const char *str, int len) |
QString & | setLatin1 (const char *str, int len) |
void | setLength (int nl) |
QString & | setNum (uint n, int base) |
QString & | setNum (int n, int base) |
QString & | setNum (short n, int base) |
QString & | setNum (float n, char format, int precision) |
QString & | setNum (ushort n, int base) |
QString & | setNum (long n, int base) |
QString & | setNum (ulong n, int base) |
QString & | setNum (qlonglong n, int base) |
QString & | setNum (double n, char format, int precision) |
QString & | setNum (qulonglong n, int base) |
QString & | setRawData (const QChar *unicode, int size) |
QString & | setUnicode (const QChar *unicode, int size) |
QString & | setUnicodeCodes (const ushort *unicode_as_ushorts, int size) |
QString & | setUtf16 (const ushort *unicode, int size) |
QString | simplified () const |
QString | simplifyWhiteSpace () const |
int | size () const |
QStringList | split (const QChar &sep, SplitBehavior behavior, Qt::CaseSensitivity cs) const |
QStringList | split (const QRegExp &rx, SplitBehavior behavior) const |
QStringList | split (const QString &sep, SplitBehavior behavior, Qt::CaseSensitivity cs) const |
QString & | sprintf (const char *cformat,...) |
void | squeeze () |
bool | startsWith (const QString &s, Qt::CaseSensitivity cs) const |
bool | startsWith (const QString &s, bool cs) const |
bool | startsWith (const QStringRef &s, Qt::CaseSensitivity cs) const |
bool | startsWith (const QLatin1String &s, Qt::CaseSensitivity cs) const |
bool | startsWith (const QChar &c, Qt::CaseSensitivity cs) const |
QString | stripWhiteSpace () const |
void | swap (QString &other) |
QByteArray | toAscii () const |
QString | toCaseFolded () const |
double | toDouble (bool *ok) const |
float | toFloat (bool *ok) const |
int | toInt (bool *ok, int base) const |
QByteArray | toLatin1 () const |
QByteArray | toLocal8Bit () const |
long | toLong (bool *ok, int base) const |
qlonglong | toLongLong (bool *ok, int base) const |
QString | toLower () const |
short | toShort (bool *ok, int base) const |
std::string | toStdString () const |
std::wstring | toStdWString () const |
QVector< uint > | toUcs4 () const |
uint | toUInt (bool *ok, int base) const |
ulong | toULong (bool *ok, int base) const |
qulonglong | toULongLong (bool *ok, int base) const |
QString | toUpper () const |
ushort | toUShort (bool *ok, int base) const |
QByteArray | toUtf8 () const |
int | toWCharArray (wchar_t *array) const |
QString | trimmed () const |
void | truncate (int position) |
const ushort * | ucs2 () const |
const QChar * | unicode () const |
QString | upper () const |
const ushort * | utf16 () const |
QByteArray | utf8 () const |
QString & | vsprintf (const char *cformat, va_list ap) |
Additional Inherited Members | |
![]() | |
int | compare (const QString &s1, const QString &s2) |
int | compare (const QString &s1, const QString &s2, Qt::CaseSensitivity cs) |
int | compare (const QString &s1, const QLatin1String &s2, Qt::CaseSensitivity cs) |
int | compare (const QLatin1String &s1, const QString &s2, Qt::CaseSensitivity cs) |
int | compare (const QString &s1, const QStringRef &s2, Qt::CaseSensitivity cs) |
QString | fromAscii (const char *str, int size) |
QString | fromLatin1 (const char *str, int size) |
QString | fromLocal8Bit (const char *str, int size) |
QString | fromRawData (const QChar *unicode, int size) |
QString | fromStdString (const std::string &str) |
QString | fromStdWString (const std::wstring &str) |
QString | fromUcs2 (const ushort *unicode, int size) |
QString | fromUcs4 (const uint *unicode, int size) |
QString | fromUtf16 (const ushort *unicode, int size) |
QString | fromUtf8 (const char *str, int size) |
QString | fromWCharArray (const wchar_t *string, int size) |
int | localeAwareCompare (const QString &s1, const QStringRef &s2) |
int | localeAwareCompare (const QString &s1, const QString &s2) |
QString | number (qlonglong n, int base) |
QString | number (double n, char format, int precision) |
QString | number (int n, int base) |
QString | number (ulong n, int base) |
QString | number (long n, int base) |
QString | number (qulonglong n, int base) |
QString | number (uint n, int base) |
![]() | |
typedef | const_reference |
typedef | ConstIterator |
typedef | Iterator |
typedef | reference |
typedef | SectionFlags |
typedef | value_type |
Detailed Description
Simple class that represents an equation.
It does not do any calculation, it serves only as a helper to write equations like in a pretty form. It can handle equations of max two variables (named x and y ) with no limits on the grade of the terms. (well, the limit is 15, but I doubt anyone will ever use such a factor for a power...)
Definition at line 49 of file equation.h.
Constructor & Destructor Documentation
EquationString::EquationString | ( | const QString & | string | ) |
Definition at line 30 of file equation.cc.
Member Function Documentation
void EquationString::addTerm | ( | double | coeff, |
const QString & | unknowns, | ||
bool & | needsign | ||
) |
Definition at line 41 of file equation.cc.
void EquationString::prettify | ( | void | ) |
Definition at line 117 of file equation.cc.
double EquationString::trunc | ( | double | d | ) |
Definition at line 35 of file equation.cc.
const QString EquationString::x | ( | ) | const |
Definition at line 105 of file equation.cc.
const QString EquationString::x2 | ( | ) | const |
Definition at line 90 of file equation.cc.
const QString EquationString::x2y | ( | ) | const |
Definition at line 80 of file equation.cc.
const QString EquationString::x3 | ( | ) | const |
Definition at line 70 of file equation.cc.
const QString EquationString::xnym | ( | int | n, |
int | m | ||
) | const |
Definition at line 123 of file equation.cc.
const QString EquationString::xy | ( | ) | const |
Definition at line 100 of file equation.cc.
const QString EquationString::xy2 | ( | ) | const |
Definition at line 85 of file equation.cc.
const QString EquationString::y | ( | ) | const |
Definition at line 110 of file equation.cc.
const QString EquationString::y2 | ( | ) | const |
Definition at line 95 of file equation.cc.
const QString EquationString::y3 | ( | ) | const |
Definition at line 75 of file equation.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.