kig
#include "conic-common.h"
#include <math.h>
#include "common.h"
#include "kigtransform.h"
#include <cmath>
#include <algorithm>
#include <config-kig.h>
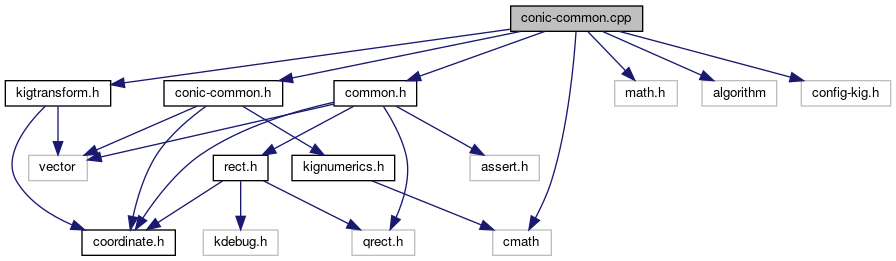
Go to the source code of this file.
Function Documentation
const LineData calcConicAsymptote | ( | const ConicCartesianData | data, |
int | which, | ||
bool & | valid | ||
) |
This function calculates the asymptote of the given conic ( data ).
A conic has two asymptotes in general, so which should be set to +1 or -1 depending on which asymptote you want. As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned line is valid, and to false if the returned line is not valid. The latter condition only occurs if the given conic does not have the specified asymptote.
Definition at line 467 of file conic-common.cpp.
const ConicPolarData calcConicBDFP | ( | const LineData & | d, |
const Coordinate & | f, | ||
const Coordinate & | p | ||
) |
function used by ConicBDFP.
It calcs the conic with directrix d, focus f, and point p on the conic..
Definition at line 433 of file conic-common.cpp.
const ConicPolarData calcConicBFFP | ( | const std::vector< Coordinate > & | args, |
int | type | ||
) |
This function is used by ConicBFFP.
It calcs the polar equation for a hyperbola ( type == -1 ) or ellipse ( type == 1 ) with focuses args[0] and args[1], and with args[2] on the edge of the conic. args.size() should be two or three. If it is two, the two points are taken to be the focuses, and a third point is chosen in a sensible way..
Definition at line 251 of file conic-common.cpp.
const ConicCartesianData calcConicByAsymptotes | ( | const LineData & | line1, |
const LineData & | line2, | ||
const Coordinate & | p | ||
) |
This calcs the hyperbola defined by its two asymptotes line1 and line2, and a point p on the edge.
Definition at line 511 of file conic-common.cpp.
const Coordinate calcConicLineIntersect | ( | const ConicCartesianData & | c, |
const LineData & | l, | ||
double | knownparam, | ||
int | which | ||
) |
This function calculates the intersection of a given line ( l ) and a given conic ( c ).
A line and a conic have two intersections in general, and as such, which should be set to -1 or 1 depending on which intersection you want. As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned point is valid, and to false if the returned point is not valid. The latter condition only occurs if the given conic and line do not have the specified intersection.
knownparam is something special: If you already know one intersection of the line and the conic, and you want the other one, then you should set which to 0, knownparam to the curve parameter of the point you already know ( i.e. the value returned by conicimp->getParam( otherpoint ) ).
Definition at line 367 of file conic-common.cpp.
const LineData calcConicPolarLine | ( | const ConicCartesianData & | data, |
const Coordinate & | cpole, | ||
bool & | valid | ||
) |
This function calculates the polar line of the point cpole with respect to the given conic data.
As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned LineData is valid, and to false if the returned line is not valid. The latter condition only occurs if a "line at infinity" would have had to be returned.
Definition at line 293 of file conic-common.cpp.
const Coordinate calcConicPolarPoint | ( | const ConicCartesianData & | data, |
const LineData & | polar | ||
) |
This function calculates the polar point of the line polar with respect to the given conic data.
As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned LineData is valid, and to false if the returned line is not valid. The latter condition only occurs if a "point at infinity" would have had to be returned.
Definition at line 325 of file conic-common.cpp.
const LineData calcConicRadical | ( | const ConicCartesianData & | cequation1, |
const ConicCartesianData & | cequation2, | ||
int | which, | ||
int | zeroindex, | ||
bool & | valid | ||
) |
This function calculates the radical line of two conics.
A radical line is the line that goes through two of the intersections of two conics. Since two conics have up to four intersections in general, there are three sets of two radical lines. zeroindex specifies which set of radical lines you want ( set it to 1, 2 or 3 ), and which is set to -1 or +1 depending on which of the two radical lines in the set you want. As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned line is valid, and to false if the returned line is not valid. The latter condition only occurs if the given conics do not have the specified radical line.
Definition at line 544 of file conic-common.cpp.
const ConicCartesianData calcConicThroughPoints | ( | const std::vector< Coordinate > & | points, |
const LinearConstraints | c1 = noconstraint , |
||
const LinearConstraints | c2 = noconstraint , |
||
const LinearConstraints | c3 = noconstraint , |
||
const LinearConstraints | c4 = noconstraint , |
||
const LinearConstraints | c5 = noconstraint |
||
) |
Calculate a conic through a given set of points.
points should contain at least one, and at most five points. If there are five points, then the conic is completely defined. If there are less, then additional points will be calculated according to the constraints given. See above for the various constraints.
An invalid ConicCartesianData is returned if there is no conic through the given set of points, or if not enough constraints are given for a conic to be calculated.
Definition at line 169 of file conic-common.cpp.
const ConicCartesianData calcConicTransformation | ( | const ConicCartesianData & | data, |
const Transformation & | t, | ||
bool & | valid | ||
) |
This calculates the image of the given conic ( data ) through the given transformation ( t ).
As the last argument, you should pass a reference to a boolean. This boolean will be set to true if the returned line is valid, and to false if the returned line is not valid. The latter condition only occurs if the given transformation is singular, and as such, the transformation of the conic cannot be calculated.
Definition at line 826 of file conic-common.cpp.
bool operator== | ( | const ConicPolarData & | lhs, |
const ConicPolarData & | rhs | ||
) |
Definition at line 875 of file conic-common.cpp.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.